How to Compare Strings Using == Operator and STRCMP Function in PHP
-
Use the PHP
==
Operator to Compare Strings in PHP -
Use the PHP
===
Operator to Compare Variables With String Values and Integer Values -
Use the
strcmp()
Function to Compare Strings in PHP
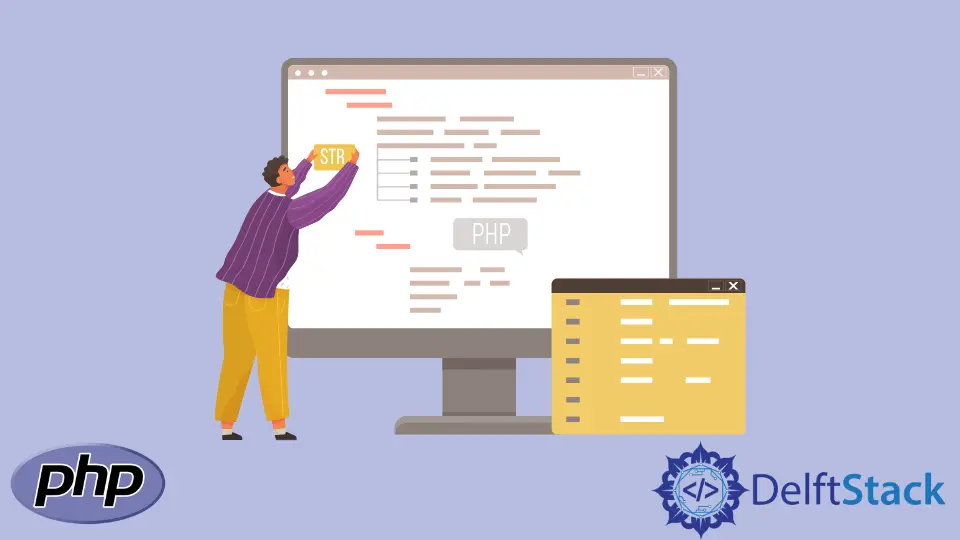
The equal operator ==
use to compare the values of variables and the identical operator ===
to compare variables with string values and integer values.
Then, we will introduce the strcmp()
function and compare strings.
Use the PHP ==
Operator to Compare Strings in PHP
The PHP ==
operator, also known as the Equal Operator, can compare the values of variables. The operator returns false if the values are not the same.
In the example below, we will compare the currencies.
<?php
// Our Currencies
$value1 = "USD";
$value2 = "EUR";
// Use the == to compare our currencies
if ($value1 == $value2) {
echo 'The Currencies are the same';
}
else {
echo 'The Currencies are not the same;
}
?>
Output:
The Currencies are not the same
The comparison above is correct since the USD
is not the same as EUR
.
Use the PHP ===
Operator to Compare Variables With String Values and Integer Values
It would be best if you avoided the ==
operator to compare variables of string values and integer values.
Here is why;
In the example code below, we will use the ==
operator to compare a variable string value 290
with an integer value 290
.
<?php
// Our variable with an Integer value
$value1 = 290;
// Our variable with a String value
$value2 = "290";
// Compare $value1 with value2 using the == operator
if ($value1 == $value2) {
echo 'The values are the same';
}
else {
echo 'The values are not the same;
}
?>
Output:
The values are the same
Technically, the output above is incorrect since the different data types.
Let us look at the correct code.
<?php
// Our variable with an Integer value
$value1 = 290;
// Our variable with a String value
$value2 = "290";
// Compare $value1 with value2 using the === operator
if ($value1 === $value2) {
echo 'The values are the same';
}
else {
echo 'The values are not the same;
}
?>
Output:
The values are not the same
The above output is true since our values are of different data types.
The ===
operator returns true when both variables have the same information and data types.
Use the strcmp()
Function to Compare Strings in PHP
The strcmp()
function can compare strings and show if the second string is greater, smaller, or equal to the first string.
In the example code below, we will compare;
- The first name of an employee with the first name of the same employee.
- The first and second name with the first name.
- The first name with the first, second, and surname.
Example code:
<?php
echo strcmp("Joyce","Joyce")."<br>"; // (Equal strings) First name with First name
echo strcmp("Joyce Lynn", "Joyce")."<br>"; // String1 greater than string2
echo strcmp("Joyce", "Joyce Lynn Lee")."<br>"; // String1 less than String 2
?>
Output:
0
5
-9
The output will always be =0
when both strings are equal.
When String1
is greater than String2
, the output will be a random value greater than 0
.
When String1
is less than String2
, the output will be a random value less than <0
.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedInRelated Article - PHP String
- How to Remove All Spaces Out of a String in PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert an Integer Into a String in PHP
- How to Convert an Array to a String in PHP
- How to Convert a String to a Number in PHP