Sottrai Datetime in Python
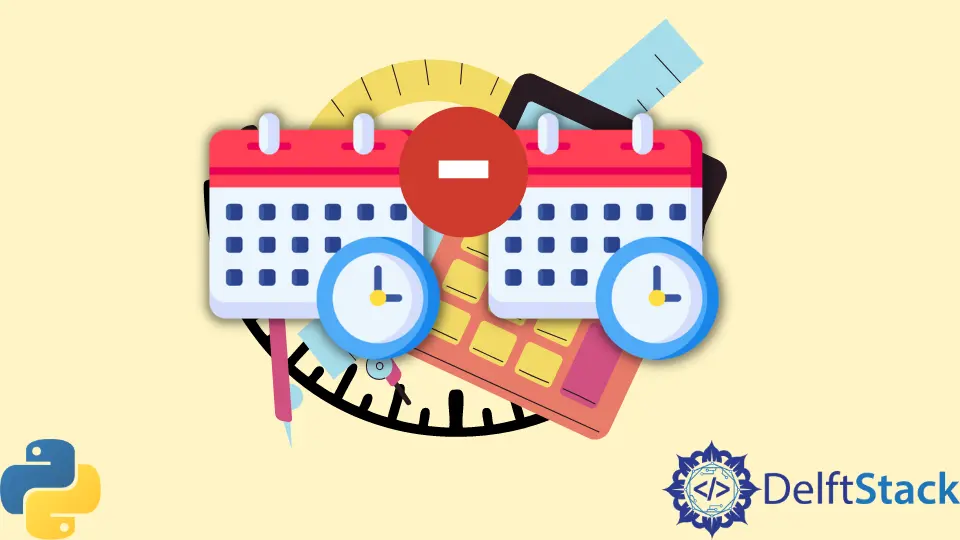
Questo tutorial introdurrà come eseguire la sottrazione datetime in Python.
Esploreremo diversi output dopo la sottrazione, come l’output del numero di secondi, minuti, ore o anni della differenza dei due oggetti datetime forniti.
Usa il modulo datetime
per sottrarre Datetime in Python
datetime
è un modulo in Python che supporterà funzioni che manipolano l’oggetto datetime
.
L’inizializzazione di un oggetto datetime
richiede tre parametri obbligatori come datetime.datetime(year, month, day)
. Accetta anche parametri opzionali sotto forma di ore, minuti, secondi, microsecondi e fuso orario.
datetime
ha anche la funzione now()
, che inizializza la data e l’ora correnti nell’oggetto.
La sottrazione di due oggetti datetime restituirà la differenza del numero di giorni e la differenza di tempo, se presente.
from datetime import datetime
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print(now - then)
Produzione:
12055 days, 5:10:00
Il risultato significa che le due date sono 12055 giorni e 5 ore e 10 minuti di distanza.
Per convertire questo risultato in diversi formati, dovremmo prima convertire il timedelta
in secondi. L’oggetto datetime ha un metodo incorporato datetime.total_seconds()
che converte l’oggetto nel totale dei secondi che contiene.
Ecco il codice sorgente completo per la sottrazione datetime utilizzando il modulo datetime
e le sue funzioni.
from datetime import datetime
def getDifference(then, now=datetime.now(), interval="secs"):
duration = now - then
duration_in_s = duration.total_seconds()
# Date and Time constants
yr_ct = 365 * 24 * 60 * 60 # 31536000
day_ct = 24 * 60 * 60 # 86400
hour_ct = 60 * 60 # 3600
minute_ct = 60
def yrs():
return divmod(duration_in_s, yr_ct)[0]
def days():
return divmod(duration_in_s, day_ct)[0]
def hrs():
return divmod(duration_in_s, hour_ct)[0]
def mins():
return divmod(duration_in_s, minute_ct)[0]
def secs():
return duration_in_s
return {
"yrs": int(yrs()),
"days": int(days()),
"hrs": int(hrs()),
"mins": int(mins()),
"secs": int(secs()),
}[interval]
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print("The difference in seconds:", getDifference(then, now, "secs"))
print("The difference in minutes:", getDifference(then, now, "mins"))
print("The difference in hours:", getDifference(then, now, "hrs"))
print("The difference in days:", getDifference(then, now, "days"))
print("The difference in years:", getDifference(then, now, "yrs"))
La prima cosa che fa il codice è inizializzare 2 date separate per la sottrazione e chiamare la funzione più volte con output diversi ogni volta. Nel caso di all
, restituirà un output che ottiene la differenza in base a tutti i casi da anni a secondi.
La funzione divmod()
accetta due numeri come dividendo e divisore e restituisce una tupla del quoziente e il resto tra di loro. È necessario solo il quoziente, ed è per questo che nel codice viene utilizzato solo l’indice 0
.
Produzione:
The difference in seconds: 1041052966
The difference in minutes: 17350882
The difference in hours: 289181
The difference in days: 12049
The difference in years: 33
Ora abbiamo la differenza tra due oggetti datetime in diversi intervalli di tempo diversi.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInArticolo correlato - Python DateTime
- Come convertire la colonna DataFrame in data e ora in pandas
- Come ottenere l'ora corrente in Python
- Ottieni il giorno della settimana in Python
- Come convertire una stringa in datario in Python
- Come ottenere l'anno da un oggetto datetime in Python
- Calcola la differenza di tempo tra due stringhe temporali in Python