How to Convert JSON to Dictionary in Python
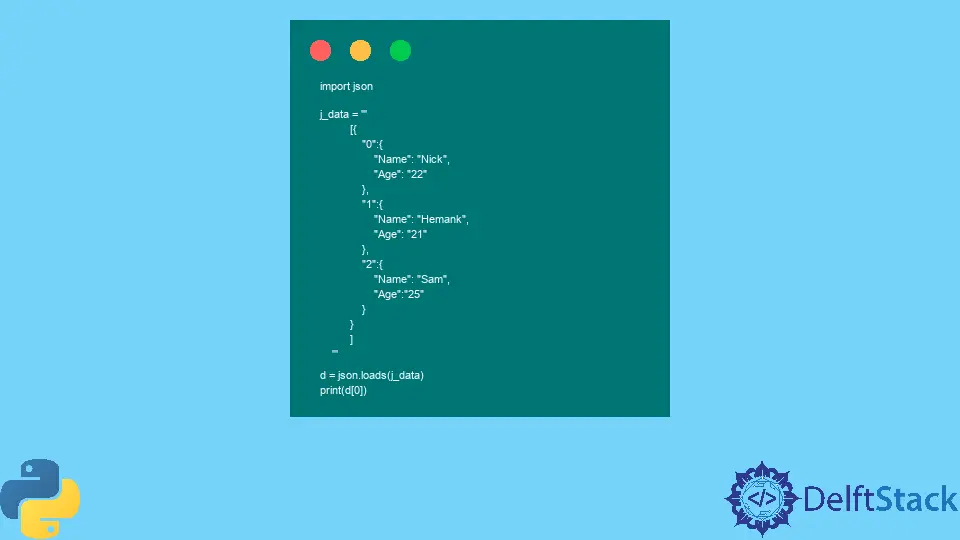
JSON is JavaScript Object Notation and is used for storing and transferring data. Python programming language supports JSON through a built-in library package called json
. Its format is very similar to a dictionary or a list in Python.
In this tutorial, we will convert a JSON string to a dictionary in Python.
The json.load()
function is used to parse the JSON string. The final type returned by this function depends on the type of JSON data it is reading. Wherever a square bracket is encountered, it reads the data as a list. When a curly brace is encountered, the final type is in a dictionary.
See the code below.
import json
j_data = """
{
"0":{
"Name": "Nick",
"Age": "22"
},
"1":{
"Name": "Hemank",
"Age": "21"
},
"2":{
"Name": "Sam",
"Age":"25"
}
}
"""
d = json.loads(j_data)
print(d)
print(d["0"]["Name"])
print(d["1"]["Age"])
Output:
{'0': {'Name': 'Nick', 'Age': '22'}, '1': {'Name': 'Hemank', 'Age': '21'}, '2': {'Name': 'Sam', 'Age': '25'}}
Nick
21
Notice the format of the JSON data in the above example. It is enclosed in curly braces. That is why the final output is in the form of the dictionary. We have also used the keys to traverse through the dictionary and access individual values.
However, if the data is enclosed in square brackets, the json.loads()
function will read the data in a list. We can select the dictionary element from the list.
For example,
import json
j_data = """
[{
"0":{
"Name": "Nick",
"Age": "22"
},
"1":{
"Name": "Hemank",
"Age": "21"
},
"2":{
"Name": "Sam",
"Age":"25"
}
}
]
"""
d = json.loads(j_data)
print(d[0])
Output:
{'0': {'Name': 'Nick', 'Age': '22'}, '1': {'Name': 'Hemank', 'Age': '21'}, '2': {'Name': 'Sam', 'Age': '25'}}
Notice the slight change in the JSON data. The final type is a list. That is why we extract the first element, which is a dictionary. We can similarly use the individual keys to traverse through the dictionary and access different values.
Related Article - Python JSON
- How to Get JSON From URL in Python
- How to Pretty Print a JSON File in Python
- How to Append Data to a JSON File Using Python
- How to Compare Multilevel JSON Objects Using JSON Diff in Python
- How to Flatten JSON in Python
- How to Serialize a Python Class Object to JSON