在 Python 中對日期時間做減法
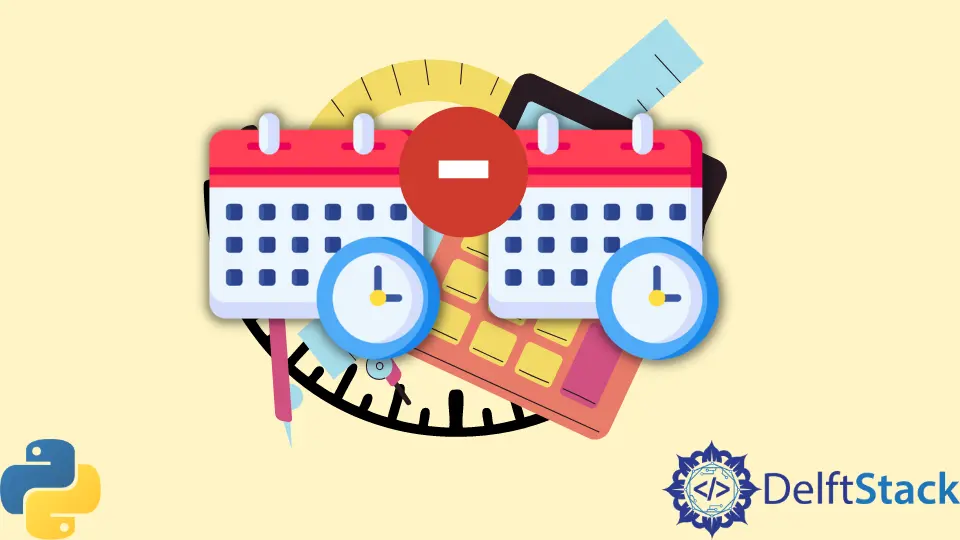
本教程將介紹如何在 Python 中執行日期時間減法。
我們瞭解減法後的不同輸出,比如輸出所提供的兩個日期時間物件之差的秒數、分數、小時數或年數。
在 Python 中使用 datetime
模組減去日期時間
datetime
是 Python 中的一個模組,它將支援操作 datetime
物件的函式。
初始化一個 datetime
物件需要三個必要的引數,即 datetime.datetime(year, month, day)
。它還接受時、分、秒、微秒和時區等形式的可選引數。
datetime
也有函式 now()
,它將當前日期和時間初始化到物件中。
減去兩個 datetime 物件將返回天數的差值和時間的差值(如果有的話)。
from datetime import datetime
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print(now - then)
輸出:
12055 days, 5:10:00
結果意味著兩個日期相差 1205 天,5 小時 10 分鐘。
要把這個結果轉換成不同的格式,我們首先要把 timedelta
轉換成秒。datetime 物件有一個內建的方法 datetime.total_seconds()
將物件轉換成它所包含的總秒數。
下面是使用 datetime
模組及其函式進行日期時間減法的完整原始碼。
from datetime import datetime
def getDifference(then, now=datetime.now(), interval="secs"):
duration = now - then
duration_in_s = duration.total_seconds()
# Date and Time constants
yr_ct = 365 * 24 * 60 * 60 # 31536000
day_ct = 24 * 60 * 60 # 86400
hour_ct = 60 * 60 # 3600
minute_ct = 60
def yrs():
return divmod(duration_in_s, yr_ct)[0]
def days():
return divmod(duration_in_s, day_ct)[0]
def hrs():
return divmod(duration_in_s, hour_ct)[0]
def mins():
return divmod(duration_in_s, minute_ct)[0]
def secs():
return duration_in_s
return {
"yrs": int(yrs()),
"days": int(days()),
"hrs": int(hrs()),
"mins": int(mins()),
"secs": int(secs()),
}[interval]
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print("The difference in seconds:", getDifference(then, now, "secs"))
print("The difference in minutes:", getDifference(then, now, "mins"))
print("The difference in hours:", getDifference(then, now, "hrs"))
print("The difference in days:", getDifference(then, now, "days"))
print("The difference in years:", getDifference(then, now, "yrs"))
程式碼的第一件事是初始化 2 個獨立的日期進行減法,並多次呼叫該函式,每次的輸出都不同。在 all
的情況下,它會返回一個輸出,根據從年到秒的所有情況得到差值。
divmod()
函式接受兩個數作為被除數和除數,並返回它們之間的商和餘數的元組。只有商是需要的,這也是為什麼在程式碼中只使用 0
指數的原因。
輸出:
The difference in seconds: 1041052966
The difference in minutes: 17350882
The difference in hours: 289181
The difference in days: 12049
The difference in years: 33
現在我們有了幾個不同時間範圍內兩個日期時間物件之間的差值。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn