WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
-
Use the
geckodriver.exe
File and Add It to the SystemPATH
-
Use the
executable_path
Parameter in thewebdriver.Firefox()
Function -
Use the
webdriver-manager
Module
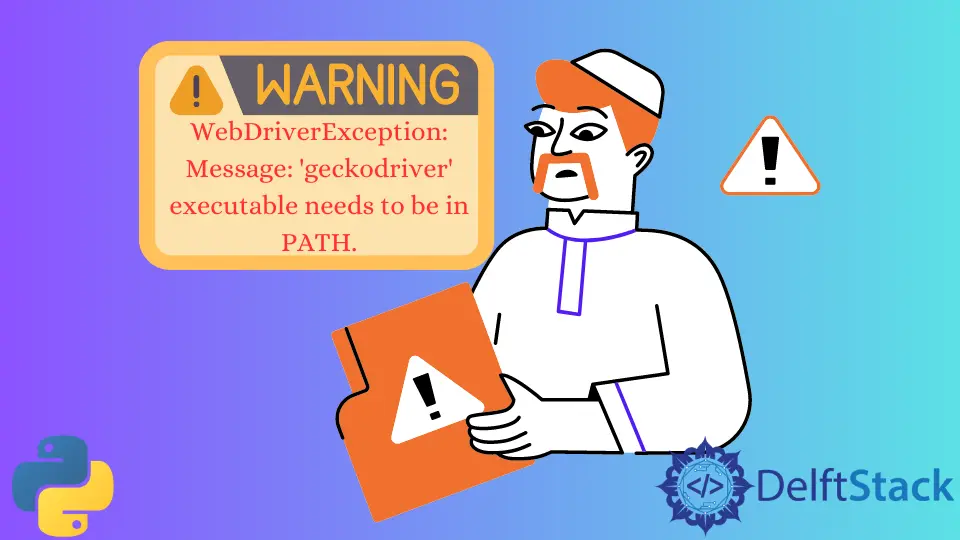
The selenium
package in Python can automate tasks on a web browser. Using their web drivers, we can use different web browsers like Google Chrome, Firefox, and more.
This tutorial will discuss the Message: 'geckodriver' executable needs to be in PATH
error in Python.
The geckodriver
is a Mozilla-developed browser engine that acts as a link between Selenium and the Firefox browser. This error occurs when the driver is not installed properly, or its path is not specified appropriately.
See the code below.
from selenium import webdriver
browser = webdriver.Firefox()
Output:
WebDriverException: Message: 'geckodriver' executable needs to be in PATH.
Let us now discuss different ways to solve this error.
Use the geckodriver.exe
File and Add It to the System PATH
Selenium tries to identify the driver executable from the system environment variable PATH
. We can add the executable path of the geckodriver
to this variable.
First, we must download the driver’s executable from the official Mozilla website. We need to add the path of the directory that contains this executable to the PATH
variable discussed previously.
The PATH
variable can be found under the Environment Variables
menu. We need to right-click the This PC
icon, go to Properties
, and select the Advance Settings
option to get this menu.
Linux users can copy the executable file directly to the /usr/local/bin
directory.
Use the executable_path
Parameter in the webdriver.Firefox()
Function
We use the webdriver.Firefox()
constructor to create the Driver
object that can open the browser window and perform the automated tasks. We can specify the path of the geckodriver
executable within this function using the executable_path
parameter.
For example:
from selenium import webdriver
driver = webdriver.Firefox(executable_path=r"user\pathofdriver\geckodriver.exe")
Mac OS users can also install the geckodriver
using homebrew
. The following command can be utilized.
brew install geckodriver
After installation, the path of the driver is shown. We can copy this path, paste it into the Finder
application, and click Go to Folder
.
This will return the full path of the driver that can be used in the executable_path
parameter.
Use the webdriver-manager
Module
The webdriver-manager
module was introduced to provide some relief in managing the web drivers of different browsers.
We can use the GeckoDriverManager().install()
functions to install and use the executable for the geckodriver
. This needs to be specified in the executable_path
parameter discussed previously.
See the code below.
from selenium import webdriver
from webdriver_manager.firefox import GeckoDriverManager
driver_object = webdriver.Firefox(executable_path=GeckoDriverManager().install())
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python