How to Handle NameError Exception in Python
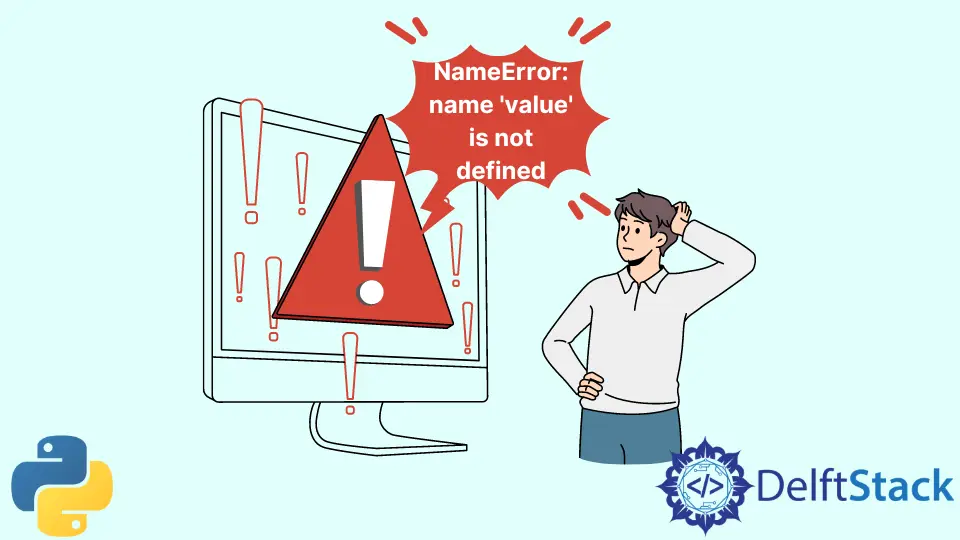
This tutorial will discuss how the NameError
exception occurs and how to handle it in Python.
the NameError
Exception in Python
The NameError
exception in Python is raised when the object called is not initialized in the current scope. It may be defined, but we aren’t using the right name to call it.
There are four distinct reasons for this exception, discussed in detail below.
Misspelled Built-In Function or Keyword
When using the built-in function or keyword, we often misspell it, or the IDE sometimes forgets to correct us. The NameError
exception is raised in this case. To handle this exception, we have to specify the correct name of the function or the keyword being used.
The code snippet below demonstrates a scenario where a built-in function is misspelled while calling, which raises the NameError
exception.
prnt("Hello World")
Output:
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-1-bcdc0d5019dd> in <module>()
----> 1 prnt("Hello World")
NameError: name 'prnt' is not defined
We misspelled the built-in print()
function to raise NameError
exception. The only way to fix this exception is to look up the official documentation of Python for the correct function definition.
Used Undefined Variable or Function
This is another popular cause for raising the NameError
exception. We tend to call a function or a variable that doesn’t exist.
This code snippet demonstrates a scenario where a built-in function is misspelled while calling, which raises the NameError
exception.
print(value)
Output:
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-2-a458d288e6eb> in <module>()
----> 1 print(value)
NameError: name 'value' is not defined
We called the undefined value
attribute to raise the NameError
exception. The only way to fix this exception is to remove the function or variable call from the code.
Called Local Variable or Function in Global Scope
We sometimes call local functions and variables outside their scope, resulting in the NameError
exception.
The following code s demonstrates a scenario where a local variable is being called in the global scope, which raises the NameError
exception.
def func():
value = 6500
print(value)
Output:
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-5-9e4aa38076bc> in <module>()
1 def func():
2 value = 6500
----> 3 print(value)
NameError: name 'value' is not defined
The local variable value
resides inside the func()
function, but we called it in the global scope, which raises the NameError
exception. We can fix this exception by understanding where we can use each variable and function to refer.
Called a Variable or Function Before Declaration
This type of error happens quite often when we call a variable or function before its declaration. Python is an interpreted language, which gets executed line-by-line in a sequence.
The following code demonstrates a variable call before the declaration, raising the NameError
exception.
print(value)
value = 1500
Output:
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-6-49e5676ec0d7> in <module>()
----> 1 print(value)
2 value = 1500
NameError: name 'value' is not defined
Before its declaration, we called the value
variable to raise the NameError
exception. We can fix this exception by writing the calling statement after the initialization.
Handle the NameError
Exception in Python
If we want to handle the NameError
exception so that our code never stops execution, we can enclose our code inside the try/except
block. We can use the NameError
exception class to handle this exception type.
The example code snippet demonstrates how to handle the NameError
exception without stopping the execution with Python’s try/except
block.
try:
print(v)
v = 1500
except NameError:
print("Nameerror exception raised")
Output:
Nameerror exception raised
We handled the NameError
exception by enclosing the error-prone code inside the try
block and writing the error message in the output with the except
block. This method doesn’t solve our problem or remove the exception.
An advantage of this method is that our code never stops its execution.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn