Python で datetime 減算
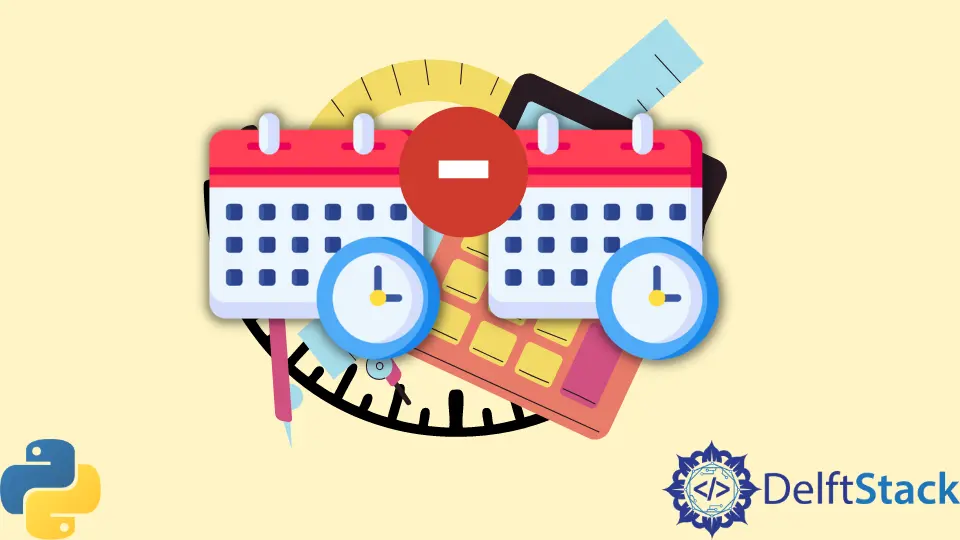
このチュートリアルでは、Python で datetime
の減算を実行する方法を紹介します。
例えば、与えられた 2つの datetime
オブジェクトの差分の秒数、分数、時間数、年数を出力するなど、減算後のさまざまな出力を探っていきます。
Python で datetime
を減算するために datetime
モジュールを使用する
datetime
は、datetime
オブジェクトを操作する関数をサポートする Python のモジュールです。
datetime
オブジェクトの初期化には、datetime.datetime(year, month, day)
という 3つのパラメータが必要です。また、オプションのパラメータとして、時、分、秒、マイクロ秒、タイムゾーンを指定することもできます。
これは現在の日付と時刻をオブジェクトに初期化します。
2つの datetime オブジェクトを引き算すると、日数と時間の差があればその差を返します。
from datetime import datetime
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print(now - then)
出力:
12055 days, 5:10:00
結果は、2つの日付が 12055 日で 5 時間 10 分離れていることを意味します。
この結果を異なる形式に変換するには、まず timedelta
を秒に変換する必要があります。datetime オブジェクトには組み込みのメソッド datetime.total_seconds()
があります。
以下に、datetime
モジュールとその関数を使った datetime 減算の完全なソースコードを示します。
from datetime import datetime
def getDifference(then, now=datetime.now(), interval="secs"):
duration = now - then
duration_in_s = duration.total_seconds()
# Date and Time constants
yr_ct = 365 * 24 * 60 * 60 # 31536000
day_ct = 24 * 60 * 60 # 86400
hour_ct = 60 * 60 # 3600
minute_ct = 60
def yrs():
return divmod(duration_in_s, yr_ct)[0]
def days():
return divmod(duration_in_s, day_ct)[0]
def hrs():
return divmod(duration_in_s, hour_ct)[0]
def mins():
return divmod(duration_in_s, minute_ct)[0]
def secs():
return duration_in_s
return {
"yrs": int(yrs()),
"days": int(days()),
"hrs": int(hrs()),
"mins": int(mins()),
"secs": int(secs()),
}[interval]
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print("The difference in seconds:", getDifference(then, now, "secs"))
print("The difference in minutes:", getDifference(then, now, "mins"))
print("The difference in hours:", getDifference(then, now, "hrs"))
print("The difference in days:", getDifference(then, now, "days"))
print("The difference in years:", getDifference(then, now, "yrs"))
このコードが最初に行うことは、減算のために 2つの別々の日付を初期化し、毎回異なる出力を持つ関数を複数回呼び出すことです。all
の場合、年から秒までのすべてのケースに基づいて差分を取得する出力を返します。
関数 divmod()
は 2つの数値を配当と除数として受け取り、商とその間の余りのタプルを返します。商だけが必要であり、コードを通して 0
のインデックスだけが使われるのはそのためです。
出力:
The difference in seconds: 1041052966
The difference in minutes: 17350882
The difference in hours: 289181
The difference in days: 12049
The difference in years: 33
これで、いくつかの異なる時間枠内の 2つの datetime
オブジェクト間の差が得られました。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn