Selenium Python을 사용하여 요소가 존재하는지 확인
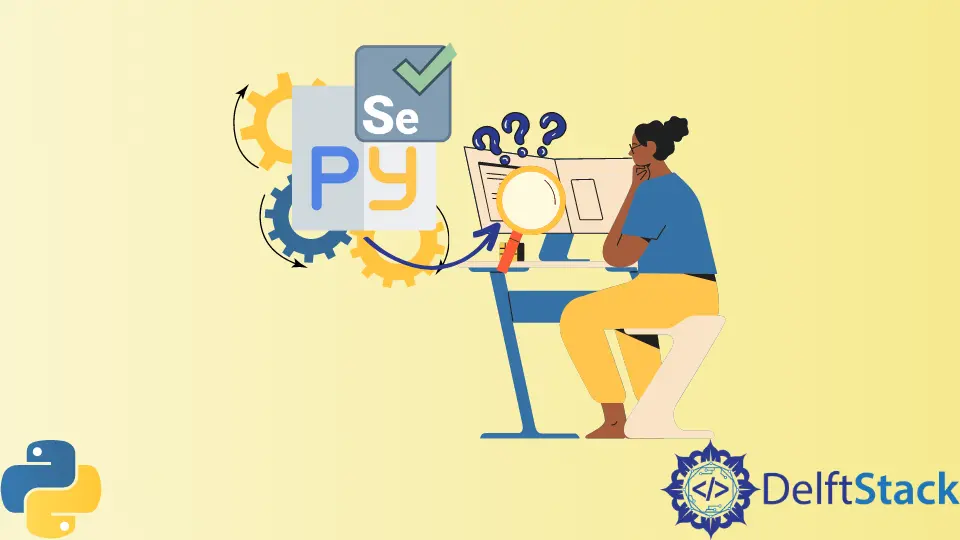
Selenium과 같은 자동화 도구를 사용하면 웹 프로세스를 자동화하고 다양한 언어와 브라우저를 통해 애플리케이션을 테스트할 수 있습니다. Python은 지원하는 많은 언어 중 하나이며 매우 쉬운 언어입니다.
Python 클라이언트는 Selenium 도구를 통해 브라우저와 연결할 수 있도록 도와줍니다. 웹 테스트는 웹 애플리케이션 개발에 필수적이지만 그 이상으로 웹 프로세스를 자동화할 수 있습니다.
이러한 프로세스를 자동화하려면 소스 코드에 액세스하고 특정 요소를 확인해야 합니다.
이 기사에서는 Python 클라이언트 및 API를 사용하여 Selenium에 요소가 있는지 확인하는 방법을 보여줍니다.
find_element()
를 사용하여 Selenium Python을 사용하여 요소가 존재하는지 확인
Selenium Python 클라이언트를 사용하려면 다음 pip
명령을 통해 해당 패키지를 설치해야 합니다.
pip install selenium
Python 클라이언트 외에도 ChromeDriver와 같은 다른 도구를 사용하려면 설치해야 합니다. 상당히 쉽게 다운로드하여 설치할 수 있습니다.
이제 Selenium
모듈과 Exception
섹션을 사용하여 요소가 있는지 확인할 수 있습니다. 먼저 webdriver
모듈을 사용하여 브라우저 에이전트(Chrome)에 액세스하고 get()
메서드를 사용하여 해당 요소를 확인하려는 웹 페이지에 액세스합니다.
그런 다음 find_element()
메서드를 사용하고 By.TAG_NAME
인수와 찾으려는 요소(예: h2
)를 전달합니다. find_element()
메서드는 By
전략과 로케이터를 사용하여 요소를 찾습니다.
아래 코드에서는 By.TAG_NAME
전략을 사용하여 원하는 요소를 찾습니다. By.CSS_SELECTOR
를 사용하여 요소를 찾을 수도 있습니다.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
driver = webdriver.Chrome(executable_path="C:\chromedriver.exe")
driver.implicitly_wait(0.5)
driver.get("https://thehackernews.com/")
try:
element = driver.find_element(By.TAG_NAME, "h2")
hackHead = element.text
print("Element exist")
print(hackHead)
except NoSuchElementException:
print("Element does not exist")
driver.close()
출력:
DeprecationWarning: executable_path has been deprecated, please pass in a Service object
driver = webdriver.Chrome(executable_path="C:\chromedriver.exe")
DevTools listening on ws://127.0.0.1:57551/devtools/browser/dce0d9db-6c42-402e-8770-13999aff0e79
Element exist
Pay What You Want for This Collection of White Hat Hacking Courses
우리는 Pay What You Want for This Collection of White Hat Hacking Courses
를 요소 콘텐츠로 얻었지만 executable_path
주변에 DeprecationWarning
이 표시될 수 있습니다.
DeprecationWarning: executable_path has been deprecated, please pass in a Service object
driver = webdriver.Chrome(executable_path="C:\chromedriver.exe")
이를 처리하려면 pip
명령을 사용하여 브라우저 상호 작용을 처리하기 위해 webdriver-manager
모듈을 설치해야 합니다.
pip install webdriver-manager
그런 다음 아래 명령문을 사용하여 모듈을 코드로 가져옵니다.
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service
그리고 executable_path
대신 service
속성을 사용하고 Service()
및 ChromeDriverManager()
메서드를 service
속성에 전달합니다.
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
이제 코드는 다음과 같습니다.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
driver.implicitly_wait(0.5)
driver.get("https://thehackernews.com/")
try:
l = driver.find_element(By.TAG_NAME, "h2")
s = l.text
print("Element exist -" + s)
except NoSuchElementException:
print("Element does not exist")
driver.close()
출력:
[WDM] - Downloading: 100%|████████████████████████████████████████████████████████████████████████████████████████████████| 6.29M/6.29M [00:03<00:00, 2.13MB/s]
DevTools listening on ws://127.0.0.1:57442/devtools/browser/2856cae0-e665-42c3-a20d-a847d52658c1
Element exist
Pay What You Want for This Collection of White Hat Hacking Courses
처음 실행되기 때문에 출력의 [WDM]
부분을 볼 수 있습니다. 그렇지 않으면 DevTools
메시지와 코드 출력만 표시되어야 합니다. 이를 통해 Python 클라이언트를 사용하여 Selenium에 요소가 있는지 쉽게 확인할 수 있습니다.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn