How to Convert Byte to Hex in Python
- Initializing a Byte Literal in Python
-
Use the
hex()
Method to Convert a Byte to Hex in Python -
Use the
binascii
Module to Convert a Byte to Hex in Python -
Use the
codecs
Module to Convert a Byte to Hex in Python -
Use the
struct
Module to Convert a Byte to Hex in Python - Conclusion
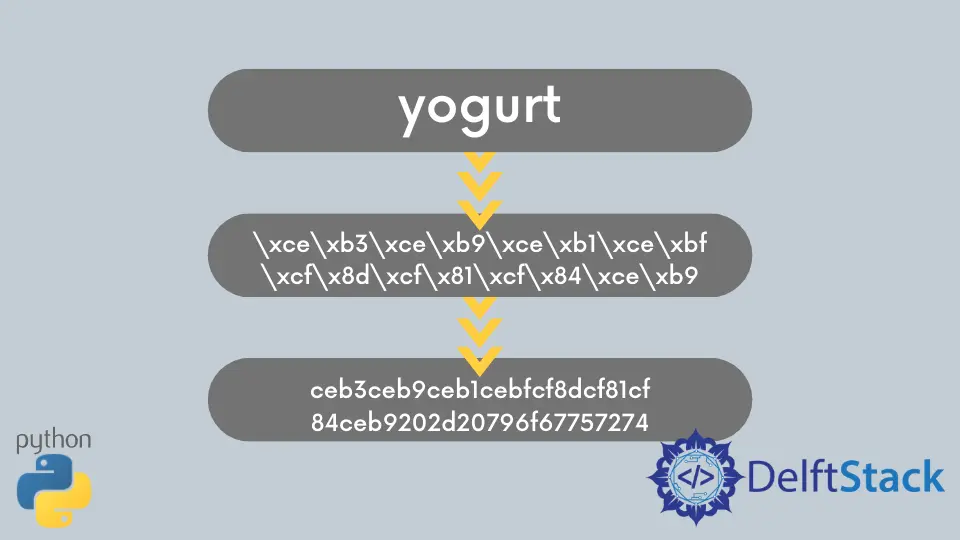
Bytes are a fundamental data type in Python, representing a sequence of bytes that can be stored on a disk as a variable. They are used for encoding and decoding purposes and are declared similarly to strings but prefixed with the character b
.
Bytes can accommodate special Unicode characters prefixed with \x
. In this tutorial, we will explore how to convert bytes into hexadecimal format in Python.
Initializing a Byte Literal in Python
Before delving into the conversion process, it’s crucial to understand the concept of bytes in Python. Bytes are a fundamental data type used to represent binary data, and they can store a sequence of bytes, each ranging from 0
to 255
.
They are particularly valuable when dealing with non-textual data, such as images, sound, or binary files.
Let’s start by understanding how to initialize a byte literal in Python. We’ll declare a string containing special characters and use the encode('utf-8')
method to transform it into a byte literal.
byte_var = "γιαούρτι - yogurt".encode("utf-8")
print(byte_var)
The output of this code will be:
b'\xce\xb3\xce\xb9\xce\xb1\xce\xbf\xcf\x8d\xcf\x81\xcf\x84\xce\xb9 - yogurt'
In this output, you can observe that the encode()
method converts the string into a byte literal, prefixed with the character b
, and special characters are transformed into their corresponding Unicode symbols.
This step is crucial when dealing with binary data or data containing characters outside the standard ASCII range.
Use the hex()
Method to Convert a Byte to Hex in Python
The hex()
function is a straightforward and convenient method for converting a byte object into a hexadecimal string.
When applied to a byte object, this involves a two-step process:
-
First, you need to convert the byte object into an integer. This is necessary because
hex()
takes an integer as input, not a byte object.You can achieve this using the
int.from_bytes()
method, specifying the byte order (usually'big'
for most use cases). -
After obtaining the integer representation, you apply the
hex()
function to this integer, resulting in the hexadecimal string.
Here’s an example using the given byte_var
:
byte_var = "γιαούρτι - yogurt".encode("utf-8")
# Converting to an integer and then to a hexadecimal string
hex_representation = hex(int.from_bytes(byte_var, byteorder="big"))
print(hex_representation)
In this code, we start with a byte object, byte_var
, which is obtained by encoding the string "γιαούρτι - yogurt"
using the UTF-8 encoding.
Next, we convert the byte object into an integer using the int.from_bytes()
method, specifying 'big'
as the byte order. We then apply the hex()
function to the integer to obtain the hexadecimal string.
Output:
0xceb3ceb9ceb1cebfcf8dcf81cf84ceb9202d20796f67757274
The result is a hexadecimal string prefixed with '0x'
, following the common Python convention for hexadecimal representations.
Using the hex()
function for byte-to-hex conversion offers several advantages.
- The
hex()
method is concise and easy to use, simplifying the process of converting binary data into a human-readable hexadecimal format. - The resulting hexadecimal string retains the
'0x'
prefix, making it instantly recognizable as a hexadecimal value. hex()
consistently produces the same output format, simplifying data processing and comparisons.- The
hex()
function is available in all Python versions, making it a reliable choice for code that needs to work across different Python versions.
Use the binascii
Module to Convert a Byte to Hex in Python
The binascii
module is a Python standard library module that provides various functions for working with binary data, including encoding and decoding operations. One of the key functions provided by the binascii
module is hexlify()
, which is used to convert binary data to a hexadecimal representation.
The hexlify()
function is used to convert binary data (in the form of bytes) into a hexadecimal representation. It works by taking a bytes-like object (e.g., a bytes object) as input and returning a hexadecimal string.
Here are the crucial steps to use binascii.hexlify()
:
-
First, you need to have your data as a byte-like object.
-
To use
hexlify()
, you need to import thebinascii
module withimport binascii
. -
Use the
binascii.hexlify()
function to convert the byte data into a hexadecimal string. This function ensures that the output is a clean hexadecimal representation without any unwanted characters. -
The result of
hexlify()
is a bytes object containing hexadecimal characters. If you need the result as a string (which is often more human-readable), you can use thedecode()
method to convert it.
Let’s see an example:
import binascii
byte_var = "γιαούρτι - yogurt".encode("utf-8")
hex_representation = binascii.hexlify(byte_var)
print(hex_representation)
In this code, we start with a byte object, byte_var
, which is obtained by encoding the string "γιαούρτι - yogurt"
using the UTF-8 encoding.
Then, we import the binascii
module to access the hexlify()
function. Finally, we use binascii.hexlify()
to convert the byte data into a hexadecimal representation.
Output:
b'ceb3ceb9ceb1cebfcf8dcf81cf84ceb9202d20796f67757274'
Note that the return value of hexlify()
is a byte literal, unlike the hex()
method, which returns a converted string. If you wish to obtain a string, you can use the decode()
function as follows:
import binascii
byte_var = "γιαούρτι - yogurt".encode("utf-8")
hex_representation = binascii.hexlify(byte_var).decode()
print(hex_representation)
The output will be:
ceb3ceb9ceb1cebfcf8dcf81cf84ceb9202d20796f67757274
Using the binascii
module for byte-to-hex conversion offers several advantages:
- The
binascii
module is a robust and reliable way to perform binary-to-hexadecimal conversions. It handles the conversion process accurately and is well-suited for a wide range of use cases. - The
hexlify()
method consistently produces clean hexadecimal output, which is important for data processing and comparisons. - This method is available in all Python versions, making it a dependable choice for cross-version compatibility.
- The
binascii
module provides various functions for different encoding and decoding operations, making it a versatile tool for binary data manipulation.
Use the codecs
Module to Convert a Byte to Hex in Python
The codecs
module in Python is primarily designed for encoding and decoding text data. It provides a versatile set of encoding and decoding operations for various character encodings.
While it’s commonly used for working with text data, it can also be applied to binary data for specific encoding tasks.
In this context, we use the codecs
module to perform byte-to-hex conversion using the codecs.encode()
function. This function can be used to encode data from a given bytes-like object (e.g., a byte object) into a specified encoding.
In our case, we’re using the hex
encoding to convert the byte data into its hexadecimal representation.
Here’s how this function is used for byte-to-hex conversion:
-
First, you need to have your data in the form of a bytes-like object, such as a bytes object.
-
To use
codecs.encode()
, you must import thecodecs
module withimport codecs
. -
Use the
codecs.encode()
function to convert the byte data into a hexadecimal representation. Specifyhex
as the encoding to be used. -
The result of
codecs.encode()
is a bytes object containing hexadecimal characters. If you need the result as a string (which is often more human-readable), you can use thedecode()
method to convert it.
Let’s see how to use the codecs
module for this purpose:
import codecs
byte_var = "γιαούρτι - yogurt".encode("utf-8")
hex_representation = codecs.encode(byte_var, "hex").decode()
print(hex_representation)
In this code:
- We start with a byte object,
byte_var
, which is obtained by encoding the string"γιαούρτι - yogurt"
using the UTF-8 encoding. - We import the
codecs
module to access theencode()
function. - We use
codecs.encode()
to convert the byte data into a hexadecimal representation using the"hex"
encoding. - The
decode()
method is applied to the result to obtain the hexadecimal string.
Output:
ceb3ceb9ceb1cebfcf8dcf81cf84ceb9202d20796f67757274
The resulting output is a hexadecimal string that represents the byte data. The codecs
module ensures that the output is a clean hexadecimal representation suitable for various applications, such as data encoding and transmission.
Using the codecs
module for byte-to-hex conversion has its advantages:
- The
codecs
module provides a wide range of encoding and decoding options for text data. While it’s primarily designed for text encodings, you can leverage it for other encoding purposes, such as byte-to-hex conversion. - The
"hex"
encoding provided by thecodecs
module consistently produces clean hexadecimal output, ensuring that the output is suitable for data processing and comparisons. - The
codecs
module is available in all Python versions, making it a dependable choice for code that needs to work across different Python versions. - Using the
codecs
module is straightforward and allows you to encapsulate encoding operations in a single line of code.
Use the struct
Module to Convert a Byte to Hex in Python
The struct
module in Python is primarily designed for packing and unpacking structured binary data. It allows you to work with binary data in a way that aligns with the data’s structure.
While it’s not the most common choice for byte-to-hex conversion, it can be employed for this purpose. The key to using the struct
module for this task is to convert the byte data into a sequence of integers and then convert those integers into a hexadecimal string.
The struct.unpack()
function is used to unpack binary data into a sequence of values, typically integers. You specify the format of the binary data using a format string, and the function converts the binary data accordingly.
After using struct.unpack()
to convert the byte data into integers, you can convert these integers into a hexadecimal string. This is done using a list comprehension and the join()
function.
The list comprehension iterates through the integers, converts each integer to a hexadecimal string using the f'{x:02x}'
formatting, and then joins these hexadecimal strings together to form the final hexadecimal representation of the byte data.
Let’s see an example:
import struct
byte_var = "γιαούρτι - yogurt".encode("utf-8")
int_list = struct.unpack(f"{len(byte_var)}B", byte_var)
hex_representation = "".join(f"{x:02x}" for x in int_list)
print(hex_representation)
In this code, we start with a byte object, byte_var
, which is obtained by encoding the string "γιαούρτι - yogurt"
using the UTF-8 encoding. We then import the struct
module to access the pack
and unpack
functions.
Then, we use struct.unpack()
to convert the byte data into a sequence of integers. The format string f'{len(byte_var)}B'
specifies the format for unpacking, where 'B'
indicates an unsigned byte.
Lastly, we convert the integers into a hexadecimal string using list comprehension and the join()
function.
Output:
ceb3ceb9ceb1cebfcf8dcf81cf84ceb9202d20796f67757274
The resulting output is a hexadecimal string that represents the byte data. While using the struct
module for byte-to-hex conversion might involve more steps compared to other methods, it provides flexibility and can be handy for more complex encoding and decoding tasks.
Using the struct
module for byte-to-hex conversion has some advantages:
- The
struct
module is versatile and can be used for a wide range of tasks involving binary data. It’s particularly useful when you need to handle structured binary data. - With the
struct
module, you have precise control over how binary data is packed and unpacked, which can be beneficial for complex data structures. - The
struct
module is part of the Python standard library, ensuring that it is available and consistent across different Python versions. - If you’re working with structured binary data, the
struct
module is a powerful tool for handling fields of different types, sizes, and endianness.
Conclusion
Converting bytes to a hexadecimal format in Python is a fundamental operation, often required in various data manipulation and encoding tasks. In this article, we’ve explored multiple methods to perform this conversion, including using the hex()
function, the binascii
module, the codecs
module, and the struct
module.
Each method has its advantages and can be chosen based on specific requirements and the complexity of the task at hand. By understanding and employing these methods, Python developers can efficiently work with binary data and seamlessly convert it into a human-readable hexadecimal format.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python Bytes
- How to Convert Bytes to Int in Python 2.7 and 3.x
- How to Convert Int to Bytes in Python 2 and Python 3
- How to Convert Int to Binary in Python
- How to Convert Bytes to String in Python 2 and Python 3
- How to Convert String to Bytes in Python
- B in Front of String in Python