How to Convert Hex to Byte in Python
- Initialize a Hexadecimal Value
-
Use the
bytes.fromhex()
Function to Convert Hex to Byte in Python -
Use the
binascii
Module to Convert a Hex to Byte in Python -
Use the
codecs.decode()
Method to Convert a Hex to Byte in Python -
Use List Comprehension and the
int()
Function to Convert a Hex to Byte in Python - Conclusion
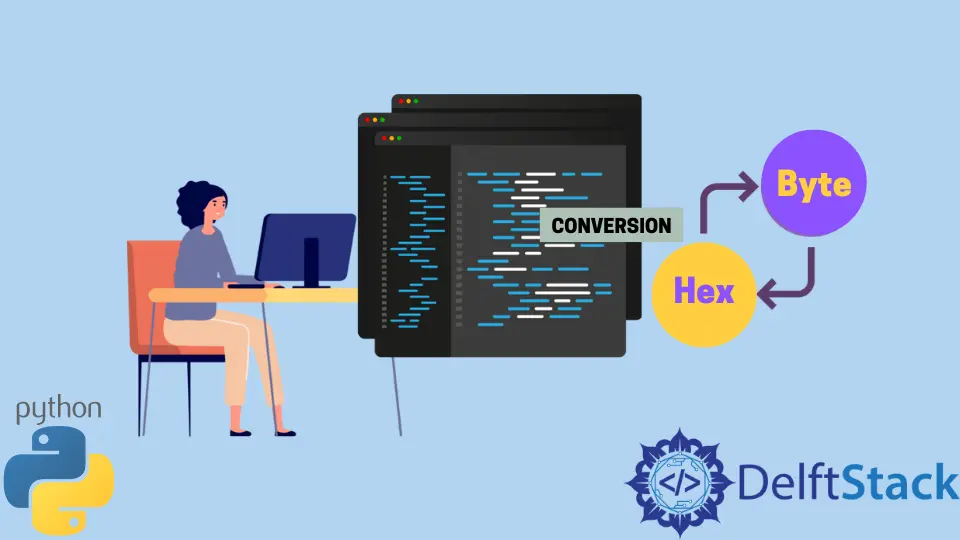
Hexadecimal, often abbreviated as hex, uses 16 symbols (0-9, a-f) to represent values, contrasting with decimal’s 10 symbols. For instance, 1000 in decimal is 3E8 in hex.
Proficiency in handling hex is crucial for programming tasks involving binary data, memory addresses, and low-level encoding. This tutorial will introduce how to convert hexadecimal values into a byte literal in Python.
Initialize a Hexadecimal Value
Let’s create a hexadecimal value using a string and convert the phrase A quick brown fox
into a hex value using the hexlify()
function in the binascii
module.
To convert a string into hexadecimal, we first need to convert the string into bytes.
import binascii
str_val = "A quick brown fox".encode("utf-8")
hex_val = binascii.hexlify(str_val).decode("utf-8")
print(hex_val)
Output:
4120717569636b2062726f776e20666f78
Now, we’ve successfully converted a string into hexadecimal. Next, let’s proceed to convert hexadecimal back into bytes.
Use the bytes.fromhex()
Function to Convert Hex to Byte in Python
The bytes.fromhex()
method is designed to convert a valid hexadecimal string into a bytes
object. It has the following syntax:
bytes.fromhex(hex_string)
hex_string
: This is a required argument and represents the input hexadecimal string that you want to convert into a byte literal.
Here’s how the bytes.fromhex()
method works:
- Input Validation: The method first validates the input
hex_string
to ensure that it contains only valid hexadecimal characters (digits 0-9 and lowercase or uppercase letters A-F). If the string contains any other characters, it will raise aValueError
. - Parsing Pairs: The method processes the hexadecimal string two characters at a time, treating each pair of characters as a single hexadecimal byte. This means that for every two characters in the input string, one byte is generated in the resulting
bytes
object. - Conversion to Bytes: Each pair of hexadecimal characters is converted into its binary equivalent.
- Constructing the
bytes
Object: As the method processes the pairs of hexadecimal characters, it constructs abytes
object by appending the binary representation of each byte. This process continues until the entire input string has been processed. - Return Value: Once the entire input string has been processed, the method returns the resulting
bytes
object, which represents the byte literal equivalent of the input hexadecimal string.
As an example, we will take the hex value from the previous result and use fromhex()
to convert it into a byte literal.
hex_val = "4120717569636b2062726f776e20666f78"
print(bytes.fromhex(hex_val))
Output:
b'A quick brown fox'
Let’s break down the example hexadecimal string 4120717569636b2062726f776e20666f78
to better understand how it’s converted into a byte literal:
41
becomes the byte 65 (ASCII value of'A'
).20
becomes the byte 32 (ASCII value of space).71
becomes the byte 113 (ASCII value of'q'
).75
becomes the byte 117 (ASCII value of'u'
).69
becomes the byte 105 (ASCII value of'i'
).63
becomes the byte 99 (ASCII value of'c'
).6b
becomes the byte 107 (ASCII value of'k'
).20
becomes the byte 32 (ASCII value of space).62
becomes the byte 98 (ASCII value of'b'
).72
becomes the byte 114 (ASCII value of'r'
).6f
becomes the byte 111 (ASCII value of'o'
).77
becomes the byte 119 (ASCII value of'w'
).6e
becomes the byte 110 (ASCII value of'n'
).20
becomes the byte 32 (ASCII value of space).66
becomes the byte 102 (ASCII value of'f'
).6f
becomes the byte 111 (ASCII value of'o'
).78
becomes the byte 120 (ASCII value of'x'
).
When all these bytes are combined, you get the byte literal A quick brown fox
, which is the final result.
Use the binascii
Module to Convert a Hex to Byte in Python
The binascii
Python module contains efficient utility functions for binary and ASCII operations. Particularly, unhexlify()
is a function within the binascii
module that converts a hexadecimal value into a byte literal.
This function has the following syntax:
binascii.unhexlify(hex_string)
hex_string
: This is the required argument representing the input hexadecimal string that you want to convert into a byte literal.
Here’s a detailed explanation of how it works:
- Input Validation: The method starts by validating the
hex_string
argument to ensure it contains only valid hexadecimal characters. Any invalid characters in the input string will result in abinascii.Error
exception. - Hexadecimal Pair Conversion: Next, the method processes the
hex_string
in pairs of characters. For example,41
represents the byte with the integer value 65 (ASCII value ofA
). - Conversion to Bytes: The hexadecimal byte pairs are converted into their binary representation. Each pair is transformed into a corresponding byte, with each character representing 4 bits.
- Constructing the Byte Literal: As the method processes each pair, it appends the resulting binary bytes to form a complete byte literal. This process continues until the entire input string has been processed.
- Return Value: Once the entire
hex_string
has been processed, theunhexlify()
function returns the resulting byte literal, represented as abytes
object.
Let’s initialize a new example with special non-ASCII characters, which will then be converted into a hexadecimal value. The example will be the Greek translation of the phrase A quick brown fox
.
import binascii
from binascii import unhexlify
str_val = "Μια γρήγορη καφέ αλεπού".encode(
"utf-8"
) # A quick brown fox in Greek translation
hex_val = binascii.hexlify(str_val).decode("utf-8")
print("String value: ", str_val.decode("utf-8"))
print("Hexadecimal: ", hex_val)
print("Byte value: ", unhexlify(hex_val))
Output:
String value: Μια γρήγορη καφέ αλεπού
Hexadecimal: ce9cceb9ceb120ceb3cf81ceaeceb3cebfcf81ceb720cebaceb1cf86cead20ceb1cebbceb5cf80cebfcf8d
Byte value: b'\xce\x9c\xce\xb9\xce\xb1 \xce\xb3\xcf\x81\xce\xae\xce\xb3\xce\xbf\xcf\x81\xce\xb7 \xce\xba\xce\xb1\xcf\x86\xce\xad \xce\xb1\xce\xbb\xce\xb5\xcf\x80\xce\xbf\xcf\x8d'
We have now successfully converted hexadecimal values to bytes.
Use the codecs.decode()
Method to Convert a Hex to Byte in Python
The codecs.decode()
function can also be used to convert a hexadecimal string into bytes. It’s part of Python’s codecs
module, which provides various encoding and decoding functionalities for different data representations.
It has the following syntax:
codecs.decode(data, encoding, errors="strict")
data
: This is the required argument representing the data that you want to decode. In this case, it should be the hexadecimal string you wish to convert into a byte literal.encoding
: This is also a required argument specifying the encoding to use for decoding. When working with hexadecimal strings, you should use thehex
encoding to indicate that the input string represents a hexadecimal value.errors
(optional): This argument specifies how to handle decoding errors, such as when the input string contains invalid characters for the specified encoding. It has a default value ofstrict
, but you can change it toignore
orreplace
to handle errors differently.
Here’s a detailed explanation of how this method works when used for decoding hexadecimal values:
- Input Validation: The method first checks the validity of the
data
argument to ensure it contains only valid hexadecimal characters. If the input string contains any non-hexadecimal characters, it will raise aUnicodeDecodeError
with details about the problematic character. - Decoding Process: The
codecs.decode()
function interprets thedata
argument as a hexadecimal string and converts it into binary data. It processes the input string in pairs of characters, treating each pair as a single hexadecimal byte. - Encoding Specification: The
encoding
argument is crucial in this context. When you specify'hex'
as the encoding, it tells Python to interpret the input string as a hexadecimal value and convert it accordingly. - Conversion to Bytes: As the method processes each pair of hexadecimal characters, it converts them into their binary representation. This transformation results in the creation of a byte literal, where each pair of characters corresponds to a single byte.
- Return Value: The
codecs.decode()
method returns the resulting byte literal as abytes
object, representing the decoded binary data.
See the example below:
import codecs
hex_val = "4120717569636b2062726f776e20666f78"
byte_val = codecs.decode(hex_val, "hex")
print(byte_val)
This program decodes the hex_val
string using the codecs.decode()
function with the encoding argument set to 'hex'
. This means it will interpret the input string as a hexadecimal value and convert it to bytes.
Output:
b'A quick brown fox'
Use List Comprehension and the int()
Function to Convert a Hex to Byte in Python
This method involves using list comprehension and the int()
function to convert a hexadecimal string into bytes.
hex_val = "4120717569636b2062726f776e20666f78"
byte_val = bytes([int(hex_val[i : i + 2], 16) for i in range(0, len(hex_val), 2)])
print(byte_val)
Here, the code uses a list comprehension to iterate over the hexadecimal string in pairs of two characters, converts each pair to an integer in base 16 (hexadecimal), and creates a list of these integers.
The bytes()
constructor is then used to convert this list of integers into a bytes object.
Output:
b'A quick brown fox'
This method is especially useful when we have a hexadecimal string with a known structure and we want to convert it into bytes efficiently. It provides control over the conversion process by allowing us to specify the encoding base (in this case, base 16 for hexadecimal).
Conclusion
In this article, we’ve covered a few ways to convert hexadecimal values to byte literals in Python.
The fromhex()
function is preferable if you don’t want added imports into your source code. Otherwise, you can choose the method that best suits your needs and coding style.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python Bytes
- How to Convert Bytes to Int in Python 2.7 and 3.x
- How to Convert Int to Bytes in Python 2 and Python 3
- How to Convert Int to Binary in Python
- How to Convert Bytes to String in Python 2 and Python 3
- How to Convert String to Bytes in Python
- B in Front of String in Python