How to Open File Exception Handling in Python
-
Python
open()
File Function - Python Open File Exception
-
Use
try-except
to Handle Exceptions When Reading a File in Python
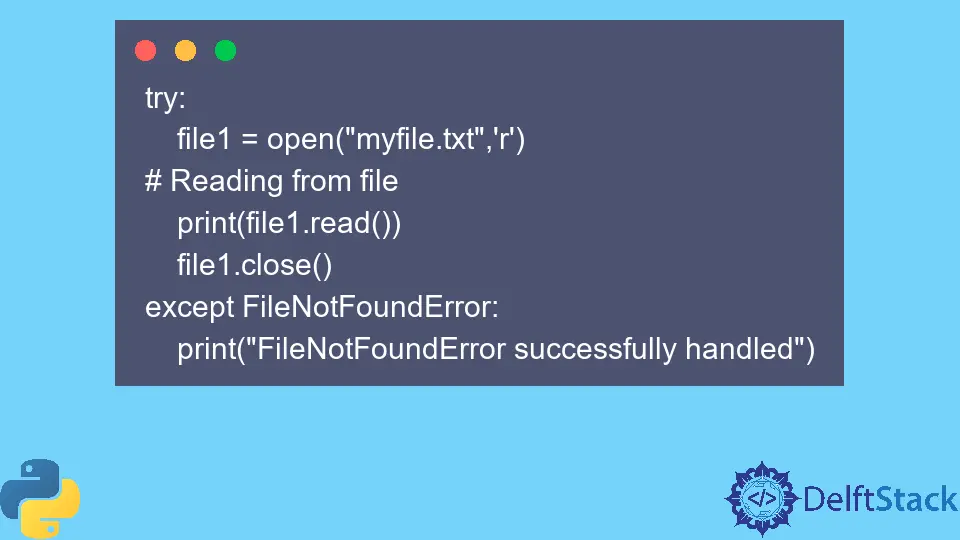
To open a file, Python has a built-in function called open()
by which the user can read or write to a file, but if, in any circumstance, the file is missing or unable to access by the compiler, so, we are encountered by a FileNotFoundError
. This article will look at how to handle Python’s file exceptions.
Python open()
File Function
This function opens the file, loads all the content, and return it as a file object.
General Syntax:
open(filename, mode="r")
This function takes two arguments. One is the file name or the whole file path; the other is access mode, which decides what action must be performed on a file.
There are various modes, including r
(read-only), w
(write-only), a
(append-only), rb
(Read-only in Binary format), etc.
Python Open File Exception
Suppose we are trying to open a file that does not exist or mistakenly entered the wrong file path, resulting in a FileNotFound
exception.
Example Code:
file1 = open("myfile.txt", "r")
# Reading from file
print(file1.read())
file1.close()
Output:
FileNotFoundError Traceback (most recent call last)
C:\Users\LAIQSH~1\AppData\Local\Temp/ipykernel_4504/3544944463.py in <module>
----> 1 file1 = open("myfile.txt",'r')
2
3 # Reading from file
4 print(file1.read())
5
FileNotFoundError: [Errno 2] No such file or directory: 'myfile.txt'
We see that the open
function is giving an error that says No such file or directory
because the file to be open is found missing by the compiler.
Use try-except
to Handle Exceptions When Reading a File in Python
One of the finest cures to this missing file problem is that the code is ambiguous and contains some errors. We wrap that part of our code in the try
block.
The try
block executes first. When the file is not found, the exception is raised.
The remaining code in the try
block is skipped, and the control jumps into the except
block. In the except
block, we mention the error type raised.
Exceptions are handled in the except
block. If there is no exception in the try
block, the except
clause will not execute.
Consider the following example.
try:
file1 = open("myfile.txt", "r")
# Reading from file
print(file1.read())
file1.close()
except FileNotFoundError:
print("FileNotFoundError successfully handled")
Output:
FileNotFoundError successfully handled
Since there was an error in the try
block, the statement in the except
block was printed in the output. So by using this trick, we can handle exceptions in our code by displaying any message in the output despite getting an error message.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn