How to Convert XML to CSV File in PowerShell
-
Use
Export-Csv
to Convert XML to CSV in PowerShell -
Use
ConvertTo-Csv
to Convert XML to CSV in PowerShell
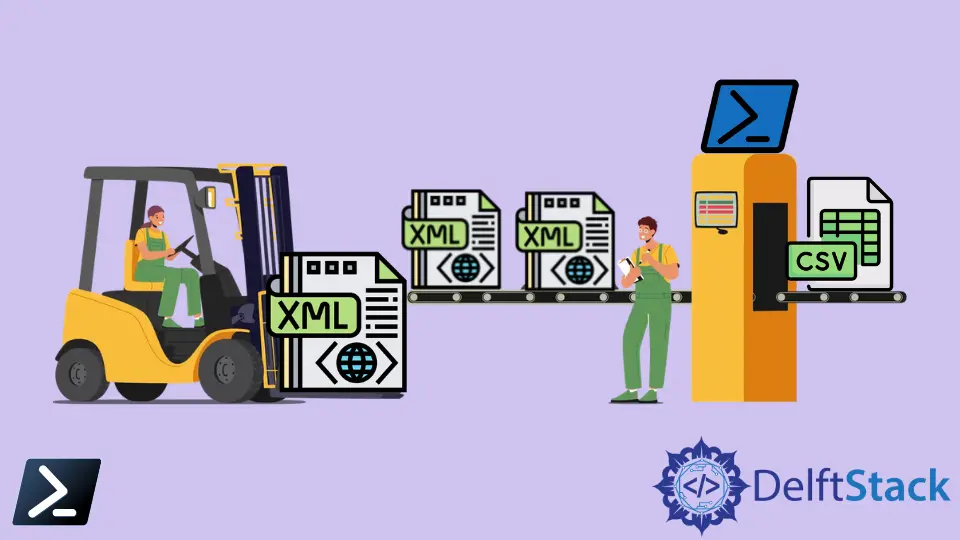
An Extensible Markup Language (XML) file is a plain text file that uses custom tags to define the document’s structure. It is mostly used to store and share structured information.
The content of the XML file looks similar to the HTML file.
A Comma-Separated Values (CSV) file is a delimited text file that allows data to be stored in a tabular format. Each value is separated by the delimiter comma
or any other characters.
This tutorial will teach you to convert an XML file to CSV in PowerShell.
Use Export-Csv
to Convert XML to CSV in PowerShell
The Export-Csv
cmdlet creates a CSV file of the objects. It converts objects into a series of CSV strings and saves them to a file.
We have created an XML file, website.xml
.
<?xml version="1.0" encoding="UTF-8"?>
<website>
<site name="DelftStack">
<content>Programming Tutorials</content>
<topic>PowerShell Howtos</topic>
<article>How to convert XML into CSV?</article>
</site>
<site name="DelftStack">
<content>Programming Tutorials</content>
<topic>Linux Howtos</topic>
<article>Delete Files Recursively in Linux</article>
</site>
</website>
You can use the following command to convert an XML file to a CSV file.
[xml]$inputFile = Get-Content "C:\New\website.xml"
$inputFile.website.ChildNodes | Export-Csv "C:\New\website.csv" -NoTypeInformation -Delimiter:"," -Encoding:UTF8
The Get-Content
cmdlet gets the content of C:\New\website.xml
file. The content objects are sent down the pipeline to the Export-Csv
cmdlet.
The Export-Csv
converts the content objects to a series of CSV strings and saves the strings to a C:\New\website.csv
file. The -Delimiter
parameter specifies a delimiter to separate the property values in CSV strings.
For example, ,
, ;
, :
, etc. The -Encoding
parameter defines the encoding for the exported CSV file.
View the content of C:\New\website.csv
.
Get-Content "C:\New\website.csv"
Output:
"name","content","topic","article"
"DelftStack","Programming Tutorials","PowerShell Howtos","How to convert XML into CSV?"
"DelftStack","Programming Tutorials","Linux Howtos","Delete Files Recursively in Linux"
Use ConvertTo-Csv
to Convert XML to CSV in PowerShell
The ConvertTo-Csv
cmdlet converts objects to CSV strings but does not save the strings to a file. You can use the Set-Content
cmdlet to write CSV strings to a file.
The Set-Content
is a string-processing cmdlet that writes new content or replaces the content in a file.
[xml]$inputFile = Get-Content "C:\New\website.xml"
$inputFile.website.ChildNodes | ConvertTo-Csv -NoTypeInformation -Delimiter ";" | Set-Content "C:\New\new.csv" -Encoding UTF8
The content objects are sent down the pipeline to the ConvertTo-Csv
cmdlet. The ConvertTo-Csv
converts the content objects to a series of CSV strings.
The strings are sent down the pipeline to the Set-Content
cmdlet. The Set-Content
writes the CSV strings to C:\New\new.csv
file.
Get-Content "C:\New\new.csv"
Output:
"name";"content";"topic";"article"
"DelftStack";"Programming Tutorials";"PowerShell Howtos";"How to convert XML into CSV?"
"DelftStack";"Programming Tutorials";"Linux Howtos";"Delete Files Recursively in Linux"
You can view the .csv
file in the table format using MS-Excel or a similar program.
Related Article - PowerShell CSV
- How to Extract a Column From a CSV File and Store It in a Variable in PowerShell
- How to Import Text File and Format and Export It to CSV in PowerShell
- How to Append Data to a CSV File in PowerShell
- How to Combine CSV Files in PowerShell
- How to Import CSV Files Into Array in PowerShell