在 Python 中对日期时间做减法
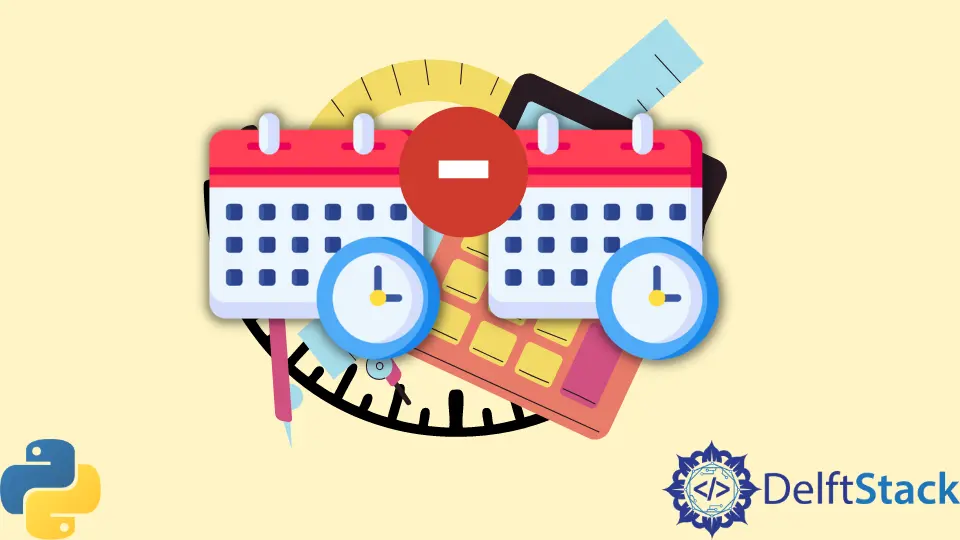
本教程将介绍如何在 Python 中执行日期时间减法。
我们了解减法后的不同输出,比如输出所提供的两个日期时间对象之差的秒数、分数、小时数或年数。
在 Python 中使用 datetime
模块减去日期时间
datetime
是 Python 中的一个模块,它将支持操作 datetime
对象的函数。
初始化一个 datetime
对象需要三个必要的参数,即 datetime.datetime(year, month, day)
。它还接受时、分、秒、微秒和时区等形式的可选参数。
datetime
也有函数 now()
,它将当前日期和时间初始化到对象中。
减去两个 datetime 对象将返回天数的差值和时间的差值(如果有的话)。
from datetime import datetime
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print(now - then)
输出:
12055 days, 5:10:00
结果意味着两个日期相差 1205 天,5 小时 10 分钟。
要把这个结果转换成不同的格式,我们首先要把 timedelta
转换成秒。datetime 对象有一个内置的方法 datetime.total_seconds()
将对象转换成它所包含的总秒数。
下面是使用 datetime
模块及其函数进行日期时间减法的完整源代码。
from datetime import datetime
def getDifference(then, now=datetime.now(), interval="secs"):
duration = now - then
duration_in_s = duration.total_seconds()
# Date and Time constants
yr_ct = 365 * 24 * 60 * 60 # 31536000
day_ct = 24 * 60 * 60 # 86400
hour_ct = 60 * 60 # 3600
minute_ct = 60
def yrs():
return divmod(duration_in_s, yr_ct)[0]
def days():
return divmod(duration_in_s, day_ct)[0]
def hrs():
return divmod(duration_in_s, hour_ct)[0]
def mins():
return divmod(duration_in_s, minute_ct)[0]
def secs():
return duration_in_s
return {
"yrs": int(yrs()),
"days": int(days()),
"hrs": int(hrs()),
"mins": int(mins()),
"secs": int(secs()),
}[interval]
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print("The difference in seconds:", getDifference(then, now, "secs"))
print("The difference in minutes:", getDifference(then, now, "mins"))
print("The difference in hours:", getDifference(then, now, "hrs"))
print("The difference in days:", getDifference(then, now, "days"))
print("The difference in years:", getDifference(then, now, "yrs"))
代码的第一件事是初始化 2 个独立的日期进行减法,并多次调用该函数,每次的输出都不同。在 all
的情况下,它会返回一个输出,根据从年到秒的所有情况得到差值。
divmod()
函数接受两个数作为被除数和除数,并返回它们之间的商和余数的元组。只有商是需要的,这也是为什么在代码中只使用 0
指数的原因。
输出:
The difference in seconds: 1041052966
The difference in minutes: 17350882
The difference in hours: 289181
The difference in days: 12049
The difference in years: 33
现在我们有了几个不同时间范围内两个日期时间对象之间的差值。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn