Python Except Exception as E
-
Python
except Exception as e
-
Understanding the
except
Statement -
Understanding
except Exception as e
Statement -
Code Example of
except Exception as e
- Conclusion
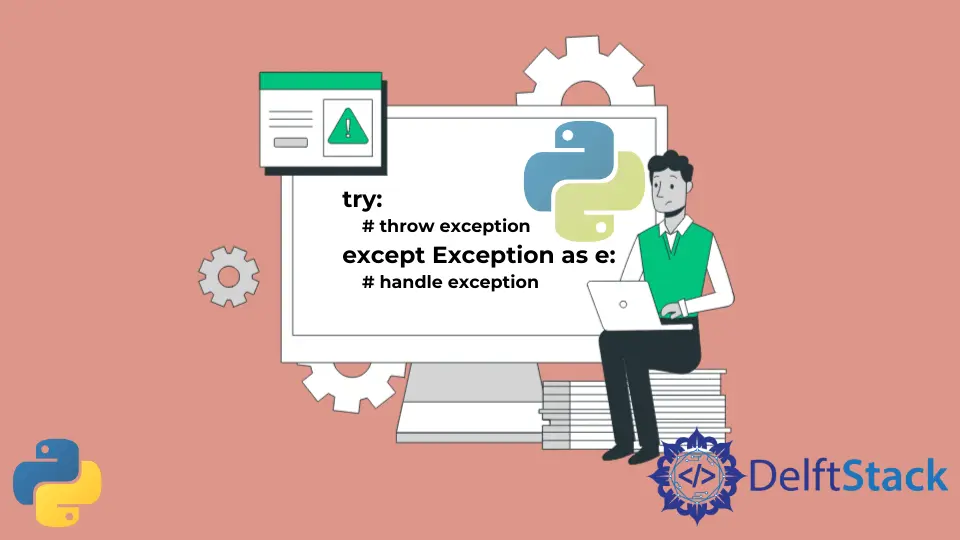
Exceptions are mainly the outcome of any given program being logically correct while still giving an error on the execution of the code.
In most cases, however, this error cannot alter the execution of the program. It rather alters or changes the default flow and functioning of the program.
In Python, the except
clause is used in conjunction with a try-except
block to handle exceptions. When an error occurs within the try
block, the code within the associated except
block is executed, allowing the program to gracefully respond to and manage the exception.
Python except Exception as e
We can define an exception
as an event that, when raised, can alter the flow of the program.
Exceptions are mainly the outcome of any given program being logically correct while still giving an error on the execution of the code. In most cases, however, this error cannot alter the execution of the program. It rather alters or changes the default flow and functioning of the program.
Python provides a powerful construct called try-except
for managing exceptions gracefully. Among the various ways to catch and handle exceptions, one widely-used and versatile approach is except Exception as e
. This construct allows developers to capture detailed information about the exception, facilitating effective debugging and error resolution.
Understanding the except
Statement
In Python, the try
and except
blocks provide a straightforward mechanism for handling exceptions and preventing program crashes. The try
block encloses the code that may raise an exception, while the except
block contains the instructions to execute if an exception occurs.
The syntax for the simple except
statement is:
try:
# write code that may throw exception
except:
# the code for handling the exception
The simple except
statement is utilized in general cases, and it accepts all the exceptions.
Understanding except Exception as e
Statement
The except Exception as e
statement in Python is used to catch and handle exceptions. It is a more specific form of exception handling that allows you to capture an exception object for further analysis or logging.
Using except Exception as e
allows you to catch a broad range of exceptions and provides you with the flexibility to inspect and manage those exceptions in a more detailed manner.
The syntax for the except Exception as e
statement is:
try:
# write code that may throw exception
except Exception as e:
# the code for handling the exception
Both except
and except Exception as e
statements are utilized to implement exception handling. However, these two statements do have a significant difference between them, not just the syntax.
e
is used to create the given Exception
in the code and shows to the user all of the attributes of the given Exception
object.
While the except Exception as e
statement is much more in-depth, it does not deliver on catching exceptions like BaseException
or some of the system-exiting exceptions like KeyboardInterrupt
and SystemExit
. However, a simple except
statement can fulfill this task and catch all these exceptions.
Code Example of except Exception as e
Let’s dive into a practical example to understand the except Exception as e
construct in action. Consider the following code snippet:
def divide_numbers(x, y):
try:
result = x / y
print("Division successful!")
except Exception as e:
print(f"Error: {e}")
print("Exception handled.")
finally:
print("Execution completed.")
# Example usage
divide_numbers(10, 2)
divide_numbers(10, 0)
In this example, we define a function divide_numbers
that attempts to perform a division operation. The try
block encapsulates the potentially problematic code, such as division by zero.
The except Exception as e
block catches any exceptions that may arise during the execution of the try
block.
The finally
block contains code that is always executed, whether an exception occurs or not. It’s useful for performing cleanup operations.
Output:
Division successful!
Execution completed.
Error: division by zero
Exception handled.
Execution completed.
We run the example with two function calls, divide_numbers(10, 2)
and divide_numbers(10, 0)
. The one that is divided by zero is handled by the exception and prints the specific exception error, then proceeds with the finally
block.
Conclusion
The except Exception as e
construct is a powerful tool for handling exceptions in Python. This method allows developers to handle errors with grace, gather useful information about exceptions, and take the necessary steps to guarantee the stability of their programs.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn