How to Select Attributes in XML Using XPath
-
Use
Select-Xml
Cmdlet to Select Attributes in XML in PowerShell -
Use the
SelectNodes
Method to Select Attributes in XML in PowerShell -
Use
Get-Content
Cmdlet to Select Attributes in XML in PowerShell
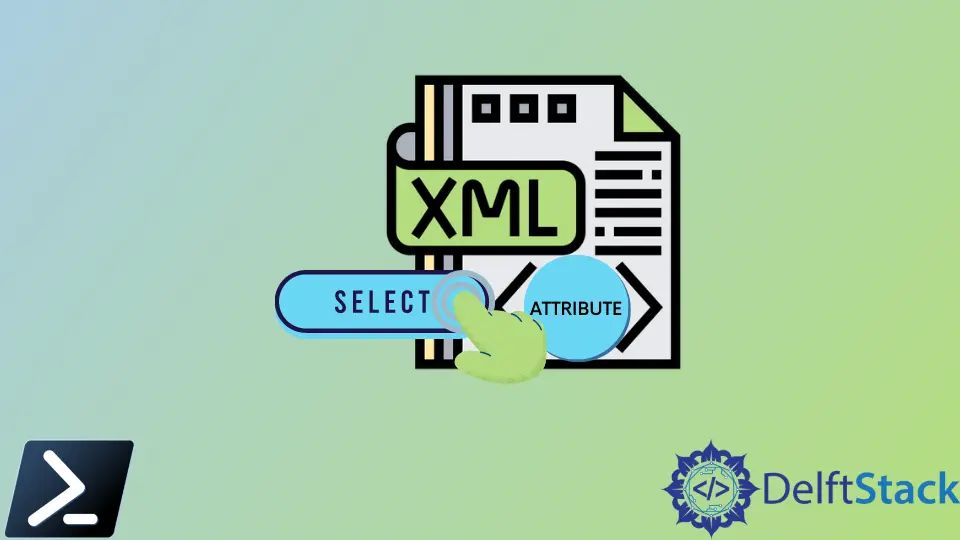
XML stands for Extensible Markup Language. An XML file is a plain text file having custom tags to define the document’s structure.
It is mostly used to store and share structured information. The content of the XML file looks similar to the HTML file.
This tutorial will introduce different methods for selecting attributes in XML using XPath in PowerShell.
Use Select-Xml
Cmdlet to Select Attributes in XML in PowerShell
The Select-Xml
cmdlet lets you find text in an XML string or document using XPath.
This is a simple example of an XML file.
<website>
<site name="DelftStack">
<content>
<topic>PowerShell Howtos</topic>
<article>How to convert XML into CSV?</article>
</content>
</site>
<site name="DelftStack">
<content>
<topic>Linux Howtos</topic>
<article>Delete Files Recursively in Linux</article>
</content>
</site>
</website>
The following command searches for the XPath query topic
in a new.xml
file in the C:\Users\rhntm
directory.
Select-Xml -Path C:\Users\rhntm\new.xml -XPath "//topic" | Select-Object -ExpandProperty Node
Output:
#text
-----
PowerShell Howtos
Linux Howtos
The -Path
parameter specifies the path and file names of the XML files to search.
The -XPath
parameter specifies an XPath query to search. The query text is case-sensitive.
Use the SelectNodes
Method to Select Attributes in XML in PowerShell
The SelectNodes()
method selects a list of nodes matching the XPath expression in an XML file.
The following example selects the node content
from a new.xml
file.
[xml]$xml = Get-Content 'C:\Users\rhntm\new.xml'
$xml.SelectNodes('//content')
Output:
topic article
----- -------
PowerShell Howtos How to convert XML into CSV?
Linux Howtos Delete Files Recursively in Linux
The [xml]
casts the variable as an XML object. The Get-Content
cmdlet gets the content of a C:\Users\rhntm\new.xml
and saves it in the $xml
variable.
To select the specific attribute, you can use the Select-Object
cmdlet. For example, the following command selects only the article
from a new.xml
file.
[xml]$xml = Get-Content 'C:\Users\rhntm\new.xml'
$xml.SelectNodes('//content') | Select-Object article
Output:
article
-------
How to convert XML into CSV?
Delete Files Recursively in Linux
Use Get-Content
Cmdlet to Select Attributes in XML in PowerShell
As you have seen above, the Get-Content
cmdlet gets the content of an XML file. You can save the content in a variable and cast it as an XML object.
The following example shows how to use Get-Content
and select attributes in an XML file.
[xml]$xml = Get-Content 'C:\Users\rhntm\new.xml'
$xml.website.site.content.topic
Output:
PowerShell Howtos
Linux Howtos
This is an easy way to use if you want the text value from the XML file. We hope this helped you with how to select attributes in XML in PowerShell.