如何在 PHP 中将字符串转换为数字
Minahil Noor
2023年1月30日
PHP
PHP String
PHP Number
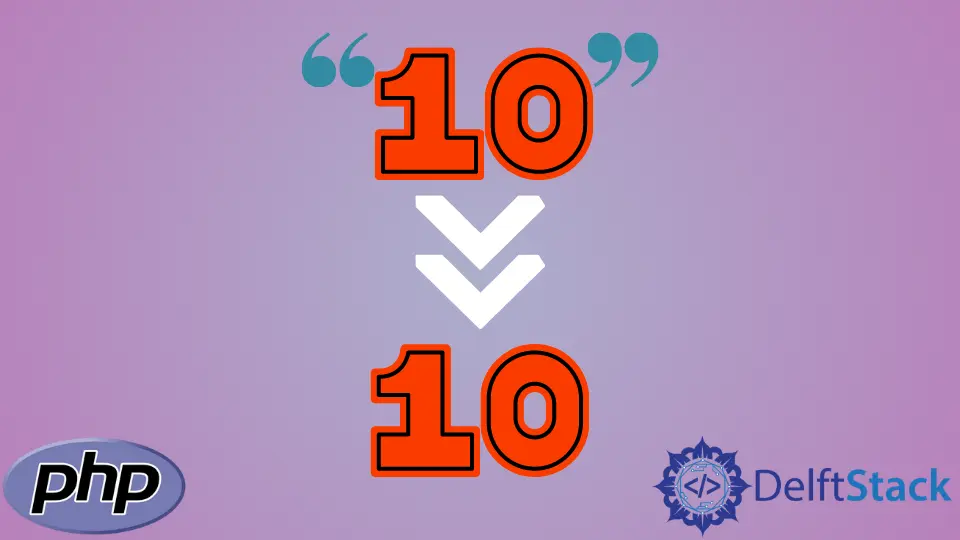
在本文中,我们将介绍在 PHP 中将字符串转换为数字的方法。
- 使用显式类型转换
- 使用
intval()
/floatval()
函数 - 使用
settype()
函数
在 PHP 中使用显式类型转换将字符串转换为数字
显式类型转换将任何类型的变量转换为所需类型的变量。它将变量转换为原始数据类型。将变量显式类型转换为 int
或 float
的正确语法如下
$variableName = (int)$stringName
$variableName = (float)$stringName
变量 $variableName
是整数变量,用于保存 number
的值。变量 $stringName
是数字字符串。我们还可以将非数字字符串转换为数字。
<?php
$variable = "10";
$integer = (int)$variable;
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "8.1";
$float = (float)$variable;
echo "The variable $variable has converted to a number and its value is $float.";
?>
输出:
The variable 10 has converted to a number and its value is 10.
The variable 8.1 has converted to a number and its value is 8.1.
对于非数字字符串,我们将得到 0 作为输出。
<?php
$variable = "abc";
$integer = (int)$variable;
echo "The variable has converted to a number and its value is $integer.";
?>
输出:
The variable has converted to a number and its value is 0.
在 PHP 中使用 intval()
函数将字符串转换为数字
intval()
函数将字符串转换为整型 int
。
floatval()
函数将字符串转换为浮点数 float
。
这些函数接受的参数是一个字符串,返回值分别是一个整数或一个浮点数。
intval( $StringName );
变量 StringName
是字符串类型。
<?php
$variable = "53";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
输出:
The variable 53 has converted to a number and its value is 53.
The variable 25.3 has converted to a number and its value is 25.3.
如果给定的字符串是数字和字符串表示形式的混合,例如 2020Time
,则 intval()
将返回 2020
。但是,如果给定的字符串不是以数字开头,则它将返回 0
。floatval()
方法也是如此。
示例代码:
<?php
$variable = "2020Time";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "Time2020";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3Time";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
echo "\n";
$variable = "Time25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
输出:
The variable 2020Time has converted to a number and its value is 2020.
The variable Time2020 has converted to a number and its value is 0.
The variable 25.3Time has converted to a number and its value is 25.3.
The variable Time25.3 has converted to a number and its value is 0.
在 PHP 中使用 settype()
函数将字符串转换为数字
settype()
函数将任何数据类型的变量转换为特定数据类型。它接受两个参数,变量和类型名称。
settype($variableName, "typeName");
$variableName
是我们要转换为数字的任何数据类型的变量。"typeName"
是我们要为 $variableName
变量使用的数据类型的名称。
<?php
$variable = "45";
settype($variable, "integer");
echo "The variable has converted to a number and its value is $variable.";
?>
settype()
函数将 $variable
的类型从字符串设置为整型 integer
。它仅适用于原始数据类型。
输出:
The variable has converted to a number and its value is 45.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
相关文章 - PHP String
- 如何在 PHP 中删除字符串中的所有空格
- 如何在 PHP 中将 DateTime 转换为字符串
- 如何在 PHP 中将字符串转换为日期和日期时间
- 如何在 PHP 中将整数转换为字符串
- 如何在 PHP 中将数组转换为字符串