How to Append Data to a CSV File in PowerShell
-
Use
Export-CSV
With-Append
Parameter to Append Data to a CSV File in PowerShell -
Use
ConvertTo-CSV
andAdd-Content
to Append Data to a CSV File in PowerShell -
Use
ConvertTo-CSV
andOut-File
With-Append
Parameter to Append Data to a CSV File in PowerShell - Conclusion
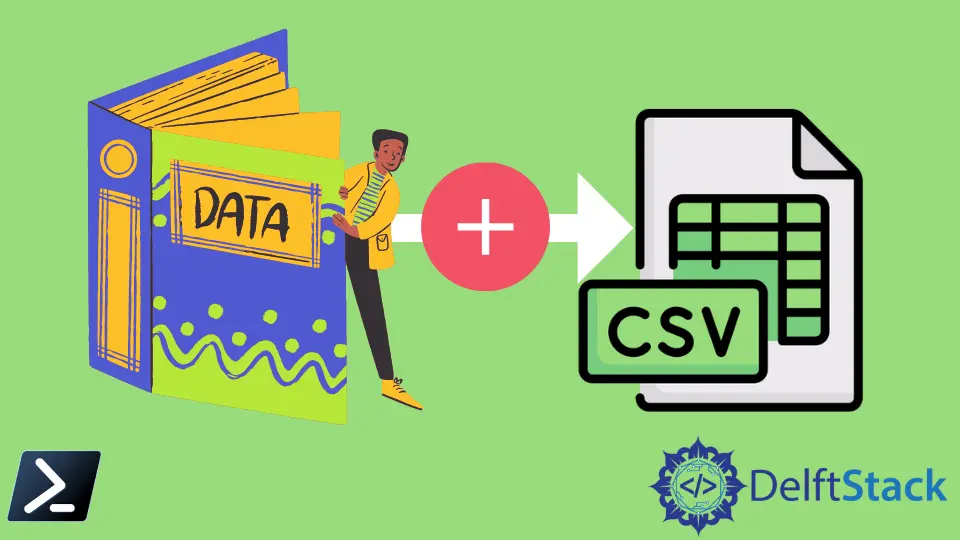
A Comma-Separated Values (CSV) file is a delimited text file that stores data in a tabular format. Each value is separated by the delimiter ,
or other characters.
The Export-Csv
cmdlet in PowerShell creates a series of CSV strings from given objects and saves them into a file. While working with CSV files on PowerShell, there are times when you will need to append a CSV file.
This tutorial will teach you to append data to a CSV file using PowerShell.
Use Export-CSV
With -Append
Parameter to Append Data to a CSV File in PowerShell
Appending data to a CSV file in PowerShell is a common task, especially when you want to add new information without overwriting existing data. The Export-CSV
cmdlet provides a convenient way to accomplish this with the -Append
parameter.
This parameter allows you to add data to an existing CSV file without erasing its contents.
Example:
$data = @{
Name = "John Doe"
Age = 30
City = "New York"
}
$obj = New-Object PSObject -Property $data
$obj | Export-Csv -Path "data.csv" -Append -NoTypeInformation
In this example, we first define a hashtable $data
containing information about an individual, including their name, age, and city. We then create a custom object $obj
from this hashtable using the New-Object
cmdlet.
Finally, we use the Export-CSV
cmdlet with the -Append
parameter to append the $obj
object to the CSV file named data.csv
. The -NoTypeInformation
parameter ensures that type information is not included in the CSV file, resulting in a cleaner output.
Output:
Use ConvertTo-CSV
and Add-Content
to Append Data to a CSV File in PowerShell
Appending data to a CSV file in PowerShell can be accomplished using the combination of the ConvertTo-CSV
cmdlet and the Add-Content
cmdlet. This method is useful when you want to add new data to an existing CSV file without overwriting its contents.
By converting data to CSV format and then appending it to the file, you can seamlessly update your CSV files with new information.
Example:
$data = @{
Name = "John Doe"
Age = 30
City = "New York"
}
$csvData = $data | ConvertTo-Csv -NoTypeInformation
Add-Content -Path "data.csv" -Value $csvData
In this example, we first create a custom object $obj
from the $data
hashtable using the New-Object
cmdlet. Then, we use ConvertTo-CSV
to convert the custom object to CSV format, storing the result in the variable $csvData
.
Finally, we append the CSV data to the file data.csv
using Add-Content
. This ensures that the correct data structure is used for conversion to CSV format before appending it to the file.
Output:
Use ConvertTo-CSV
and Out-File
With -Append
Parameter to Append Data to a CSV File in PowerShell
Appending data to a CSV file in PowerShell can be accomplished using the combination of the ConvertTo-CSV
cmdlet and the Add-Content
cmdlet. This method is useful when you want to add new data to an existing CSV file without overwriting its contents.
By converting data to CSV format and then appending it to the file, you can seamlessly update your CSV files with new information.
Example:
$data = @{
Name = "John Doe"
Age = 30
City = "New York"
}
$obj = New-Object PSObject -Property $data
$csvData = $obj | ConvertTo-Csv -NoTypeInformation
$csvData | Out-File -FilePath "data.csv" -Append
In this example, we first define a hashtable $data
containing information about an individual. We then create a custom object $obj
from this hashtable using the New-Object
cmdlet. Next, we use ConvertTo-CSV
to convert the custom object to CSV format and store the result in the variable $csvData
.
Finally, we use Out-File
with the -Append
parameter to append the CSV data to the file data.csv
. This ensures that the data is appended to the existing file without overwriting its contents.
Output:
Conclusion
In conclusion, appending data to a CSV file in PowerShell is a common requirement for updating existing data without losing any information. We explored three methods to achieve this: using Export-CSV
with the -Append
parameter, employing ConvertTo-CSV
with Add-Content
, and utilizing ConvertTo-CSV
with Out-File
and the -Append
parameter.
Each method offers its own advantages and can be chosen based on specific requirements. To further enhance your PowerShell skills, consider exploring more advanced topics such as manipulating CSV data, handling errors, or automating complex tasks with PowerShell scripting.