How to Plot 3D Scatter Plot in Plotly
-
Use the
scatter_3d()
Function of Plotly to Create a 3D Scatter Plot in Python - Change Color and Traces of the 3D Scatter Plot in Python
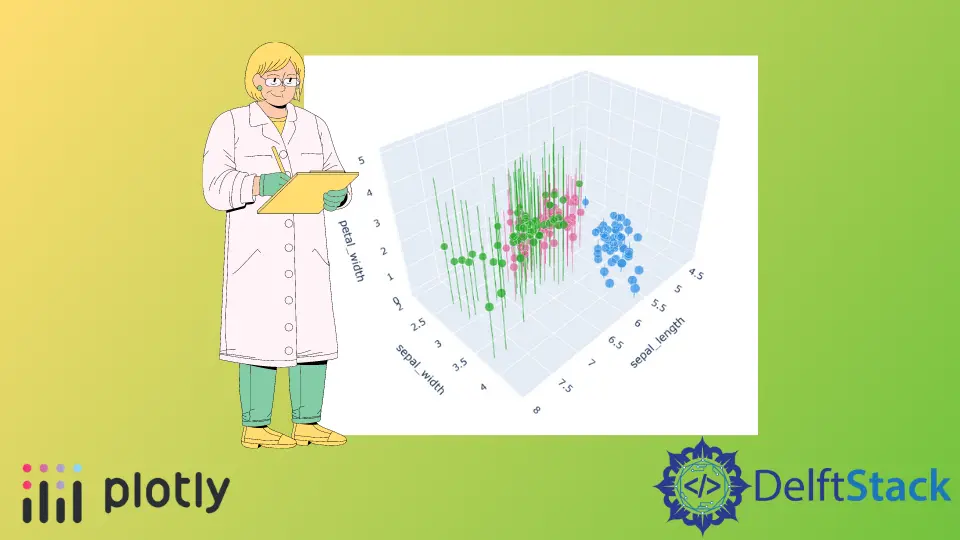
This tutorial will discuss creating a 3D scatter plot using the scatter_3d()
function of Plotly in Python.
Use the scatter_3d()
Function of Plotly to Create a 3D Scatter Plot in Python
A scatter plot shows data points as circles or bubbles on a graph. To create a 3D scatter plot, we can use the scatter_3d()
function of plotly.express
.
To create the 3D scatter plot, we must pass the x, y, and z-axis values inside the scatter_3d()
function. If only one axis value is given, the function will use the indices of the values as the second and third axis values.
For example, let’s create a 3D scatter plot using the iris
data set already present in Plotly. See the code below.
import plotly.express as px
df = px.data.iris()
fig = px.scatter_3d(
df, x="sepal_length", y="sepal_width", z="petal_width", color="species"
)
fig.show()
Output:
The width
and height
arguments are used to set the width and height of the above figure in pixels. We can also create a 3D scatter plot using a data frame.
In the case of a data frame, we can use the data_frame
argument to pass the data inside the scatter_3d()
function. We can give random color to each bubble using the color
argument.
The scatter_3d()
function will use a color sequence to set the color of each bubble. We can set the size of each bubble using the size
argument and set its value to a list of integers or a data frame column.
We can set the hover title for each bubble using the hover_name
argument and set its value to a list of strings or a data frame column.
We can also add text inside each bubble using the text
argument and set its value to a list of strings or a data frame column.
For example, let’s change the properties mentioned above. See the code below.
import plotly.express as px
df = px.data.iris()
fig = px.scatter_3d(
df,
x="sepal_length",
y="sepal_width",
z="petal_width",
color="species",
size="sepal_width",
hover_name="species",
)
fig.show()
Output:
Change Color and Traces of the 3D Scatter Plot in Python
We can also add error bars on each bubble using the error_x
for the x-axis error, error_y
for the y-axis error, and error_z
for the z-axis error.
We can change the default color sequence using the color_discrete_sequence
and set its value to a Plotly supported color sequence like Dark24
and Light24
using the plotly.express.color.qualitative
attribute.
For example, let’s change the color sequence of the above 3D scatter plot. See the code below.
import plotly.express as px
df = px.data.iris()
fig = px.scatter_3d(
df,
x="sepal_length",
y="sepal_width",
z="petal_width",
color="species",
size="sepal_width",
error_z="petal_width",
color_discrete_sequence=px.colors.qualitative.Dark24,
)
fig.show()
Output:
To change the color sequence, we need to change the color sequence name in the above code, like from Dark24
to Light24
. If we want to give each bubble our color instead of a color sequence, we can use the color_discrete_map
argument to override the color sequence.
Check this link for more details about the Plotly colors.
For example, let’s change the color of the first two bubbles sequences present in the above plot. See the code below.
import plotly.express as px
df = px.data.iris()
fig = px.scatter_3d(
df,
x="sepal_length",
y="sepal_width",
z="petal_width",
color="species",
color_discrete_map={"setosa": "red", "versicolor": "green"},
)
fig.show()
Output:
We only changed the first two colors, and the rest of the colors will be from the default color sequence. We can set the opacity of the bubbles using the opacity
argument and set its value from 0 to 1.
We can also change the axis to log scale using the log_x
, log_y
, and log_z
arguments and set its value to true. We can set the title of the figure using the title
argument.
We can also change many other properties of the 3D scatter plot using the scatter_3d()
function and traces using the fig.update_traces()
function. We can hide the legends using the showlegend
argument and set its value to false.
We can change the color of the error bars, which is by default the same as the bubble color using the error_y_color
argument.
For example, let’s change the traces mentioned above. See the code below.
import plotly.express as px
df = px.data.iris()
fig = px.scatter_3d(
df,
x="sepal_length",
y="sepal_width",
z="petal_width",
color="species",
error_z="petal_width",
)
fig.update_traces(showlegend=False, error_z_color="red")
fig.show()
Output:
Check this link for more details about the scatter_3d()
function traces.