How to Mock Raise Exception in Python
- What Is Mocking?
-
How to Python
Mock
Raise Exception Using theside_effect
Parameter -
How to Python Mock Raise Exception Using
MagicMock
With the__call__
Method -
How to Python Mock Raise Exception Using the
unittest.mock.patch
Decorator - Conclusion
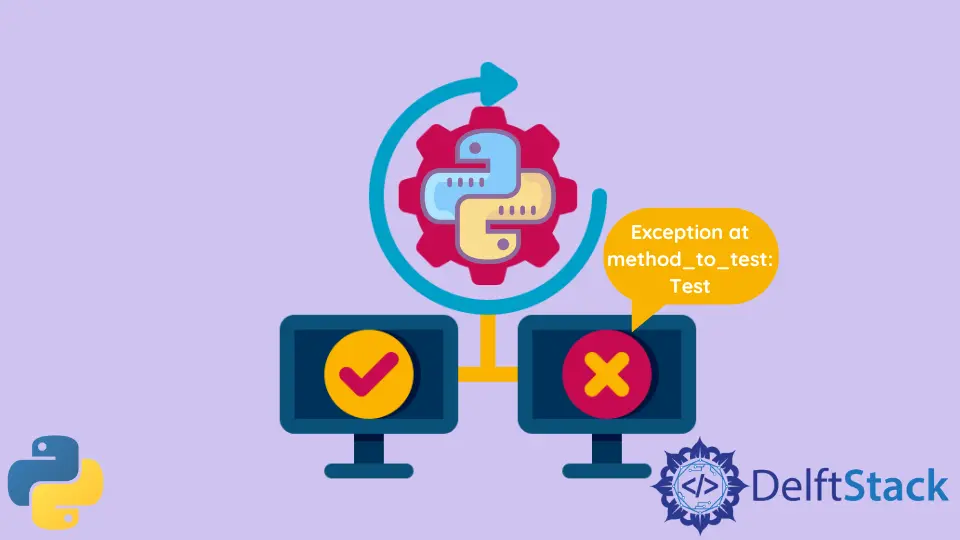
Testing is a significant phase in the software development lifecycle, ensuring the reliability and resilience of code under various conditions. In Python, the ability to simulate exceptions during testing is vital for evaluating the effectiveness of error-handling mechanisms.
This article explores three powerful techniques for achieving this: using the unittest.mock
module’s side_effect
parameter, leveraging the MagicMock
class with the __call__
method, and employing the @patch
decorator. Each method offers a unique approach to creating controlled testing environments, allowing developers to scrutinize how their code responds to specific exception scenarios.
What Is Mocking?
Mocking, in the context of software development, is a versatile and indispensable technique employed to emulate the behavior of real objects or components. The primary objective is to create surrogate entities known as mocks, which replicate the functionalities of actual components within the codebase.
This practice holds particular significance when testing, as it facilitates the isolation of components, the simulation of scenarios, and the establishment of controlled environments for thorough testing.
The fundamental concept behind mocking lies in its ability to mimic the behavior of a real object without executing its actual functionality. This is achieved through the creation of mock instances or objects, instances of the unittest.mock.Mock
class in Python.
These mock objects act as stand-ins, responding to method calls and attribute accesses just like their genuine counterparts.
Understanding the essence of mocking becomes crucial when dealing with components that have external dependencies or interactions. By introducing mocks, developers gain the flexibility to evaluate the behavior of their code in isolation without the influence of external factors.
In Python, the unittest.mock
module, introduced in Python 3.3, serves as a cornerstone for implementing mocking techniques. It provides essential tools, including the Mock
class and the patch
decorator, simplifying the process of creating mocks and ensuring the seamless integration of these surrogate objects into the testing workflow.
Mocking proves to be instrumental in enhancing the reliability and effectiveness of testing processes. It enables developers to control and manipulate the behavior of dependencies, simulate diverse scenarios, and meticulously assess the performance of their code under various conditions.
How to Python Mock
Raise Exception Using the side_effect
Parameter
Before diving into the side_effect
parameter, let’s briefly review the basics of mocking in Python. The unittest.mock
module provides the Mock
class, which allows us to create a mock object with specified behaviors for function or method calls
These mock objects can replace real objects during testing, enabling us to control their responses and interactions.
from unittest.mock import Mock
# Creating a simple mock object
my_mock = Mock()
The side_effect
parameter of the Mock
class is a powerful tool for customizing the behavior of a mock object.
It allows you to specify a callable (such as a function or a class) that will be called when the mock is called. This is particularly useful for simulating exceptions during testing.
Now, let’s apply the side_effect
parameter to test exception handling in a Python function.
Consider the divide
function, which performs division but raises a ValueError
when attempting to divide by zero. Our goal is to test how the code handles this specific exception scenario using the side_effect
parameter.
# File: calculator.py
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Now, let’s create a test script using the unittest
module and the @patch
decorator with the side_effect
parameter to mock the divide
function and force it to raise a ValueError
.
# File: test_calculator.py
import unittest
from unittest.mock import patch
from calculator import divide
class TestCalculator(unittest.TestCase):
# Use the patch decorator to mock the divide function and raise a ValueError
@patch("calculator.divide", side_effect=ValueError("Cannot divide by zero"))
def test_divide_by_zero(self, mock_divide):
# Test the scenario where divide function raises a ValueError
# Use a context manager to catch the expected ValueError
with self.assertRaises(ValueError) as context:
# Call the divide function with arguments that cause division by zero
result = divide(10, 0)
# Assert that the caught exception has the expected error message
self.assertEqual(str(context.exception), "Cannot divide by zero")
if __name__ == "__main__":
# Running the unit tests
unittest.main()
Python Mock
Raise Exception Using side_effect
Steps
Let’s break down the Python program to understand it better:
Step 1: Importing Modules
We start by importing the necessary modules - unittest
for creating test cases and patch
from unittest.mock
for creating mock objects. Additionally, we import the divide
function from calculator.py
.
import unittest
from unittest.mock import patch
from calculator import divide
Step 2: Creating the Test Class
We create a test class, TestCalculator
, which inherits from unittest.TestCase
. This class will contain our test methods.
class TestCalculator(unittest.TestCase):
Step 3: Using @patch
Decorator
We use the @patch
decorator to temporarily replace the divide
function with a mock version. The side_effect
parameter is set to ValueError("Cannot divide by zero")
, indicating that calling the mock function should raise a ValueError
with the specified message.
@patch("calculator.divide", side_effect=ValueError("Cannot divide by zero"))
Step 4: Defining the Test Method
We define a test method, test_divide_by_zero
, which takes mock_divide
as a parameter to configure the behavior of the mock instance. Inside the method, we use self.assertRaises
to ensure that calling divide(10, 0)
results in a ValueError
.
We also check if the correct error message is raised.
def test_divide_by_zero(self, mock_divide):
with self.assertRaises(ValueError) as context:
result = divide(10, 0)
self.assertEqual(str(context.exception), "Cannot divide by zero")
Step 5: Running the Test
Finally, we run the test script using unittest.main()
.
if __name__ == "__main__":
unittest.main()
Code Output:
Upon running the test script, the output should indicate a successful test:
This demonstrates effective exception handling of the scenario in the divide
function using the side_effect
parameter.
How to Python Mock Raise Exception Using MagicMock
With the __call__
Method
The unittest.mock
module provides the MagicMock
class, which is an extension of the Mock
class. MagicMock
includes several magic methods, such as __call__
, which allows instances of the class to be called as functions.
Leveraging this method, we can customize the behavior of the mock when it’s called, including raising exceptions.
Let’s consider a Python function, divide
, which normally performs division but raises a ValueError
when attempting to divide by zero. Our objective is to explore the use of MagicMock
with the __call__
method to mock the divide
function and force it to raise an exception during testing.
# File: calculator.py
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Now, let’s create a test function using the unittest
module, the MagicMock
class, and the __call__
method to mock the divide
function and make it raise a ValueError
.
# File: test_calculator.py
import unittest
from unittest.mock import patch, MagicMock
from calculator import divide
class TestCalculator(unittest.TestCase):
def test_divide_exception(self):
# Create a Magic Mock object to mock the divide function
mock_divide = MagicMock()
# Set the side_effect of the MagicMock to raise a ValueError
mock_divide.side_effect = ValueError("Cannot divide by zero")
# Replace the actual divide function with the mock instance in the test scope
with patch("calculator.divide", mock_divide):
# Call the mocked divide function, which will now raise the specified exception
with self.assertRaises(ValueError):
result = divide(10, 0)
# Check the exception message separately
self.assertEqual(str(mock_divide.side_effect), "Cannot divide by zero")
if __name__ == "__main__":
unittest.main()
Python Mock Raise Exception Using MagicMock
With __call__
Steps
Let’s break down the example code above to understand it better:
Step 1: Importing Modules
We begin by importing the necessary modules - unittest
for creating test cases, MagicMock
from unittest.mock
for creating mock objects, and the divide function
from calculator.py
.
import unittest
from unittest.mock import MagicMock
from calculator import divide
Step 2: Creating the Test Class
Similar to the previous example, we create a test class, TestCalculator
, which inherits from unittest.TestCase
.
class TestCalculator(unittest.TestCase):
Step 3: Defining the Test Method
We define a test method, test_divide_exception
. Inside this method, we create a MagicMock
object named mock_divide
to simulate the behavior of the divide
function.
def test_divide_exception(self):
# Create a MagicMock object to mock the divide function
mock_divide = MagicMock()
Step 4: Setting side_effect
for MagicMock
We set the side_effect
of the mock instance mock_divide
to raise a ValueError
with the message "Cannot divide by zero"
when the mock is called.
This allows us to simulate scenarios where the original function raises specific exceptions during execution. It is essential to match the exception class to the one expected in the test.
mock_divide.side_effect = ValueError("Cannot divide by zero")
Step 5: Replacing the Actual Function
Within the test scope, we replace the actual divide
function with our mock_divide
using the patch
decorator.
with patch("calculator.divide", mock_divide):
Step 6: Calling the Mocked Function
We then call the mocked function divide
within the test block. This call will now raise the specified ValueError
.
with self.assertRaises(ValueError):
result = divide(10, 0)
self.assertEqual(str(mock_divide.side_effect), "Cannot divide by zero")
Code Output:
Upon running the test script, the output should indicate a successful test:
This output confirms that the divide
function correctly raises an exception with the specified error message when attempting to divide by zero. Using MagicMock
with the __call__
method provides a clean and effective way to simulate exceptions during testing, enhancing the reliability of your code.
How to Python Mock Raise Exception Using the unittest.mock.patch
Decorator
The unittest.mock.patch
decorator provides a convenient way to temporarily replace objects or functions during testing. This is particularly useful for isolating the code under test from external dependencies or simulating different scenarios, such as raising exceptions.
The decorator can be applied to functions, methods, or even entire classes, allowing you to control their behavior during testing.
Consider the same Python function, divide
, which performs division but raises a ValueError
when attempting to divide by zero. This time, we will use the patch
decorator to mock the divide
function and make it raise a ValueError
during testing.
# File: calculator.py
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Now, let’s create an example code using the unittest
module and the patch
decorator to simulate the exception scenario.
# File: test_calculator.py
import unittest
from unittest.mock import patch
from calculator import divide
class TestCalculator(unittest.TestCase):
# Use the patch decorator to mock the divide function
@patch("calculator.divide", side_effect=ValueError("Cannot divide by zero"))
def test_divide_by_zero(self, mock_divide):
# Test the scenario where divide function raises a ValueError
# Use a context manager to catch the expected ValueError
with self.assertRaises(ValueError) as context:
# Call the divide function with arguments that cause division by zero
result = divide(10, 0)
# Assert that the caught exception has the expected error message
self.assertEqual(str(context.exception), "Cannot divide by zero")
if __name__ == "__main__":
unittest.main()
Python Mock Raise Exception Using unittest.mock.patch
Steps
Let’s break down the example code above to understand the usage of the unittest
module and the patch
decorator to simulate the error scenario.
Step 1: Importing Modules
Begin by importing the necessary modules - unittest
for creating test cases, and patch
from unittest.mock
for using the patch
decorator. Additionally, import the divide
function from calculator.py
.
import unittest
from unittest.mock import patch
from calculator import divide
Step 2: Creating the Test Class
Create a test class, TestCalculator
, which inherits from unittest.TestCase
. This class will contain our test methods.
class TestCalculator(unittest.TestCase):
Step 3: Using @patch
Decorator
Apply the @patch
decorator to the test method, specifying the target function to be patched (calculator.divide
) and setting the side_effect
to raise a ValueError
with the desired message.
@patch("calculator.divide", side_effect=ValueError("Cannot divide by zero"))
Step 4: Defining the Test Method
Define a test method, test_divide_by_zero
, which takes mock_divide
as a parameter. Inside this method, use self.assertRaises
to ensure that calling divide(10, 0)
results in a ValueError
.
Additionally, check if the correct error message is raised.
def test_divide_by_zero(self, mock_divide):
with self.assertRaises(ValueError) as context:
result = divide(10, 0)
self.assertEqual(str(context.exception), "Cannot divide by zero")
Step 5: Running the Test
Run the test script using unittest.main()
.
if __name__ == "__main__":
unittest.main()
Code Output:
After running the example code, the output should indicate a successful test:
This output confirms that the divide
function correctly raises a ValueError
with the specified error message when attempting to divide by zero.
The unittest.mock.patch
function acts as a context manager, temporarily replacing the original function with the mock instance. Inside the context manager, we can set the desired behavior, such as raising exceptions, to ensure our test cases cover various error scenarios.
This precise control is valuable for testing edge cases and error or exception handling in our code.
Conclusion
Mocking functions and raising exceptions during testing is an essential technique for ensuring that your code behaves as expected in various scenarios. The methods explored in this article, including the side_effect
parameter, the MagicMock
class with __call__
, and the @patch
decorator, provide developers with versatile tools to create controlled testing environments.
Whether it’s raising exceptions with specific error messages or mimicking unexpected scenarios, these techniques contribute to comprehensive testing practices. By incorporating these methods into testing workflows, we can bolster the reliability and resilience of our Python applications, ensuring they stand strong in the face of unforeseen challenges.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Exception
- How to Open File Exception Handling in Python
- How to Rethrow Exception in Python
- How to Raise Exception in Python
- How to Handle NameError Exception in Python
- Python Except Exception as E