Python에서 Selenium으로 요소 찾기
- Python에서 Selenium으로 요소 찾기
-
Python에서
find_elements_by_name()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_id()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_xpath()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_link_text()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_partial_link_text()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_tag_name()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_class_name()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements_by_css_selector()
함수를 사용하여 Selenium이 있는 요소 찾기 -
Python에서
find_elements()
함수를 사용하여 Selenium이 있는 요소 찾기 - 결론
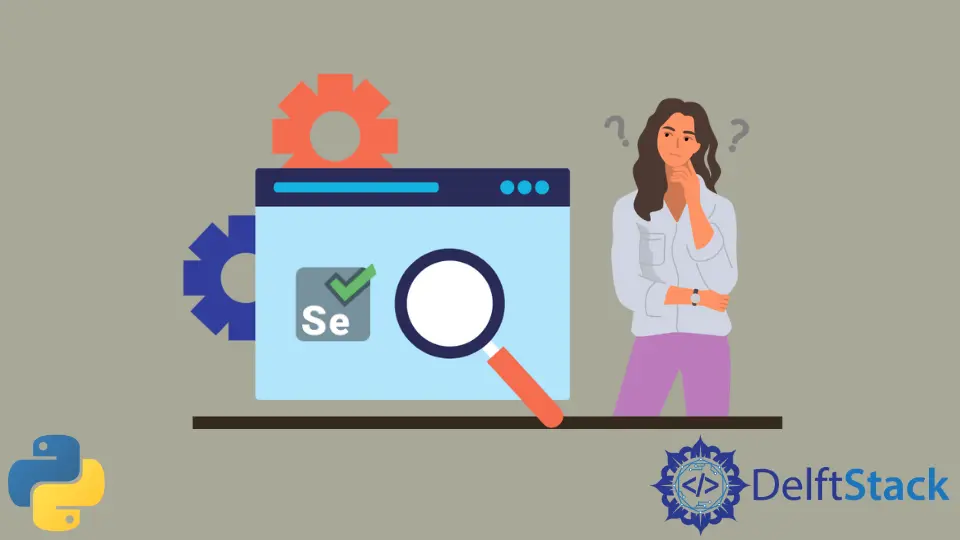
Python의 셀레늄 패키지는 웹 브라우저 자동화에 사용됩니다. 거의 모든 주요 브라우저와 호환됩니다. 브라우저에서 일부 작업을 자동화하는 스크립트를 Python으로 작성할 수 있습니다.
이 튜토리얼은 Python에서 셀레늄을 사용하여 웹 페이지에서 요소를 찾는 다양한 방법을 보여줍니다.
Python에서 Selenium으로 요소 찾기
요소는 웹 페이지의 기본 구조이며 구조를 정의하는 데 사용됩니다. 셀레늄의 다른 기능을 사용하여 원소를 찾을 수 있습니다.
이러한 함수는 name
, xpath
, id
등과 같은 다양한 속성을 사용하여 요소를 찾는 데 사용됩니다. 아래의 HTML 문서에서 요소를 검색하는 방법을 사용합니다.
<html>
<body>
<p class="content">Some text</p>
<a href="link.html"> Link_text </a>
<form id="some_form">
<input name="email" type="text" />
<input name="pass" type="password" />
</form>
</body>
</html>
어떤 경우에도 일치하는 항목이 없으면 NoSuchElementException
예외가 발생합니다. 모두 일치하는 요소 목록을 반환합니다.
방법은 아래에서 설명합니다.
Python에서 find_elements_by_name()
함수를 사용하여 Selenium이 있는 요소 찾기
웹 페이지의 다른 요소에는 이름
속성이 지정되어 있습니다. find_elements_by_name()
함수를 사용하여 name
속성 값과 일치하는 요소 목록을 검색할 수 있습니다.
아래 코드를 참조하십시오.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_name("email")
위의 코드를 이해해 봅시다.
- 자동화를 위한 브라우저를 만들기 위해 먼저
webdriver
클래스를 가져옵니다. 이 경우 Google Chrome 브라우저에chromedriver.exe
를 사용합니다. get()
기능을 사용하여 요소를 가져오려는 웹사이트를 검색합니다.- 요소 목록을 가져오려면
find_element_by_name()
함수를 사용하고 함수 내에서name
속성 값을 지정합니다.
코드는 아래에서 설명하는 모든 방법에 대해 동일하게 유지됩니다. 요소를 검색하는 함수(이 경우 find_element_by_name()
)만 변경됩니다.
Python에서 find_elements_by_id()
함수를 사용하여 Selenium이 있는 요소 찾기
id
특성은 웹 페이지에서 찾은 요소 목록을 반환할 수도 있습니다. 이를 위해 find_elements_by_id()
함수를 사용할 수 있습니다.
예를 들어,
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_id("some_form")
Python에서 find_elements_by_xpath()
함수를 사용하여 Selenium이 있는 요소 찾기
xpath
를 사용하여 경로 표현식을 사용하여 문서에서 노드를 검색할 수 있습니다. xpath
를 사용하여 요소를 찾으려면 find_elements_by_xpath()
함수를 사용할 수 있습니다.
경로 표현식은 함수에 지정됩니다.
예를 들어,
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_xpath("/html/body/form[1]")
Python에서 find_elements_by_link_text()
함수를 사용하여 Selenium이 있는 요소 찾기
문서에는 다른 웹 페이지로 리디렉션할 수 있는 요소가 있습니다. 이것은 앵커
태그를 사용하여 달성됩니다.
find_elements_by_link_text()
함수를 사용하여 링크 텍스트를 사용하여 요소를 검색할 수 있습니다.
아래 코드를 참조하십시오.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_link_text("Link_text")
Python에서 find_elements_by_partial_link_text()
함수를 사용하여 Selenium이 있는 요소 찾기
이 기능은 이전 기능과 유사합니다. 차이점은 링크의 부분 텍스트와 일치하는 요소를 반환한다는 것입니다.
예를 들어,
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_partial_link_text("Link_")
위의 예에서 부분 링크 텍스트를 사용하여 요소를 검색할 수 있음을 관찰할 수 있습니다.
Python에서 find_elements_by_tag_name()
함수를 사용하여 Selenium이 있는 요소 찾기
HTML 문서의 모든 요소에는 태그 이름이 있습니다. 태그 이름을 사용하여 요소를 찾으려면 Python에서 find_element_by_tag_name()
을 사용할 수 있습니다.
아래 코드를 참조하십시오.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_tag_name("form")
Python에서 find_elements_by_class_name()
함수를 사용하여 Selenium이 있는 요소 찾기
find_elements_by_class_name()
함수를 사용하여 웹 페이지에서 주어진 class
속성과 일치하는 요소를 검색할 수 있습니다.
아래 코드를 참조하십시오.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_class_name("content")
Python에서 find_elements_by_css_selector()
함수를 사용하여 Selenium이 있는 요소 찾기
CSS 선택기 구문을 사용하여 HTML 요소의 스타일을 지정할 수 있습니다. CSS 선택기 구문을 사용하여 find_elements_by_css_selector()
함수로 요소를 찾을 수도 있습니다.
다음 예를 참조하십시오.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_css_selector("p.content")
Python에서 find_elements()
함수를 사용하여 Selenium이 있는 요소 찾기
find_elements()
함수는 HTML 문서에서 요소를 검색할 수 있는 전용 메서드입니다. 그것은 이전에 논의된 모든 방법의 정점과 같습니다.
이전 속성을 모두 매개변수로 사용하여 요소를 찾을 수 있습니다.
예를 들어,
from selenium.webdriver.common.by import By
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_elements(By.NAME, "email")
위의 예는 이전과 같이 name
속성을 사용하여 요소를 찾습니다.
결론
Python에서 셀레늄을 사용하여 다양한 속성을 기반으로 요소를 찾는 다양한 방법에 대해 논의했습니다. 모든 속성에는 기능이 있습니다.
또한 개인 메서드인 find_elements
를 사용하고 다른 속성을 매개 변수로 제공할 수 있습니다. 주목해야 할 또 다른 사항은 이 문서의 메서드가 가능한 모든 일치 항목에 대한 요소 목록을 반환한다는 것입니다.
하나의 요소만 찾아야 하는 경우 논의된 각 함수에서 요소
를 요소
로 대체할 수 있습니다. 예를 들어 find_elements
는 find_element
가 됩니다.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn