Python Unittest Discovery
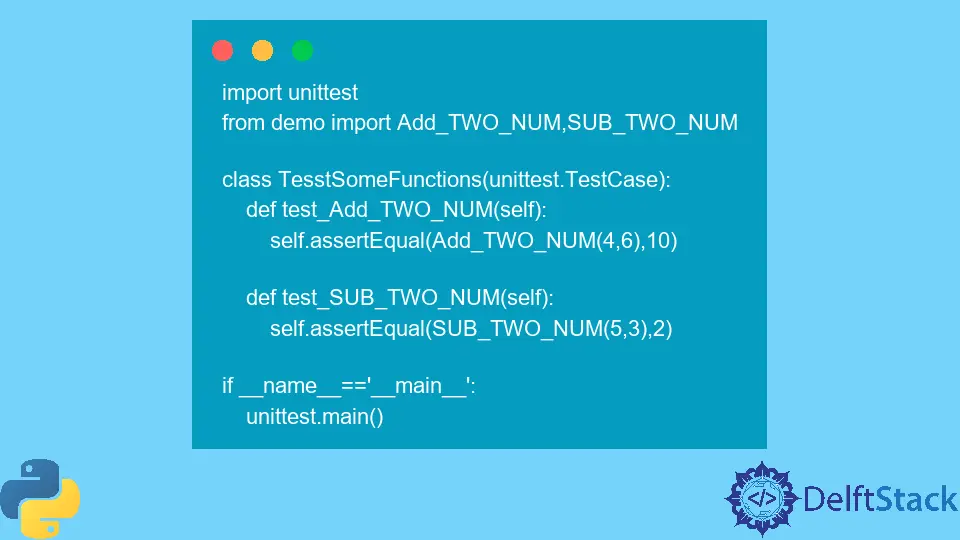
This article will teach us about the unit test and how to run it for a particular piece of code. We will learn the usage of the discover
command to recognize the module name automatically and why it may not work in Python.
Use the unittest
Module to Recognize the Test Module With the Help of the discover
Command in Python
We cannot touch, smell, or feel the software to ascertain its quality, but we can test it using a testing tool. Unit test is the method that instantiates a small portion of your application and verifies its behavior independently from other parts.
In other words, you take a small part of your code and test it in a small function separately. This part of development often gets skipped, leading to the customer testing of your software is a poor user experience.
A typical unit test has three phases: Arrange, Act, and Assert.
Arrange creates proper conditions for the unit test to run or instancing an object. Act gives that object some input or data, and Assert checks whether the output data was as expected.
Let’s create a new file called demo.py
and start writing a code; we will create two functions that add and subtract two numbers. These functions can only accept integers or float as an argument; otherwise, it will throw a TypeError.
def Add_TWO_NUM(a, b):
return a + b
def SUB_TWO_NUM(a, b):
return a - b
Now, we will create a test_functions.py
file for the test using unittest
because unittest
comes inbuilt with Python distribution. We do not need to do anything to install it; however, we can import unittest
in our code.
We have added this extra prefix here, test_
, in front of the name of our file, which is functions.py
. This test_
prefix will help unittest
to recognize that this is the file in which the unit testing functions are written.
At first, as you can see, we import the module unittest
and then import the function from the modules we want to test, and then we initialize a class called TesstSomeFunctions
. If you need to write a test class, you should write a test class before writing all test functions.
Since we are testing, that is why in the parenthesis, we need to give it unittest.TestCase
; the user-defined class should be derived from unittest.TestCase
. To run a test is the only way for functions to be invoked by the unittest
; it is a case of unit tests, and every function in this class will be a single unit test.
We can define this as any other function in our Python script and write the def
keyword. Then start the name with the test prefix like test_
, and then whatever name we can give it.
The unittest
module provides us with assertion. You can see all of the assets in the unittest
module using unittest.
or all the methods on the official documentation.
Using the assertEqual()
method, we will check whether this function is returning 10 or not. Assertion indicates you want to assert that the function returns the desired result as we expect the result 10.
We provide 4 and 6 as an argument to this Add_TWO_NUM()
function. In the second argument of the assertEqual()
method, we provide a check whether it is equal or not.
We will also test another function called test_SUB_TWO_NUM()
, the process would be the same, but in this function, we are testing a function that subtracts two numbers. Now we need to go down to the file and write a line if __name__=='__main__'
and run the unit testing using the command unittest.main()
.
import unittest
from demo import Add_TWO_NUM, SUB_TWO_NUM
class TesstSomeFunctions(unittest.TestCase):
def test_Add_TWO_NUM(self):
self.assertEqual(Add_TWO_NUM(4, 6), 10)
def test_SUB_TWO_NUM(self):
self.assertEqual(SUB_TWO_NUM(5, 3), 2)
if __name__ == "__main__":
unittest.main()
The two test cases are now ready, so let’s run the Python file. As you can see, it gave us information about the two tests that were run in 0.0 seconds.
These two dots represent the tests passed. You would see a capital F
if the test did not pass.
..
----------------------------------------------------------------------
Ran 2 tests in 0.001s
OK
What if you do not want to run your test module from the file as a module? Well, you can do that by typing the command in the terminal.
python -m unittest test_functions.py
This command works all the same.
..
----------------------------------------------------------------------
Ran 2 tests in 0.001s
OK
If you want more information, you can put v -
, which means verbose, giving you more details on each unit test. It tells you which test is the best.
python -m unittest -v test_functions.py
Output:
test_Add_TWO_NUM (test_functions.TesstSomeFunctions) ... ok
test_SUB_TWO_NUM (test_functions.TesstSomeFunctions) ... ok
----------------------------------------------------------------------
Ran 2 tests in 0.002s
OK
There is also a third option to run the unit test from the terminal, discover
. This command will automatically discover all of the modules that start with a test, so we do not need to pass the module name individually.
That is why it is essential to name all your modules starting from test
.
python -m unittest discover
Output:
..
----------------------------------------------------------------------
Ran 2 tests in 0.001s
OK
If the test file name does not start with test
, then the discover
command will return nothing because it recognizes it as a normal Python file or module. For more information about the unittest
module, you can visit python.org.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn