How to Open and Close Tabs in a Browser Using Selenium Python
- Install Selenium and Chrome WebDriver
- Open a Tab in a Browser Using Selenium Python
- Open a New Tab in a Browser Using Selenium Python
- Close a Tab in a Browser Using Selenium Python
- Close a Tab and Switch to Another Tab in a Browser Using Selenium Python
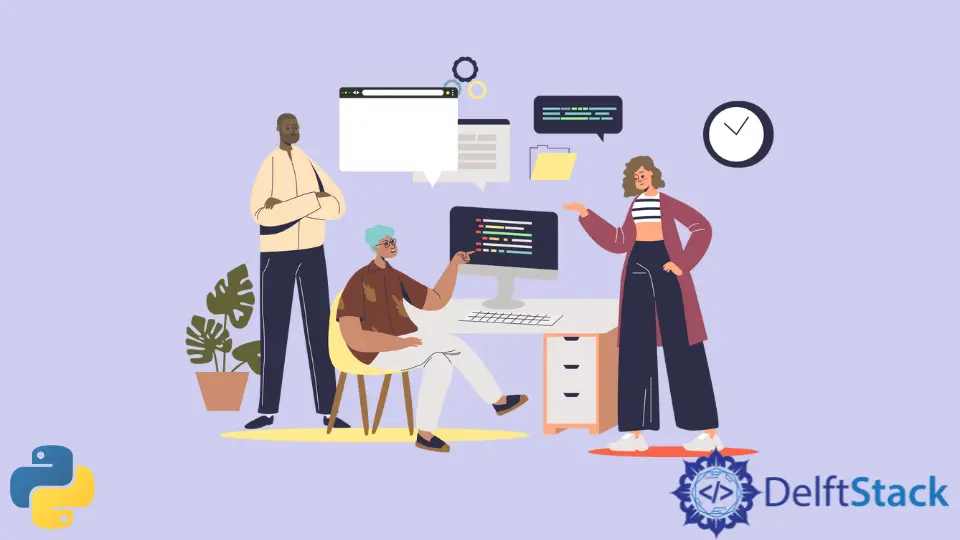
Selenium is powerful web automation and testing tool. We write scripts using Selenium, which takes control over web browsers and performs specific actions.
In this guide, we will write a script in Python that will automatically open and close a website in a new tab.
Install Selenium and Chrome WebDriver
To install Selenium, we use the following command.
#Python 3.x
pip install selenium
ChromeDriver is another executable that Selenium WebDriver uses to interact with Chrome. If we want to automate tasks on the Chrome web browser, we also need to install ChromeDriver.
Based on the version of the Chrome browser, we need to select a compatible driver for it. Following are the steps to install and configure the Chrome driver:
- Click on this link. Download Chrome driver according to the version of your Chrome browser and the type of operating system.
- If you want to find the version of your Chrome browser, click on the three dots on the top right corner of Chrome, click on Help, and select About Google Chrome. You can see the Chrome version in the about section.
- Extract the zip file and run the Chrome driver.
Open a Tab in a Browser Using Selenium Python
We created the WebDriver instance in the following code and specified the path to the Chrome driver. Then, we have set the URL of the target website using the get()
method with the driver instance.
It will open the target website in the Chrome browser.
Example code:
# Python 3.x
from selenium import webdriver
driver = webdriver.Chrome(r"E:\download\chromedriver.exe")
driver.get("https://www.verywellmind.com/what-is-personality-testing-2795420")
Output:
Open a New Tab in a Browser Using Selenium Python
To open a new tab in the same browser window, we will use the JavaScript executer. It executes JavaScript commands using the execute_script()
method.
We will pass the JavaScript command to this method as an argument. We will use the window.open()
command to open another tab in the window.
The window handle stores the unique address of the windows opened in the web browser. The switch_to_window()
method switches to the specified window address.
1
represents the address of the second window. Finally, we will provide the URL of the new website using the get()
method.
Example code:
# Python 3.x
from selenium import webdriver
driver = webdriver.Chrome(r"E:\download\chromedriver.exe")
driver.get("https://www.verywellmind.com/what-is-personality-testing-2795420")
driver.execute_script("window.open('');")
driver.switch_to.window(driver.window_handles[1])
driver.get(
"https://www.indeed.com/career-advice/career-development/types-of-personality-test"
)
Output:
Close a Tab in a Browser Using Selenium Python
We will use the close()
method with the driver to close the tab.
Example code:
# Python 3.x
from selenium import webdriver
driver = webdriver.Chrome(r"E:\download\chromedriver.exe")
url = "https://www.16personalities.com/free-personality-test"
driver.get(url)
driver.close()
Close a Tab and Switch to Another Tab in a Browser Using Selenium Python
Using Selenium in the following code, we have opened a URL in a tab. We opened another tab and switched to it using the switch_to.window(driver.window_handles[1])
.
The new tab will open the specified URL. Now, we will close this tab using the close()
method and switch back to the previous tab using the switch_to.window(driver.window_handles[0])
method.
Example code:
# Python 3.x
from selenium import webdriver
driver = webdriver.Chrome(r"E:\download\chromedriver.exe")
url = "https://www.16personalities.com/free-personality-test"
driver.get(url)
driver.execute_script("window.open('');")
driver.switch_to.window(driver.window_handles[1])
driver.get("https://www.16personalities.com/personality-types")
driver.close()
driver.switch_to.window(driver.window_handles[0])
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn