Python 中带有时区的日期时间对象
-
在 Python 中使用带有
timezone
的datetime
对象 -
在 Python 中使用
tzinfo
和timezone
类 - Python 中日期时间对象的属性
- Python 中日期时间对象的类型
-
Python 中需要时区
datatime aware
对象 -
通过 Python 中的
tzinfo
检查对象是否timezone aware
-
在 Python 中创建
timezone aware
日期时间对象 - 结论
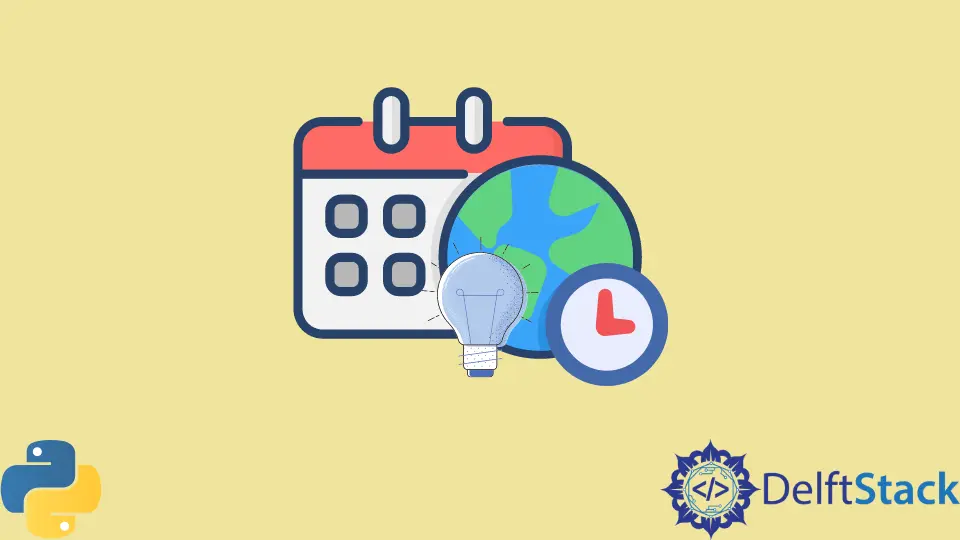
本指南将带你了解 datetime
模块,教你如何使用日期和时间来检查和创建 timezone-aware
python 对象。
在 Python 中使用带有 timezone
的 datetime
对象
Python 附带一个包含的模块,其中包含你可以在编码时使用的日期和时间。这些是对象,这意味着修改它们会改变对象,而不是实际的日期或时间。
以下提供了各种用于格式化或操作以下内容的类,以便在你的代码中使用。
日期
:年-月-日
格式的当前日期。最好记住日期时间中允许的最小和最大年份是 1 和 9999。
MINYEAR 和 MAXYEAR 代表这些。同样,最小日期为 1,1,1,最大日期为 9999,12,31,分别由 date.min
和 date.max
表示。
from datetime import date
# Date specified by user
supplied = date(2022, 1, 30)
# Today's date called
current = date.today()
print("Your date: ")
print(supplied)
print("Current: ")
print(current)
输出:
Your date:
2022-01-30
Current:
2022-01-29
Time
:一天中的当前时间。时间
不遵循闰秒,通过将 24 小时乘以 60 分钟和每分钟再乘以 60 秒,将每天分类为 86,400 秒。
显示的时间显示从小时到微秒(范围在 0 到 1000000 之间)和 tzinfo
的时间。
from datetime import time
# Specifying time using constructor
t = time(17, 14, 47)
print("Your time: ", t)
输出:
Your time: 17:14:47
日期时间
:日期时间
类返回当前日期和时间的组合。
from datetime import datetime
# Date and time specified both
moment = datetime(2022, 1, 30, 10, 30, 45, 812210)
print("My moment: ", moment)
# Calling now() function
current = datetime.now()
print("Current Datetime: ", current)
输出:
My moment: 2022-01-30 10:30:45.812210
Current Datetime: 2022-01-29 05:00:50.742904
TimeDelta
:timedelta
返回两个times
、dates
或datetimes
之间的差异。它用于计算两个不同时间之间的持续时间。
from datetime import datetime, timedelta
# Current
current = datetime.now()
print("Current: ", str(current))
# Future time
future = current + timedelta(days=4)
# printing future time
print("After 4 days: ", str(future))
# printing time jump
print("Jumped Time:", str(future - current))
输出:
Current: 2022-01-29 05:01:54.844705
After 4 days: 2022-02-02 05:01:54.844705
Jumped Time: 4 days, 0:00:00
在 Python 中使用 tzinfo
和 timezone
类
tzinfo
是 Python 中的一个抽象类,允许根据国际标准操纵时间。你可以使用此类来描述特定的时区
,例如 DST、GMT 等,或时间偏移。
timezone
类是另一个抽象类,你可以使用它来提供与时间标准的偏移量以调节你的时钟对象。指定 timezones
和 tzinfo
可能会很棘手,因此你应该安装 pytz
模块。
pytz
模块在其数据库中包含所有 timezone
,可轻松指定使用和时间增量计算。你可以在命令行中使用 pip
安装它:
pip install pytz
安装后,你可以开始使用 pytz
。
import datetime
import pytz
# Defining a new datetime with time from US Pacific
UStime = datetime.datetime.now(pytz.timezone("US/Pacific"))
print(UStime)
输出:
2022-01-28 21:04:50.440392-08:00
你可以使用 pytz.all_timezones
在 pytz
模块中找到所有可用的时区
Python 中日期时间对象的属性
在 python 中使用上述类的对象具有特定的属性。
- 这些是不可变的。
- 这些是可散列的。
- 这些支持 pickling。
Python 中日期时间对象的类型
你可以将 Python 中的所有日期时间对象分为两种类型; aware
和 naive
对象。
aware
对象可以相对于所有其他感知对象定位自己。它表示无法解释的特定时间点。
naive
对象与 aware
对象相反。naive
的对象缺乏意识;即,它缺乏适当的信息,因此不能将自己定位于相似的对象,但 naive
对象很容易使用。
Python 中需要时区 datatime aware
对象
python 中的 datatime aware
对象对于模拟现实世界的情况是必要的。在设计需要跟踪全球多个时区的软件或应用程序时,正确配置时间可能具有挑战性。
Datetime
模块和 aware
对象允许你参考不同日期的不规则时区,并根据 GMT、DST 等设置时间以获得最佳功能。
请记住使用 pytz
模块,因为它包含所有时区的数据库,并且可以简化你的编码。
通过 Python 中的 tzinfo
检查对象是否 timezone aware
由于 tzinfo
是一个抽象类,你可以在其中指定要遵循的 timezone
,aware
对象将具有指定的时区,而 naive
对象将设置为 None
。
# Importing the datetime module
import datetime
current = datetime.datetime.now()
# tzinfo is None
if current.tzinfo == None or current.tzinfo.utcoffset(current) == None:
print("The object is not Aware")
else:
print("The object is Aware")
输出:
The object is not Aware
在 Python 中创建 timezone aware
日期时间对象
你可以通过为其 tzinfo
指定时区或 UTC 偏移轻松创建 timezone aware
对象。
import datetime
# Current Date taken
current = datetime.datetime.now()
# Changing current's tzinfo
current = current.replace(tzinfo=datetime.timezone.utc)
# Printing Date
print(current)
# Checking if tzinfo is None or not
if current.tzinfo == None or current.tzinfo.utcoffset(current) == None:
print("The object is not Aware")
else:
print("The object is Aware")
输出:
2022-01-29 05:14:49.064797+00:00
The object is Aware
aware
对象的上述代码与前一个类似。我们使用 datetime.now()
来指定一个新对象。
唯一的添加是用于将当前对象的 tzinfo
替换为 UTC 的 replace()
函数,这会更改 tzinfo
,使对象 aware
。
结论
现在我们了解了 datetime
模块的概念,如何使用该模块在代码中使用日期和时间,aware
和 naive
对象之间的区别,并创建 timezone-aware
对象。