How to Login to a Website Using Selenium Python
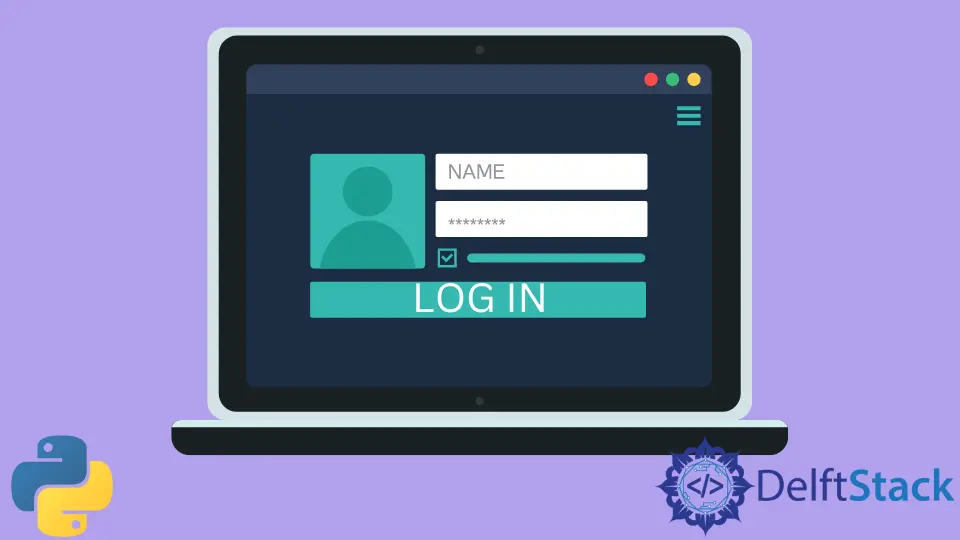
This article will demonstrate how to log in to a website using Python.
First, we will explain why we need to log in through some programming mechanism instead of manual logins. Then, we will see the available method for login into a website using Python.
Benefits of Automated Login
An automatic web login can be a good option when you want to change your account setting or want to extract some information or data. In the modern era of science, the new fuel is data.
In Python, Selenium WebDriver
is a highly well-liked framework for logging into a website and acquiring the required data.
Login to a Website Through Selenium WebDriver
in Python
Selenium WebDriver drives a browser natively, as a user would, either locally or on a remote machine using the Selenium server, marks a leap forward in terms of browser automation.
Selenium WebDriver
is a browser-controlling library that is easy to use and supports many browsers, including Chrome, Firefox, Mozilla, etc. Let’s start with how to use Selenium WebDriver
for logging in.
First, install the selenium
library.
!pip install requests
!pip install bs4
!pip install html5lib
!pip install selenium
The next step is to install the web driver specific to your browser. Since we are using Chrome, we will install the Chrome Driver.
You may see other drivers related to your use case on this page.
For this tutorial, we will be logging into GitHub. GitHub is a CI and CD platform for developers.
Run the following code in Google’s Colab.
text
!apt-get update
!apt install chromium-chromedriver
cp /usr/lib/chromium-browser/chromedriver /usr/bin
import sys
sys.path.insert(0,’/usr/lib/chromium-browser/chromedriver')
<!--adsense-->
We installed the Chrome Driver in the above code snippet and then set its path. You may download the driver and place it into your current working directory if you use a Python interpreter on your local system.
```python
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("--headless")
chrome_options.add_argument("--no-sandbox")
chrome_options.add_argument("--disable-dev-shm-usage")
# Chrome driver initialization
driver = webdriver.Chrome("chromedriver", chrome_options=chrome_options)
# Credential for my login to GitHub
username = "username"
password = "password"
Next, we set the driver arguments as described above. We can set username and password credentials with original values.
We must inspect its HTML for login fields to log in to any website. You visit the GitHub page and go to its login page.
Go to the username field, right-click, and click Inspect Element. The Inspect Element Developer Tool will highlight the HTML code for the login field.
Now, right-click on it and copy XPath
. We will use it in the following code.
from selenium.webdriver.common.by import By
The latest selenium
version includes a new class, By
, to access the HTML elements. There are plenty of options to access an element using this class.
These are as follows:
- Locating by Id
- Locating by Name
- Locating by XPath
You may find their details on the following page.
# get the login page
driver.get("https://github.com/log in")
# find a login user field and send the username value
driver.find_element(By.XPATH, """//*[@id="log in_field"]""").send_keys(username)
# insert password to the Git Login password field
driver.find_element(By.XPATH, """//*[@id="password"]""").send_keys(password)
# click submit button on the login page
driver.find_element(By.XPATH, """//*[@id="log in"]/div[4]/form/div/input[12]""").click()
# wait for 10 seconds to complete the ready state field
WebDriverWait(driver=driver, timeout=10).until(
lambda x: x.execute_script("return document.readyState === 'complete'")
)
errors = driver.find_element(By.CLASS_NAME, "flash-error")
The driver.get()
function returns the login page. Then you access the username, password and login button fields using the find_element()
function.
After that, we need to send these credentials using the send_keys()
function.
We wait for 10 seconds and see if logging in is successful or not. If there is any error, we may access it using the below code.
driver.current_url
You may check whether the login is successful or not by using the current_url()
function. The above code returns the output as given below.
https://github.com/session
It means we have started the GitHub session by logging into our account.
For logging into any other website, you can follow the same guidelines. But to access the elements, you need to make sure you are using the right HTML tag ids for login.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn