How to Box Plot in Plotly
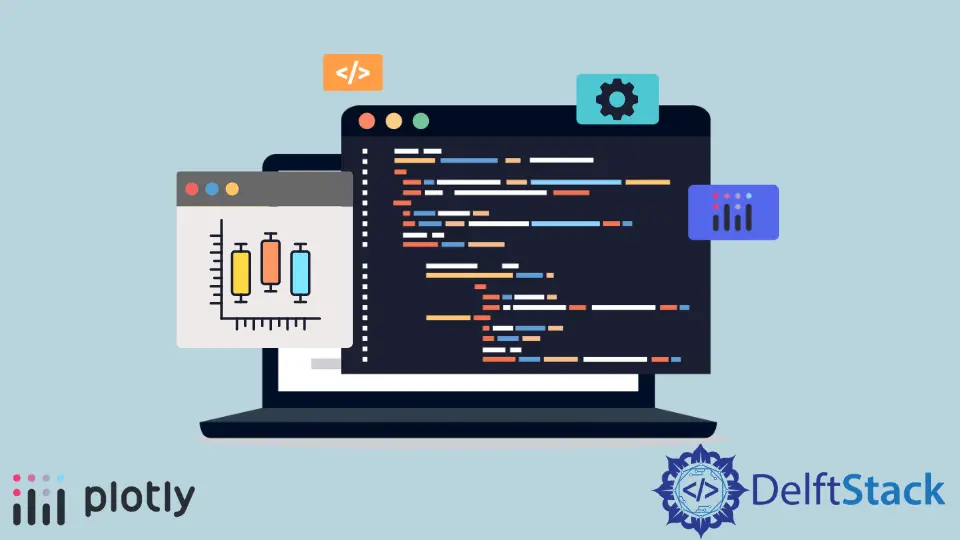
This tutorial will discuss creating a box plot using the box()
function of Plotly.
Plotly Box Plot
A box plot in Plotly represents the distribution of a variable through its quartiles. The ends of the box describe the lower and upper quartiles, while a line inside the box marks the median or second quartile.
We can use the box()
function of Plotly to create a box plot of the given data. We must provide the data frame or values and labels inside the box()
function to create the box plot.
The code below creates a box plot of some random data.
import plotly.express as px
labels = ["A", "B", "C"]
value = [[10, 50, 30], [20, 30, 60], [10, 20, 30], [10, 10, 10]]
fig = px.box(x=labels, y=value, width=500, height=400)
fig.show()
Output:
The width
and height
argument is used to set the width and height of the figure in pixels. We can give each cell a default color using the color
argument and set its value to labels.
We can set the boxes’ orientation using the orientation
argument and a string h
for horizontal and v
for vertical orientation. We can set the axis to log scale using the log_x
for the x_axis and log_y
for the y-axis and set its value to true.
We can set the shape of the boxes to notched using the notched
argument and set its value to true. We can give the graph a title using the title
argument.
We can use the facet_col
and facet_row
to create subplots with separate cells and set their value to a list of integers or strings to set the title for the subplot. We can set the distance between two subplots using the facet_col_spacing
for column spacing and facet_row_spacing
for row spacing.
The code below changes the arguments mentioned above.
import plotly.express as px
labels = ["A", "B", "C"]
value = [[10, 50, 30], [20, 30, 60], [10, 20, 30], [10, 10, 10]]
fig = px.box(
x=labels,
y=value,
width=700,
height=400,
color=labels,
notched=True,
title="Plotly Box Plot",
facet_col=[1, 2, "b"],
)
fig.show()
Output:
By default, the box()
function gives each cell a different color, but we can set each cell’s color using the color
and color_discrete_map
arguments. We must pass each cell label inside the color argument and then give each label a color using the color_discrete_map
argument to change the color of each cell. If we don’t define the color for a label, the box()
function automatically gives it a random color.
The code below sets the color of each cell.
import plotly.express as px
labels = ["A", "B", "C"]
value = [[10, 50, 30], [20, 30, 60], [10, 20, 30], [10, 10, 10]]
fig = px.box(
x=labels,
y=value,
width=700,
height=400,
color=labels,
color_discrete_map={
"A": "green",
"B": "cyan",
"C": "yellow",
},
)
fig.show()
Output:
We can change the default color scale or sequence to set the color of each cell using the color_discrete_sequence
argument. The argument’s value should be a list of valid CSS colors. We can use Plotly’s built-in color sequence like the Vivid
, Light24
, and Dark2
.
The code below changes the pie chart’s colors using the Dark2
color sequence.
import plotly.express as px
labels = ["A", "B", "C"]
value = [[10, 50, 30], [20, 30, 60], [10, 20, 30], [10, 10, 10]]
fig = px.box(
x=labels,
y=value,
width=700,
height=400,
color=labels,
color_discrete_sequence=px.colors.qualitative.Dark2,
)
fig.show()
Output:
To change the color sequence in the code, we must change the color sequence’s name from Dark2
to Vivid
. Visit this link for more details about Plotly’s color sequence.
We can update traces of the bar()
function using the fig.update_traces()
function. We can change the fill color of boxes using the fillcolor
argument and set its value to a color name like yellow. We can change the boxes’ opacity using the opacity
argument and set its value from 0 to 1.
We can set the legend’s title group using the legendgrouptitle_text
argument and set its value to a string.
The code below changes the traces mentioned above using the fig.update_traces()
function.
import plotly.express as px
labels = ["A", "B", "C"]
value = [[10, 50, 30], [20, 30, 60], [10, 20, 30], [10, 10, 10]]
fig = px.box(x=labels, y=value, width=700, height=400, color=labels)
fig.update_traces(fillcolor="yellow", opacity=0.8, legendgrouptitle_text="LegendTitle")
fig.show()
Output:
Visit this link for more details about the box()
function traces.