在 PHP 中使用 == 运算符和 STRCMP 函数进行字符串比较
John Wachira
2023年1月30日
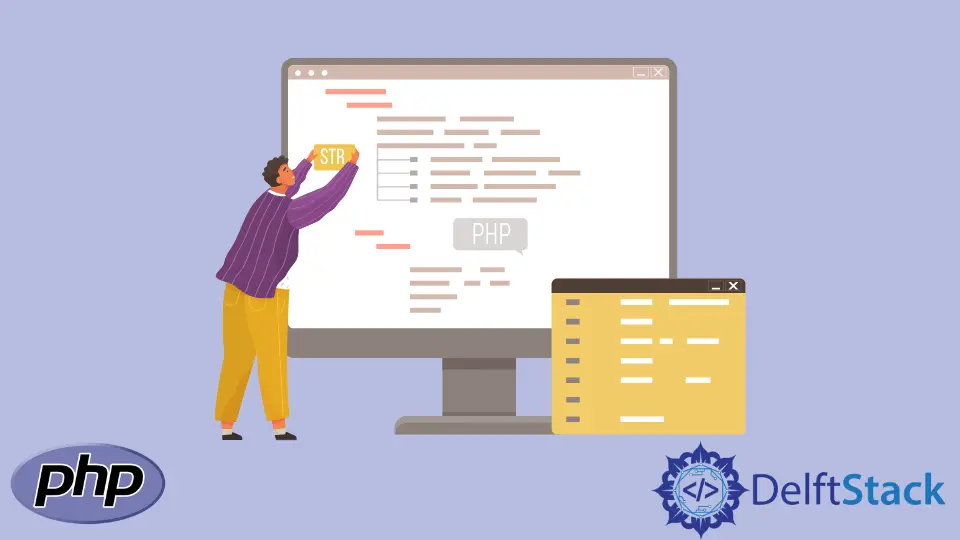
等号运算符 ==
用于比较变量的值,而相同的运算符 ===
用于比较变量与字符串值和整数值。
然后,我们将介绍 strcmp()
函数并比较字符串。
使用 PHP ==
运算符比较 PHP 中的字符串
PHP ==
运算符,也称为相等运算符,可以比较变量的值。如果值不同,则运算符返回 false。
在下面的示例中,我们将比较货币。
<?php
// Our Currencies
$value1 = "USD";
$value2 = "EUR";
// Use the == to compare our currencies
if ($value1 == $value2) {
echo 'The Currencies are the same';
}
else {
echo 'The Currencies are not the same;
}
?>
输出:
The Currencies are not the same
上面的比较是正确的,因为 USD
与 EUR
不同。
使用 PHP ===
运算符将变量与字符串值和整数值进行比较
最好避免使用 ==
运算符来比较字符串值和整数值的变量。
这就是为什么;
在下面的示例代码中,我们将使用 ==
运算符将变量字符串值 290
与整数值 290
进行比较。
<?php
// Our variable with an Integer value
$value1 = 290;
// Our variable with a String value
$value2 = "290";
// Compare $value1 with value2 using the == operator
if ($value1 == $value2) {
echo 'The values are the same';
}
else {
echo 'The values are not the same;
}
?>
输出:
The values are the same
从技术上讲,上面的输出是不正确的,因为数据类型不同。
让我们看看正确的代码。
<?php
// Our variable with an Integer value
$value1 = 290;
// Our variable with a String value
$value2 = "290";
// Compare $value1 with value2 using the === operator
if ($value1 === $value2) {
echo 'The values are the same';
}
else {
echo 'The values are not the same;
}
?>
输出:
The values are not the same
上述输出为真,因为我们的值属于不同的数据类型。
当两个变量具有相同的信息和数据类型时,===
运算符返回 true。
在 PHP 中使用 strcmp()
函数比较字符串
strcmp()
函数可以比较字符串并显示第二个字符串是否大于、小于或等于第一个字符串。
在下面的示例代码中,我们将进行比较;
- 员工的名字与同一员工的名字。
- 第一个和第二个名字与名字。
- 第一个名字,第二个和姓氏。
示例代码:
<?php
echo strcmp("Joyce","Joyce")."<br>"; // (Equal strings) First name with First name
echo strcmp("Joyce Lynn", "Joyce")."<br>"; // String1 greater than string2
echo strcmp("Joyce", "Joyce Lynn Lee")."<br>"; // String1 less than String 2
?>
输出:
0
5
-9
当两个字符串相等时,输出将始终为 =0
。
当 String1
大于 String2
时,输出将是一个随机值大于 0
。
当 String1
小于 String2
时,输出将是一个随机值小于<0
。
作者: John Wachira
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn相关文章 - PHP String
- 如何在 PHP 中删除字符串中的所有空格
- 如何在 PHP 中将 DateTime 转换为字符串
- 如何在 PHP 中将字符串转换为日期和日期时间
- 如何在 PHP 中将整数转换为字符串
- 如何在 PHP 中将数组转换为字符串
- 如何在 PHP 中将字符串转换为数字