Python 中帶有時區的日期時間物件
-
在 Python 中使用帶有
timezone
的datetime
物件 -
在 Python 中使用
tzinfo
和timezone
類 - Python 中日期時間物件的屬性
- Python 中日期時間物件的型別
-
Python 中需要時區
datatime aware
物件 -
通過 Python 中的
tzinfo
檢查物件是否timezone aware
-
在 Python 中建立
timezone aware
日期時間物件 - まとめ
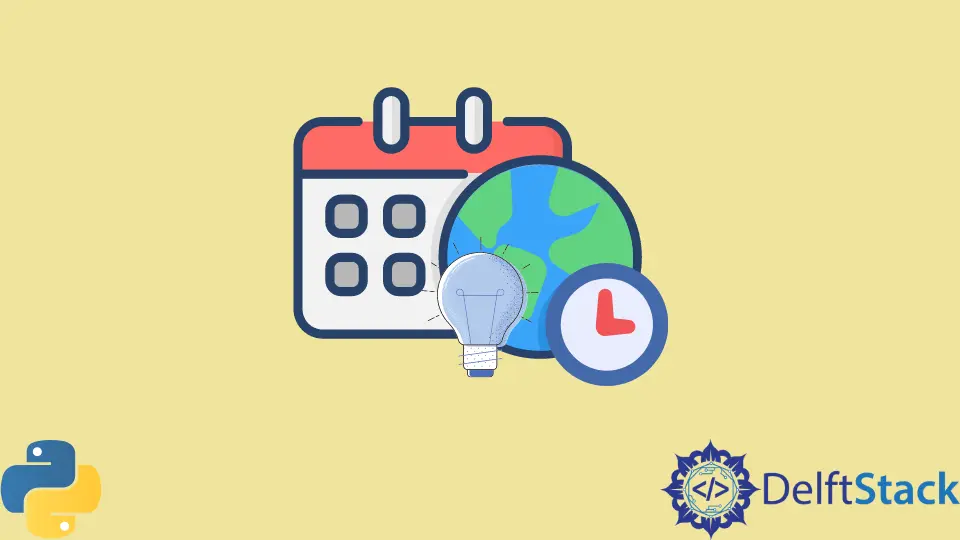
本指南將帶你瞭解 datetime
模組,教你如何使用日期和時間來檢查和建立 timezone-aware
python 物件。
在 Python 中使用帶有 timezone
的 datetime
物件
Python 附帶一個包含的模組,其中包含你可以在編碼時使用的日期和時間。這些是物件,這意味著修改它們會改變物件,而不是實際的日期或時間。
以下提供了各種用於格式化或操作以下內容的類,以便在你的程式碼中使用。
日期
:年-月-日
格式的當前日期。最好記住日期時間中允許的最小和最大年份是 1 和 9999。
MINYEAR 和 MAXYEAR 代表這些。同樣,最小日期為 1,1,1,最大日期為 9999,12,31,分別由 date.min
和 date.max
表示。
from datetime import date
# Date specified by user
supplied = date(2022, 1, 30)
# Today's date called
current = date.today()
print("Your date: ")
print(supplied)
print("Current: ")
print(current)
輸出:
Your date:
2022-01-30
Current:
2022-01-29
Time
:一天中的當前時間。時間
不遵循閏秒,通過將 24 小時乘以 60 分鐘和每分鐘再乘以 60 秒,將每天分類為 86,400 秒。
顯示的時間顯示從小時到微秒(範圍在 0 到 1000000 之間)和 tzinfo
的時間。
from datetime import time
# Specifying time using constructor
t = time(17, 14, 47)
print("Your time: ", t)
輸出:
Your time: 17:14:47
日期時間
:日期時間
類返回當前日期和時間的組合。
from datetime import datetime
# Date and time specified both
moment = datetime(2022, 1, 30, 10, 30, 45, 812210)
print("My moment: ", moment)
# Calling now() function
current = datetime.now()
print("Current Datetime: ", current)
輸出:
My moment: 2022-01-30 10:30:45.812210
Current Datetime: 2022-01-29 05:00:50.742904
TimeDelta
:timedelta
返回兩個times
、dates
或datetimes
之間的差異。它用於計算兩個不同時間之間的持續時間。
from datetime import datetime, timedelta
# Current
current = datetime.now()
print("Current: ", str(current))
# Future time
future = current + timedelta(days=4)
# printing future time
print("After 4 days: ", str(future))
# printing time jump
print("Jumped Time:", str(future - current))
輸出:
Current: 2022-01-29 05:01:54.844705
After 4 days: 2022-02-02 05:01:54.844705
Jumped Time: 4 days, 0:00:00
在 Python 中使用 tzinfo
和 timezone
類
tzinfo
是 Python 中的一個抽象類,允許根據國際標準操縱時間。你可以使用此類來描述特定的時區
,例如 DST、GMT 等,或時間偏移。
timezone
類是另一個抽象類,你可以使用它來提供與時間標準的偏移量以調節你的時鐘物件。指定 timezones
和 tzinfo
可能會很棘手,因此你應該安裝 pytz
模組。
pytz
模組在其資料庫中包含所有 timezone
,可輕鬆指定使用和時間增量計算。你可以在命令列中使用 pip
安裝它:
pip install pytz
安裝後,你可以開始使用 pytz
。
import datetime
import pytz
# Defining a new datetime with time from US Pacific
UStime = datetime.datetime.now(pytz.timezone("US/Pacific"))
print(UStime)
輸出:
2022-01-28 21:04:50.440392-08:00
你可以使用 pytz.all_timezones
在 pytz
模組中找到所有可用的時區
Python 中日期時間物件的屬性
在 python 中使用上述類的物件具有特定的屬性。
- 這些是不可變的。
- 這些是可雜湊的。
- 這些支援 pickling。
Python 中日期時間物件的型別
你可以將 Python 中的所有日期時間物件分為兩種型別; aware
和 naive
物件。
aware
物件可以相對於所有其他感知物件定位自己。它表示無法解釋的特定時間點。
naive
物件與 aware
物件相反。naive
的物件缺乏意識;即,它缺乏適當的資訊,因此不能將自己定位於相似的物件,但 naive
物件很容易使用。
Python 中需要時區 datatime aware
物件
python 中的 datatime aware
物件對於模擬現實世界的情況是必要的。在設計需要跟蹤全球多個時區的軟體或應用程式時,正確配置時間可能具有挑戰性。
Datetime
模組和 aware
物件允許你參考不同日期的不規則時區,並根據 GMT、DST 等設定時間以獲得最佳功能。
請記住使用 pytz
模組,因為它包含所有時區的資料庫,並且可以簡化你的編碼。
通過 Python 中的 tzinfo
檢查物件是否 timezone aware
由於 tzinfo
是一個抽象類,你可以在其中指定要遵循的 timezone
,aware
物件將具有指定的時區,而 naive
物件將設定為 None
。
# Importing the datetime module
import datetime
current = datetime.datetime.now()
# tzinfo is None
if current.tzinfo == None or current.tzinfo.utcoffset(current) == None:
print("The object is not Aware")
else:
print("The object is Aware")
輸出:
The object is not Aware
在 Python 中建立 timezone aware
日期時間物件
你可以通過為其 tzinfo
指定時區或 UTC 偏移輕鬆建立 timezone aware
物件。
import datetime
# Current Date taken
current = datetime.datetime.now()
# Changing current's tzinfo
current = current.replace(tzinfo=datetime.timezone.utc)
# Printing Date
print(current)
# Checking if tzinfo is None or not
if current.tzinfo == None or current.tzinfo.utcoffset(current) == None:
print("The object is not Aware")
else:
print("The object is Aware")
輸出:
2022-01-29 05:14:49.064797+00:00
The object is Aware
aware
物件的上述程式碼與前一個類似。我們使用 datetime.now()
來指定一個新物件。
唯一的新增是用於將當前物件的 tzinfo
替換為 UTC 的 replace()
函式,這會更改 tzinfo
,使物件 aware
。
まとめ
現在我們瞭解了 datetime
模組的概念,如何使用該模組在程式碼中使用日期和時間,aware
和 naive
物件之間的區別,並建立 timezone-aware
物件。