Assert Equal in Python
- What Is an Assert Statement in Python
-
the
assertEquals()
Method in Python -
the
assertEqual()
Method in Python - Conclusion
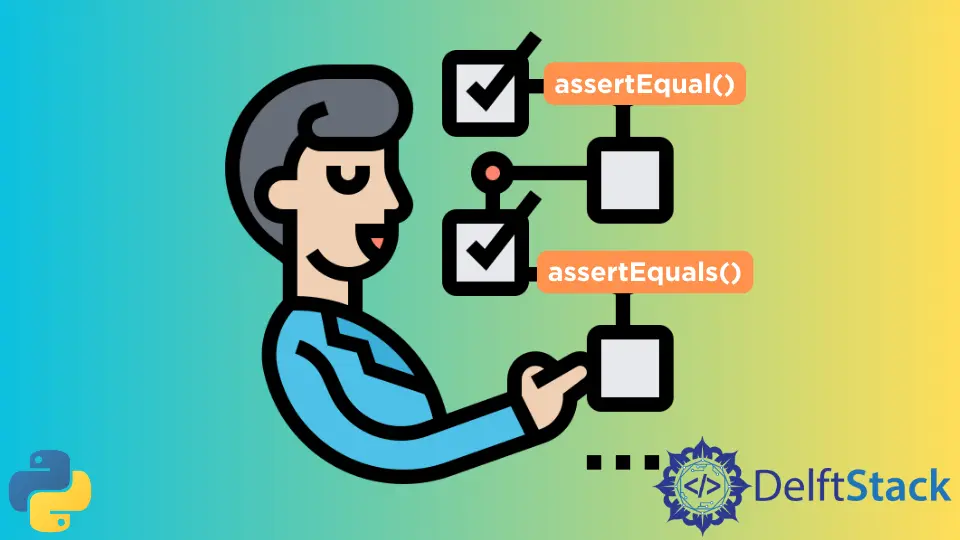
While building software, we need to implement business logic using the code.
To ensure that we implement all the logic and constraints, we use the assert statements in our programs. In big applications, we use unit testing with the help of the assertEquals()
and the assertEqual()
method in Python.
We will discuss how an assert statement
works in Python. We will also see how we can use the assertEquals()
and the assertEqual()
method to implement the business logic and the constraints in Python.
What Is an Assert Statement in Python
In Python, an assert statement checks if an expression is True
or False
. The syntax of the assert statement is as follows.
assert conditional_expression
Here, assert
is the keyword. The conditional_expression
is a conditional statement that evaluates statements as True
or False
.
If the condition_expression
evaluates to True
, the program’s execution advances to the next statement. On the flip side, if the conditional_expression
evaluates to False
, the program raises the AssertionError
exception.
We can see all these below.
num1 = 10
num2 = 5
num3 = 10
print("This statement will get printed")
assert num1 == num3
print(
"This statement will also get printed as the expression in the above assert statement is True."
)
assert num2 == num3
print(
"This statement will not get printed as the expression in the above assert statement is False. This line of code is unreachable."
)
Output:
This statement will get printed
This statement will also get printed as the expression in the above assert statement is True.
Traceback (most recent call last):
File "tempy.py", line 9, in <module>
assert num2 == num3
AssertionError
Here, you can observe that the first print statement is executed automatically.
The statement assert num1 == num3
raises no error as 10==10
evaluates to True
. So, the second print statement is also executed.
After that, the statement "assert num2 == num3"
raises the AssertionError
as 5==10
evaluates to False
. Due to this, the program execution stops, and the third print statement never gets executed.
We can also display a message when the AssertionError
exception occurs. For this, we will use the following syntax.
assert conditional_expression, message
Here, the message
is a string that is printed when the conditional_expression
evaluates to False
and the AssertionError
occurs. We can see this below.
num1 = 10
num2 = 5
num3 = 10
print("This statement will get printed")
assert num1 == num3, "{} is not equal to {}".format(num1, num2)
print(
"This statement will also get printed as the expression in the above assert statement is True."
)
assert num2 == num3, "{} is not equal to {}".format(num2, num3)
print(
"This statement will not get printed as the expression in the above assert statement is False. This line of code is unreachable."
)
Output:
This statement will get printed
This statement will also get printed as the expression in the above assert statement is True.
Traceback (most recent call last):
File "tempy.py", line 9, in <module>
assert num2 == num3, "{} is not equal to {}".format(num2, num3)
AssertionError: 5 is not equal to 10
The output 5 is not equal to 10
is also printed after notifying the AssertionError
. Including these types of messages will help you test the functions of your program more easily as you can notify the requirement using the message whenever the AssertionError
exception occurs.
We can use the assert statement to enforce constraints or implement business logic in Python. However, using the assert statement has a drawback: it stops the program’s execution once the conditional statement in an assert statement
evaluates to False
.
So, in large programs with thousands of constraints and conditions, we will have to execute the program as many times as the AssertionError
exception occurs.
To overcome this, we can use the assertEquals()
or the assertEqual()
statement as discussed ahead.
the assertEquals()
Method in Python
To enforce constraints and business logic in the software, we can use the unittest
module.
The unittest
module provides us with many methods that we can use to enforce constraints. To implement assertions for equality, we can use the assertEquals()
method and the assertEqual()
method.
To implement assertions for equality using the assertEquals()
method, we will first create a class that is a subclass of the TestCase
class defined in the unittest
module. Then, we can define assertions for equality using the following syntax of the assertEquals()
method.
self.assertEquals(self, first, second)
Here, the parameter first
accepts the first value as the input argument. The parameter second
accepts the second value as the input argument.
If the parameter first
is equal to the value in the parameter second
, the unit test will pass successfully. Otherwise, an AssertionError
exception is raised at the current line, and the user is notified about the error.
Hence, the test case fails, but the program’s execution doesn’t stop as it did in the case of the assert
statement. The program runs all the test cases and then notifies the developer of all the errors.
We can see this below.
import unittest
class Tester(unittest.TestCase):
def setUp(self):
self.num1 = 10
self.num2 = 5
self.num3 = 10
def tearDown(self):
print("\nTest case completed. Result:")
def test_condition1(self):
self.assertEquals(self.num1, self.num3)
def test_condition2(self):
self.assertEquals(self.num2, self.num3)
if __name__ == "__main__":
unittest.main()
Output:
/home/aditya1117/PycharmProjects/pythonProject/webscraping.py:14: DeprecationWarning: Please use assertEqual instead.
self.assertEquals(self.num1, self.num3)
.F
======================================================================
FAIL: test_condition2 (__main__.Tester)
----------------------------------------------------------------------
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 17, in test_condition2
self.assertEquals(self.num2, self.num3)
AssertionError: 5 != 10
----------------------------------------------------------------------
Ran 2 tests in 0.001s
FAILED (failures=1)
Test case completed. Result:
Test case completed. Result:
Here, when the unittest.main()
method is executed, an instance of the Tester
class is created. After that, the setUp()
method is executed. The setUp()
method initializes variables and imports values from other modules to the Tester
class.
You can also observe that we have implemented methods test_condition1()
and test_condition2()
. Here, we have included test_
before the name condition1
and condition2
to make the interpreter understand that these methods are being used to enforce the test cases.
If we don’t specify the method name starting with test_
, the method will not get executed by the python interpreter.
The tearDown()
method is executed after every test case. You can use this method to reinitialize variables and other values.
After executing all the test cases, the result shows that a test case has failed. We can also print an optional message each time the assertEquals()
method raises the AssertionError
exception (i.e., the test case fails).
For this, we have to pass the message string as the third input argument to the assertEquals()
method, as shown below.
import unittest
class Tester(unittest.TestCase):
def setUp(self):
self.num1 = 10
self.num2 = 5
self.num3 = 10
def tearDown(self):
print("\nTest case completed. Result:")
def test_condition1(self):
message = "{} is not equal to {}".format(self.num1, self.num3)
self.assertEquals(self.num1, self.num3, message)
def test_condition2(self):
message = "{} is not equal to {}".format(self.num2, self.num3)
self.assertEquals(self.num2, self.num3, message)
if __name__ == "__main__":
unittest.main()
Output:
Test case completed. Result:
Test case completed. Result:
/home/aditya1117/PycharmProjects/pythonProject/webscraping.py:15: DeprecationWarning: Please use assertEqual instead.
self.assertEquals(self.num1, self.num3,message)
.F
======================================================================
FAIL: test_condition2 (__main__.Tester)
----------------------------------------------------------------------
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 19, in test_condition2
self.assertEquals(self.num2, self.num3,message)
AssertionError: 5 != 10 : 5 is not equal to 10
----------------------------------------------------------------------
Ran 2 tests in 0.001s
FAILED (failures=1)
Here, you can observe that the interpreter also prints the message 5 is not equal to 10
when the second test case fails.
The assertEquals()
method was deprecated in 2010. So, while using the assertEquals()
method, you will get a warning that the method has been deprecated with a message DeprecationWarning: Please use assertEqual instead
.
As Python suggests us to use the assertEqual()
method, let us use it to implement assertions for equality in Python.
the assertEqual()
Method in Python
Except for an s
in its name, the working of the assertEqual()
method is entirely similar to the assertEquals()
method. The syntax of both methods is also the same.
Therefore, you can use the assertEqual()
method instead of the assertEquals()
method as follows.
import unittest
class Tester(unittest.TestCase):
def setUp(self):
self.num1 = 10
self.num2 = 5
self.num3 = 10
def tearDown(self):
print("\nTest case completed. Result:")
def test_condition1(self):
self.assertEqual(self.num1, self.num3)
def test_condition2(self):
self.assertEqual(self.num2, self.num3)
if __name__ == "__main__":
unittest.main()
Output:
.F
======================================================================
FAIL: test_condition2 (__main__.Tester)
----------------------------------------------------------------------
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 17, in test_condition2
self.assertEqual(self.num2, self.num3)
AssertionError: 5 != 10
----------------------------------------------------------------------
Ran 2 tests in 0.000s
FAILED (failures=1)
Test case completed. Result:
Test case completed. Result:
Process finished with exit code 1
In the output, we can observe that the program works the same as the previous codes. Also, we haven’t received any warning about the depreciation.
You can add messages to the test cases as follows.
import unittest
class Tester(unittest.TestCase):
def setUp(self):
self.num1 = 10
self.num2 = 5
self.num3 = 10
def tearDown(self):
print("\nTest case completed. Result:")
def test_condition1(self):
message = "{} is not equal to {}".format(self.num1, self.num3)
self.assertEqual(self.num1, self.num3, message)
def test_condition2(self):
message = "{} is not equal to {}".format(self.num2, self.num3)
self.assertEqual(self.num2, self.num3, message)
if __name__ == "__main__":
unittest.main()
Output:
.F
======================================================================
FAIL: test_condition2 (__main__.Tester)
----------------------------------------------------------------------
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 19, in test_condition2
self.assertEqual(self.num2, self.num3, message)
AssertionError: 5 != 10 : 5 is not equal to 10
----------------------------------------------------------------------
Ran 2 tests in 0.001s
FAILED (failures=1)
Test case completed. Result:
Test case completed. Result:
In this article, you can observe that we have implemented a subclass of the TestCase
class defined in the unittest
module to use the assertEquals()
method and the assertEqual()
method.
While developing programs with the Django framework, you might end up implementing a subclass of the TestCase
class defined in the Django.test
module. The program will run into an error in such a case, as shown below.
import unittest
from django.test import TestCase
class Tester(TestCase):
def setUp(self):
self.num1 = 10
self.num2 = 5
self.num3 = 10
def tearDown(self):
print("\nTest case completed. Result:")
def test_condition1(self):
message = "{} is not equal to {}".format(self.num1, self.num3)
self.assertEqual(self.num1, self.num3, message)
def test_condition2(self):
message = "{} is not equal to {}".format(self.num2, self.num3)
self.assertEqual(self.num2, self.num3, message)
if __name__ == "__main__":
unittest.main()
Output:
E
======================================================================
ERROR: setUpClass (__main__.Tester)
----------------------------------------------------------------------
Traceback (most recent call last):
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/test/testcases.py", line 1201, in setUpClass
super().setUpClass()
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/test/testcases.py", line 187, in setUpClass
cls._add_databases_failures()
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/test/testcases.py", line 209, in _add_databases_failures
cls.databases = cls._validate_databases()
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/test/testcases.py", line 195, in _validate_databases
if alias not in connections:
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/utils/connection.py", line 73, in __iter__
return iter(self.settings)
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/utils/functional.py", line 48, in __get__
res = instance.__dict__[self.name] = self.func(instance)
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/utils/connection.py", line 45, in settings
self._settings = self.configure_settings(self._settings)
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/db/utils.py", line 144, in configure_settings
databases = super().configure_settings(databases)
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/utils/connection.py", line 50, in configure_settings
settings = getattr(django_settings, self.settings_name)
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/conf/__init__.py", line 84, in __getattr__
self._setup(name)
File "/home/aditya1117/.local/lib/python3.8/site-packages/django/conf/__init__.py", line 65, in _setup
raise ImproperlyConfigured(
django.core.exceptions.ImproperlyConfigured: Requested setting DATABASES, but settings are not configured. You must either define the environment variable DJANGO_SETTINGS_MODULE or call settings.configure() before accessing settings.
----------------------------------------------------------------------
Ran 0 tests in 0.003s
FAILED (errors=1)
Here, you can observe that the program runs into an error when we use the TestCase
class from the django.test
module. Hence, no test case is executed.
So, make sure that you always use the TestCase
class defined in the unittest module and not in the Django.test
module.
Conclusion
We discussed using the assert statement
, the assertEquals()
, and the assertEqual()
methods for testing our application.
Here, it would help if you remembered that the assert statement
and the assertEqual()
method could not be used in actual applications in the production environment. You can only use these methods to test your application before deploying the code in the production environment.
Also, make sure that you use the assertEqual()
method instead of the assertEquals()
method as the latter has been deprecated from the python programming language.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub