How to Check if a Variable Exists in Python
- Method 1: Using the locals() Function
- Method 2: Using the globals() Function
- Method 3: Using Try-Except Block
- Method 4: Using the in Keyword with a Dictionary
- Conclusion
- FAQ
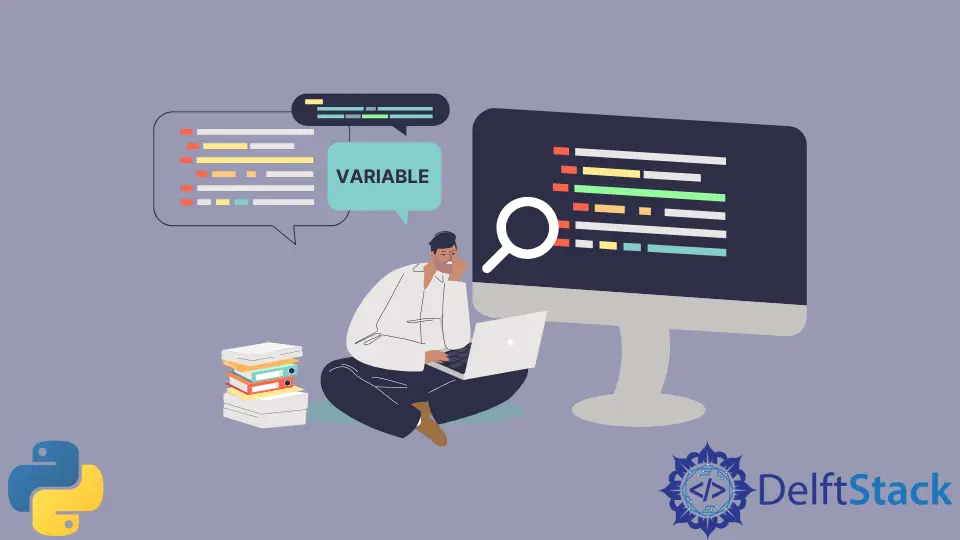
In the world of programming, managing variables is a fundamental skill. Sometimes you might find yourself in a situation where you need to check if a variable exists before performing operations on it. In Python, there are several ways to accomplish this, using built-in functions like locals()
, globals()
, and the in
keyword. Understanding these methods can help you avoid errors and write cleaner, more efficient code.
In this article, we will explore how to check if a variable exists in Python, complete with code examples and detailed explanations. Whether you’re a beginner or an experienced developer, this guide will enhance your Python programming skills.
Method 1: Using the locals() Function
The locals()
function in Python returns a dictionary representing the current local symbol table. This means it contains all the local variables in the current scope. By checking if a variable name exists in this dictionary, you can determine if the variable has been defined.
Here’s how you can use locals()
to check for a variable:
x = 10
if 'x' in locals():
print("Variable 'x' exists.")
else:
print("Variable 'x' does not exist.")
Output:
Variable 'x' exists.
In this example, we first define a variable x
with a value of 10. The if 'x' in locals():
statement checks if the string ‘x’ is a key in the dictionary returned by locals()
. If it is, the code prints that the variable exists; otherwise, it states that it does not. This method is particularly useful when you’re working within a function or a local scope, as it allows you to verify the existence of variables without raising a NameError
.
Method 2: Using the globals() Function
Similar to locals()
, the globals()
function returns a dictionary representing the global symbol table. This is useful when you want to check for variables that are defined in the global scope.
Here’s an example of how to use globals()
:
y = 20
if 'y' in globals():
print("Variable 'y' exists.")
else:
print("Variable 'y' does not exist.")
Output:
Variable 'y' exists.
In this snippet, we define a global variable y
with a value of 20. The if 'y' in globals():
line checks if ‘y’ is present in the global variables. If it is, it confirms the existence of the variable. This method is particularly beneficial when working with modules or scripts where you need to ensure that certain global variables are available before accessing them.
Method 3: Using Try-Except Block
Another effective way to check for the existence of a variable is by using a try-except block. This approach is straightforward and allows you to handle the situation gracefully if the variable is not defined.
Here’s how it works:
try:
z
print("Variable 'z' exists.")
except NameError:
print("Variable 'z' does not exist.")
Output:
Variable 'z' does not exist.
In this case, we attempt to access the variable z
directly. If z
is not defined, a NameError
is raised, and the code in the except block executes, indicating that the variable does not exist. This method is particularly useful when you are unsure whether a variable has been defined and want to avoid cluttering your code with additional checks.
Method 4: Using the in Keyword with a Dictionary
If you’re working with a dictionary that holds your variables, you can easily check for the existence of a variable using the in
keyword. This method is particularly useful when you have a dynamic set of variables stored in a dictionary.
For example:
variables = {'a': 1, 'b': 2}
if 'a' in variables:
print("Variable 'a' exists.")
else:
print("Variable 'a' does not exist.")
Output:
Variable 'a' exists.
In this example, we have a dictionary named variables
that contains some key-value pairs. The if 'a' in variables:
line checks if ‘a’ is a key in the dictionary. If it is, it confirms the existence of the variable. This method is particularly effective for managing multiple variables in a structured way, making your code cleaner and more organized.
Conclusion
Checking if a variable exists in Python is a crucial skill that can save you from potential errors and enhance your coding efficiency. By utilizing functions like locals()
and globals()
, or employing try-except blocks and dictionary checks, you can ensure that your code runs smoothly. Each method has its advantages, so choose the one that best fits your coding style and project requirements. With these techniques in your toolkit, you’ll be better prepared to handle variables in your Python programs.
FAQ
-
How can I check for a variable in a function scope?
You can use the locals() function to check if a variable exists in a function’s local scope. -
What happens if I try to access a variable that doesn’t exist?
Accessing a non-existent variable will raise a NameError in Python. -
Can I use globals() in a function?
Yes, you can use globals() within a function to access global variables. -
Is using try-except the best way to check for a variable’s existence?
It can be effective, especially when you are unsure about the variable’s existence, but it may not be the most efficient method for all situations. -
How do I check if a variable exists in a dictionary?
You can use the in keyword to check if a key exists in the dictionary.
Related Article - Python Exception
- How to Open File Exception Handling in Python
- How to Mock Raise Exception in Python
- How to Rethrow Exception in Python
- How to Raise Exception in Python
- How to Handle NameError Exception in Python
- Python Except Exception as E