How to Import CSV Files Into Array in PowerShell
- The Comma-Separated Values File
-
Use the
Import-CSV
Cmdlet to Import CSV Files Into Array in PowerShell -
Use the
Get-Content
andConvertFrom-Csv
Cmdlet to Import CSV Files Into Array in PowerShell -
Use the
System.IO.File
andConvertFrom-Csv
Cmdlet to Import CSV Files Into Array in PowerShell - Conclusion
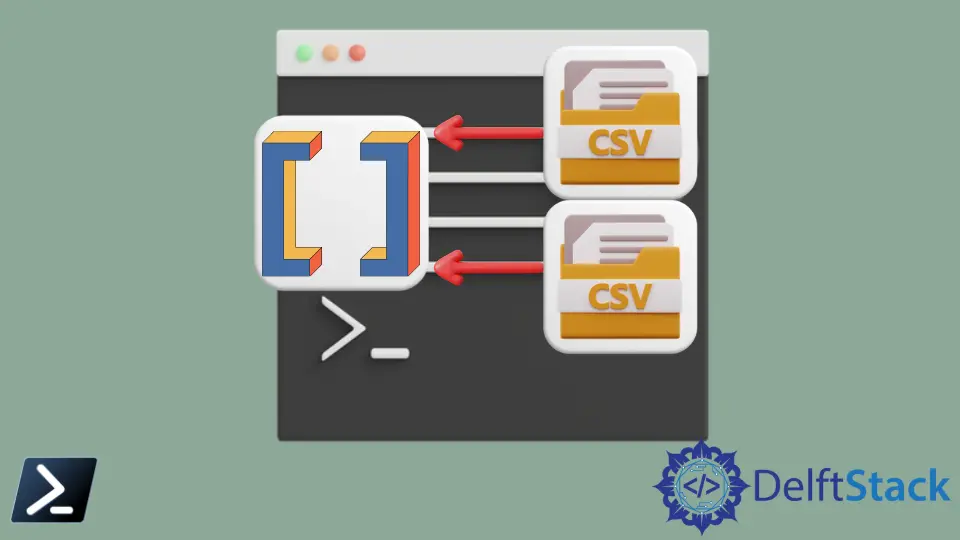
CSV (Comma-Separated Values) files serve as a fundamental data format, encapsulating information in a tabular structure with fields separated by commas. Although commonly associated with spreadsheet software, their simplistic yet versatile nature finds application across various platforms, including PowerShell.
In PowerShell scripting, efficiently reading and processing CSV data is crucial for automation and data manipulation tasks. This article explores three methods—Import-CSV
, Get-Content
cmdlets and System.IO.File
class with ConvertFrom-Csv
cmdlet—for importing CSV files into arrays, offering insights into their usage, advantages, and application scenarios.
The Comma-Separated Values File
A CSV (Comma-Separated Values) file contains data or set separated by commas. This allows the data to be saved in a tabular format.
Users can utilize CSV files with most spreadsheet programs, such as Microsoft Excel or Google Spreadsheets. However, when we open it in software that doesn’t cater to tabular formats, the data will be comma-separated, which PowerShell can use to separate values into arrays.
For example, below is a raw CSV format with two columns.
users.csv
file:
Name,Salary
John,1000
Paul,2000
Mary,1500
Matt,3000
Use the Import-CSV
Cmdlet to Import CSV Files Into Array in PowerShell
The Import-CSV
command in Windows PowerShell creates a table like custom objects from the items presented in the CSV file above.
In the Windows PowerShell script below, we will use the Import-CSV
command to assign the CSV file data to a Windows PowerShell array type variable.
$users = Import-Csv path\users.csv
$users
We start by using the Import-Csv
cmdlet to read data from a CSV file located at path\users.csv
. This cmdlet reads the content of the CSV file and converts it into a PowerShell object.
We then assign the imported CSV data to the variable $users
. This variable now holds the content of the CSV file as an array of objects, with each object representing a row from the CSV file.
Once executed, we can see the CSV file values turned to a format list called an ArrayList
object.
Output:
Access Elements After Importing CSV Files Into Array in PowerShell
When we import a CSV file using Import-Csv
in PowerShell, each row of the CSV file is represented as an object, and the entire CSV data is stored as an array of these objects. To access individual rows or elements within the CSV data, we use array indexing.
$users = Import-Csv path\users.csv
$users[0]
We start by importing a CSV file named users.csv
located at the specified path. We use the Import-Csv
cmdlet for this purpose.
This cmdlet reads the content of the CSV file and converts it into a PowerShell object.
After importing the CSV file, we want to access the first row of the CSV data. We achieve this by using array indexing.
In PowerShell, array indexing starts at 0, so $users[0]
refers to the first element (or row) in the $users
array.
Output:
We can query for the property from a particular element from the Windows PowerShell array by treating the column name as a property name like the example below.
$users = Import-Csv path/users.csv
$users[0].Name
After importing the CSV file and storing its contents in the $users
variable, we proceed to access a specific property of the first row of the CSV data. In this case, we want to retrieve the value of the Name
property from the first row.
By specifying $users[0].Name
, we are accessing the Name
property of the object representing the first row of the CSV data. This allows us to retrieve the value associated with the Name
column in the CSV file for the first row.
Output:
We can count the number of elements in an array using the Count
property below.
$users = Import-Csv path/users.csv
$users.Count
After importing the CSV file and storing its contents in the $users
variable, we want to determine the number of items (rows) in the array.
By using $users.Count
, we are accessing the Count
property of the array stored in the $users
variable. This property returns the number of items (rows) in the array.
Output:
Use the Get-Content
and ConvertFrom-Csv
Cmdlet to Import CSV Files Into Array in PowerShell
One straightforward approach to accomplish this task is by using the Get-Content
and ConvertFrom-Csv
cmdlets. This method allows us to read the contents of a CSV file as text using Get-Content
and then convert it into PowerShell objects using ConvertFrom-Csv
, thereby creating an array of objects representing each row of the CSV data.
$content = Get-Content -Path "C:\path\data.csv"
$array = $content | ConvertFrom-Csv
$array
We start by using Get-Content
to read the content of the CSV file located at the specified path and store it in the $content
variable.
Next, we pipe the content of the CSV file stored in $content
to ConvertFrom-Csv
. This cmdlet converts the CSV text into PowerShell objects and stores them in the $array
variable.
Once executed, we can see the CSV file values turned to a format list called an ArrayList
object.
Output:
Use the System.IO.File
and ConvertFrom-Csv
Cmdlet to Import CSV Files Into Array in PowerShell
The System.IO.File
class enables the reading of CSV file contents, which are then transformed into PowerShell objects using the ConvertFrom-Csv
cmdlet. This method not only provides flexibility but also ensures ease of use, empowering users to seamlessly manage CSV data within their PowerShell scripts.
$content = [System.IO.File]::ReadAllText("C:\path\data.csv")
$array = $content | ConvertFrom-Csv
$array
We use [System.IO.File]::ReadAllText
to read the content of the CSV file located at the specified path and store it in the $content
variable.
Next, we pipe the content of the CSV file stored in $content
to ConvertFrom-Csv
. This cmdlet converts the CSV text into PowerShell objects and stores them in the $array
variable.
Once executed, we can see the CSV file values turned to a format list called an ArrayList
object.
Output:
Conclusion
In PowerShell scripting, handling CSV data seamlessly is imperative for effective automation and data processing. By leveraging the Import-CSV
, Get-Content
cmdlets or the System.IO.File
class with ConvertFrom-Csv
cmdlet, users can efficiently read CSV files into arrays, empowering them to manipulate data with ease and flexibility.
Whether extracting insights from large datasets or automating repetitive tasks, these methods provide powerful tools for PowerShell developers. With a clear understanding of these techniques, users can harness the power of CSV data within their scripts, streamlining workflows and enhancing productivity.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell Array
- Array of Strings in PowerShell
- How to Create an Empty Array of Arrays in PowerShell
- Byte Array in PowerShell
- How to Pass an Array to a Function in PowerShell
- How to Sorting Array Values Using PowerShell
- How to Count the Length of Array in PowerShell