How to Find Elements With Selenium in Python
- Find Elements With Selenium in Python
-
Use the
find_elements_by_name()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_id()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_xpath()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_link_text()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_partial_link_text()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_tag_name()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_class_name()
Function to Find Elements With Selenium in Python -
Use the
find_elements_by_css_selector()
Function to Find Elements With Selenium in Python -
Use the
find_elements()
Function to Find Elements With Selenium in Python - Conclusion
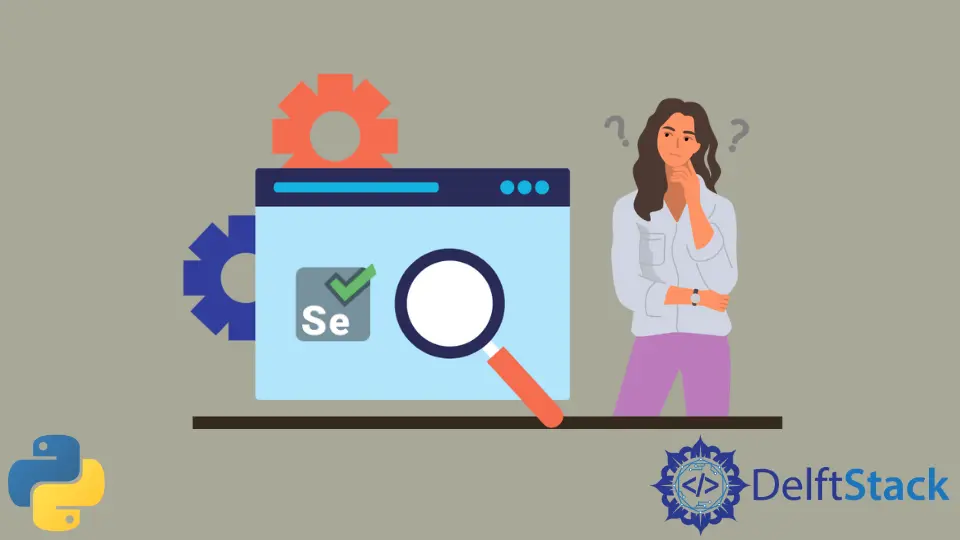
The selenium package in Python is used for automation with web browsers; it is compatible with almost all major browsers. We can write scripts in Python that will automate some tasks on a browser.
This tutorial will demonstrate different methods to find elements in a webpage using selenium in Python.
Find Elements With Selenium in Python
The elements are the basic constructs of a webpage and are used to define its structure. We can find elements using different functions of selenium.
These functions are used to find the elements using different attributes like name
, xpath
, id
, and more. We will use the methods to retrieve elements from the HTML document below.
<html>
<body>
<p class="content">Some text</p>
<a href="link.html"> Link_text </a>
<form id="some_form">
<input name="email" type="text" />
<input name="pass" type="password" />
</form>
</body>
</html>
Note that if there are no matches in any case, then a NoSuchElementException
exception is raised. They will all return a list of elements where the match is found.
The methods are discussed below.
Use the find_elements_by_name()
Function to Find Elements With Selenium in Python
Different elements on a web page have a name
attribute assigned. We can use the find_elements_by_name()
function to retrieve the list of elements that match the value of the name
attribute.
See the code below.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_name("email")
Let us understand the above code.
- We first import the
webdriver
class to create the browser for automation. In our case, we will use thechromedriver.exe
for the Google Chrome browser. - We retrieve the website from which we wish to get the elements using the
get()
function. - To get the list of elements, we use the
find_element_by_name()
function and specify the value for thename
attribute within the function.
Note that the code will remain the same for every method discussed below. Only the function to retrieve the elements (in this case find_element_by_name()
) will change.
Use the find_elements_by_id()
Function to Find Elements With Selenium in Python
The id
attribute can also return a list of elements found on a web page. For this, we can use the find_elements_by_id()
function.
For example,
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_id("some_form")
Use the find_elements_by_xpath()
Function to Find Elements With Selenium in Python
We can use the path expressions using xpath
to retrieve nodes from a document. To find elements using the xpath
, we can use the find_elements_by_xpath()
function.
The path expression is specified in the function.
For example,
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_xpath("/html/body/form[1]")
Use the find_elements_by_link_text()
Function to Find Elements With Selenium in Python
We have elements in the document that can redirect to other web pages. This is achieved using the anchor
tag.
Using the find_elements_by_link_text()
function, we can retrieve the elements using the link text.
See the code below.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_link_text("Link_text")
Use the find_elements_by_partial_link_text()
Function to Find Elements With Selenium in Python
This function is similar to the previous one. The difference is that it returns the elements that match the partial text of the link.
For example,
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_partial_link_text("Link_")
In the above example, we can observe that we were able to retrieve the element by just using the partial link text.
Use the find_elements_by_tag_name()
Function to Find Elements With Selenium in Python
Every element in the HTML document has a tag name. To find elements using the tag name, we can use the find_element_by_tag_name()
in Python.
See the code below.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_tag_name("form")
Use the find_elements_by_class_name()
Function to Find Elements With Selenium in Python
We can use the find_elements_by_class_name()
function to retrieve the elements that match a given class
attribute on a web page.
See the code below.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_class_name("content")
Use the find_elements_by_css_selector()
Function to Find Elements With Selenium in Python
We can style HTML elements using the CSS selector syntax. The CSS selector syntax can also be used to find the elements with the find_elements_by_css_selector()
function.
See the following example.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_element_by_css_selector("p.content")
Use the find_elements()
Function to Find Elements With Selenium in Python
The find_elements()
function is a private method that can retrieve elements from the HTML document. It is like the culmination of all the previously discussed methods.
We can use all the previous attributes as parameters to find the elements.
For example,
from selenium.webdriver.common.by import By
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.get("https://www.sample.org/")
e = driver.find_elements(By.NAME, "email")
The above example will find the elements using the name
attribute as done previously.
Conclusion
We have discussed different methods to find elements based on different attributes with selenium in Python. Every attribute has its function.
We can also use the private method find_elements
and provide different attributes as parameters. Another thing to note is that the methods in this article return a list of elements for all possible matches.
If we need to find only one element, we can substitute elements
for element
in each discussed function. For example, find_elements
becomes find_element
.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python