How to Check if Element Exists Using Selenium Python
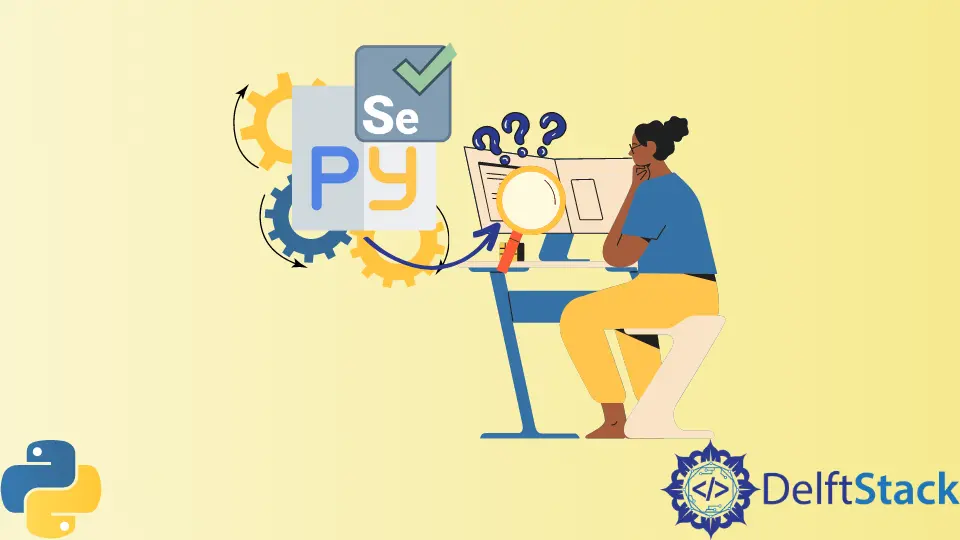
Automation tools like Selenium allow us to automate web processes and test applications via different languages and browsers. Python is one of many languages it supports and is a very easy language.
Its Python client helps us to connect with browsers through Selenium tools. Web testing is vital to developing web applications, but more than that, it allows us to automate web processes.
We need access to the source code and check for certain elements to automate such processes.
This article shows you how to check if an element exists in Selenium using its Python client and API.
Use find_element()
to Check if Element Exists Using Selenium Python
To make use of the Selenium Python client, we need to install its package via this pip
command:
pip install selenium
Aside from the Python client, we need to have other tools installed if we are to use them, such as the ChromeDriver. You can download and install it fairly easily.
Now, we can use the Selenium
module and its Exception
section to check if an element exists. First, we get to use the webdriver
module to access the browser agent (Chrome) and use the get()
method to access the webpage we want to check its elements.
Afterward, use the find_element()
method, and pass the By.TAG_NAME
argument and the element you want to find (e.g., h2
). The find_element()
method uses the By
strategy and locator to find elements.
In the code below, we use the By.TAG_NAME
strategy to find the element we want. We can also use By.CSS_SELECTOR
to find elements.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
driver = webdriver.Chrome(executable_path="C:\chromedriver.exe")
driver.implicitly_wait(0.5)
driver.get("https://thehackernews.com/")
try:
element = driver.find_element(By.TAG_NAME, "h2")
hackHead = element.text
print("Element exist")
print(hackHead)
except NoSuchElementException:
print("Element does not exist")
driver.close()
Output:
DeprecationWarning: executable_path has been deprecated, please pass in a Service object
driver = webdriver.Chrome(executable_path="C:\chromedriver.exe")
DevTools listening on ws://127.0.0.1:57551/devtools/browser/dce0d9db-6c42-402e-8770-13999aff0e79
Element exist
Pay What You Want for This Collection of White Hat Hacking Courses
We obtained Pay What You Want for This Collection of White Hat Hacking Courses
as the element’s content, but you might notice a DeprecationWarning
around the executable_path
.
DeprecationWarning: executable_path has been deprecated, please pass in a Service object
driver = webdriver.Chrome(executable_path="C:\chromedriver.exe")
To deal with that, we need to install the webdriver-manager
module to handle browser interactions using the pip
command.
pip install webdriver-manager
Afterward, import the module to your code using the statements below.
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service
And use the service
attribute instead of executable_path
, and pass the Service()
and ChromeDriverManager()
methods to the service
attribute.
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
Now, the code becomes:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
driver.implicitly_wait(0.5)
driver.get("https://thehackernews.com/")
try:
l = driver.find_element(By.TAG_NAME, "h2")
s = l.text
print("Element exist -" + s)
except NoSuchElementException:
print("Element does not exist")
driver.close()
Output:
[WDM] - Downloading: 100%|████████████████████████████████████████████████████████████████████████████████████████████████| 6.29M/6.29M [00:03<00:00, 2.13MB/s]
DevTools listening on ws://127.0.0.1:57442/devtools/browser/2856cae0-e665-42c3-a20d-a847d52658c1
Element exist
Pay What You Want for This Collection of White Hat Hacking Courses
Because it’s the first time running, you might see the [WDM]
part of the output; otherwise, it’s just the DevTools
message and your code output that should be visible. With this, you can easily check if an element exists in Selenium using its Python client.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn