How to Datetime Objects With Timezone in Python
-
Using
datetime
Objects Withtimezone
in Python -
Using the
tzinfo
andtimezone
Class in Python - Properties of Datetime Objects in Python
- Types of Datetime Objects in Python
-
Need for Timezone
datetime aware
Objects in Python -
Check if an Object Is
timezone aware
by Itstzinfo
in Python -
Create
timezone aware
Datetime Objects in Python - Conclusion
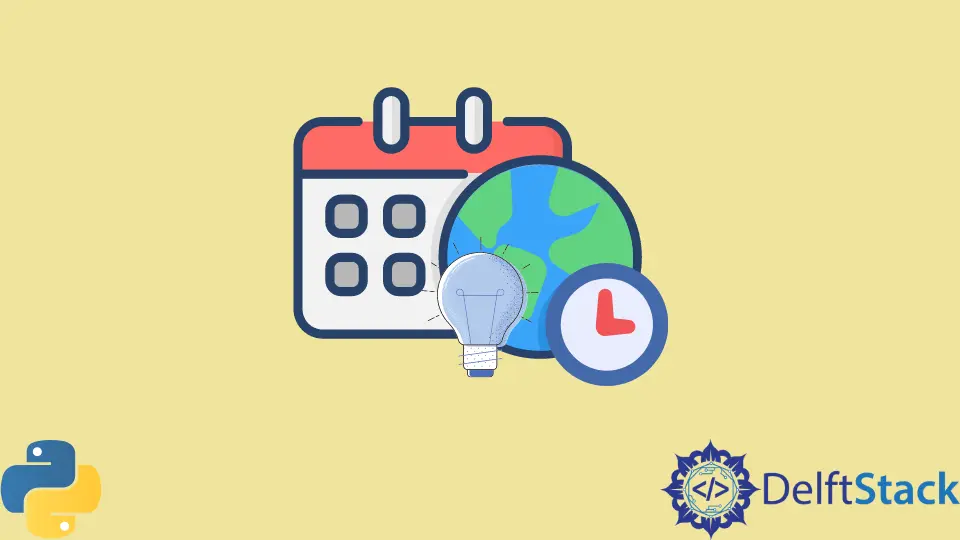
This guide will take you through the datetime
module, teaching you how to work with dates and times to check and create timezone-aware
python objects.
Using datetime
Objects With timezone
in Python
Python comes with an included module that contains dates and times that you can use while coding. These are objects, which means that modifying them alters the objects and not the actual date or time.
The following provides various classes for formatting or manipulating the following for usage in your code.
Date
: The current date inYear-Month-Date
format. It would be best to remember that the minimum and maximum years allowed in datetime are 1 and 9999.
MINYEAR and MAXYEAR represent these. Similarly, the minimum date is 1,1,1, and the maximum is 9999,12,31 represented by date.min
and date.max
respectively.
from datetime import date
# Date specified by user
supplied = date(2022, 1, 30)
# Today's date called
current = date.today()
print("Your date: ")
print(supplied)
print("Current: ")
print(current)
Output:
Your date:
2022-01-30
Current:
2022-01-29
Time
: The current time of the day.Time
does not follow leap seconds and classifies each day as 86,400 seconds long by multiplication of 24 hours into 60 minutes and each minute into a further 60 seconds.
The time displayed shows time starting from hours and going up to microseconds (ranging between 0 and 1000000), and tzinfo
.
from datetime import time
# Specifying time using constructor
t = time(17, 14, 47)
print("Your time: ", t)
Output:
Your time: 17:14:47
Datetime
: Thedatetime
class returns a combination of the current day and time.
from datetime import datetime
# Date and time specified both
moment = datetime(2022, 1, 30, 10, 30, 45, 812210)
print("My moment: ", moment)
# Calling now() function
current = datetime.now()
print("Current Datetime: ", current)
Output:
My moment: 2022-01-30 10:30:45.812210
Current Datetime: 2022-01-29 05:00:50.742904
TimeDelta
: Thetimedelta
returns the difference between twotimes
,dates
, ordatetimes
. It is used for calculating the duration between two different times.
from datetime import datetime, timedelta
# Current
current = datetime.now()
print("Current: ", str(current))
# Future time
future = current + timedelta(days=4)
# printing future time
print("After 4 days: ", str(future))
# printing time jump
print("Jumped Time:", str(future - current))
Output:
Current: 2022-01-29 05:01:54.844705
After 4 days: 2022-02-02 05:01:54.844705
Jumped Time: 4 days, 0:00:00
Using the tzinfo
and timezone
Class in Python
The tzinfo
is an abstract class in Python that allows for manipulating time according to international standards. You can use this class for describing a specific timezone
such as DST, GMT, etc., or a time offset.
The timezone
class is another abstract class that you can use to provide offsets from the Time Standards to regulate your clock object. Specifying timezones
and tzinfo
can be tricky, so you should install the pytz
module.
The pytz
module includes all timezones
in its database, easily specified for usage and time delta calculations. You can install it by using pip
in the command line as:
pip install pytz
Once installed, you can start working with pytz
.
import datetime
import pytz
# Defining a new datetime with time from US Pacific
UStime = datetime.datetime.now(pytz.timezone("US/Pacific"))
print(UStime)
Output:
2022-01-28 21:04:50.440392-08:00
You can find all the timezones
available in the pytz
module using pytz.all_timezones
Properties of Datetime Objects in Python
The objects using the above classes in python have specific properties.
- These are immutable.
- These are hashable.
- These support pickling.
Types of Datetime Objects in Python
You can categorize all datetime objects in Python into two types; aware
and naive
objects.
An aware
object can situate itself relatively to all other aware objects. It denotes a specific point in time that cannot be interpreted.
A naive
object is the opposite of an aware
object. A naive
object lacks awareness; i.e., it lacks proper information and hence cannot situate itself to similar objects, but naive
objects are easy to work with.
Need for Timezone datetime aware
Objects in Python
Datetime aware
objects in python are necessary to mimic the real world’s circumstances. While designing software or applications that need to track several timezones across the globe, properly configuring the time can be challenging.
Datetime
module and aware
objects allow you to refer to the irregular timezones of different dates and set the time according to GMT, DST, and more for best functionality.
Remember to use the pytz
module as it contains a database of all timezones and can ease your coding.
Check if an Object Is timezone aware
by Its tzinfo
in Python
Since tzinfo
is an abstract class where you can specify a timezone
to follow, aware
objects will have a specified timezone, whereas naive
objects will be set to None
.
# Importing the datetime module
import datetime
current = datetime.datetime.now()
# tzinfo is None
if current.tzinfo == None or current.tzinfo.utcoffset(current) == None:
print("The object is not Aware")
else:
print("The object is Aware")
Output:
The object is not Aware
Create timezone aware
Datetime Objects in Python
You can easily create timezone aware
objects by specifying a timezone or UTC offset to its tzinfo
.
import datetime
# Current Date taken
current = datetime.datetime.now()
# Changing current's tzinfo
current = current.replace(tzinfo=datetime.timezone.utc)
# Printing Date
print(current)
# Checking if tzinfo is None or not
if current.tzinfo == None or current.tzinfo.utcoffset(current) == None:
print("The object is not Aware")
else:
print("The object is Aware")
Output:
2022-01-29 05:14:49.064797+00:00
The object is Aware
The above code for the aware
objects is similar to the previous one. We use datetime.now()
to specify a new object.
The only addition is the replace()
function for replacing the current object’s tzinfo
to UTC, which changes the tzinfo
, making the object aware
.
Conclusion
Now we understand the concept of the datetime
module, how you can use the module to use dates and times with your code, the difference between aware
and naive
objects, and create timezone-aware
objects.