Como converter uma string para um número em PHP
- Use Fundição do tipo explícita para converter uma string em um número em PHP
-
Utilize
intval()
Função para converter uma String para um número em PHP -
Utilize
settype()
Função para Converter uma String para um Número em PHP
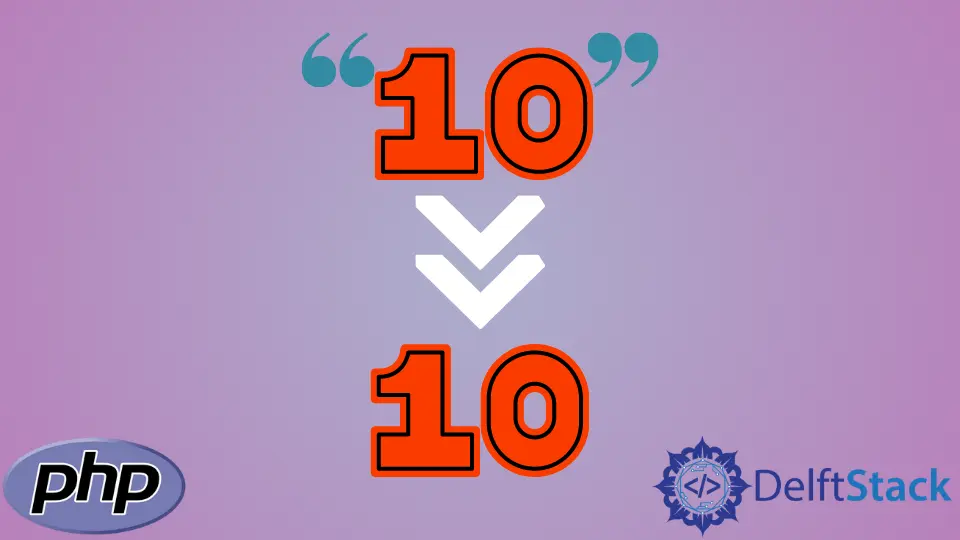
Neste artigo, vamos introduzir métodos para converter uma string para um número em PHP.
- Usando a função de tipo explícito
- Utilizando a função
intval()
/floatval()
- Utilizando a função
settype()
Use Fundição do tipo explícita para converter uma string em um número em PHP
A fundição do tipo explícito converte uma variável de qualquer tipo em uma variável do tipo requerido. Converte uma variável em tipos de dados primitivos. A sintaxe correta para digitar explicitamente uma variável para um int
ou um float
é a seguinte
$variableName = (int)$stringName
$variableName = (float)$stringName
A variável $variableName
é a variável inteira para manter o valor do número. A variável $stringName
é a variável numérica string
. Também podemos converter strings não-numéricas em um número.
<?php
$variable = "10";
$integer = (int)$variable;
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "8.1";
$float = (float)$variable;
echo "The variable $variable has converted to a number and its value is $float.";
?>
Resultado:
The variable 10 has converted to a number and its value is 10.
The variable 8.1 has converted to a number and its value is 8.1.
Para strings não-numéricas, obteremos um 0 como saída.
<?php
$variable = "abc";
$integer = (int)$variable;
echo "The variable has converted to a number and its value is $integer.";
?>
Resultado:
The variable has converted to a number and its value is 0.
Utilize intval()
Função para converter uma String para um número em PHP
A função intval()
converte um string
em um int
.
A função floatval()
converte uma string
em uma float
.
O parâmetro que essas funções aceitam é um string
e o valor de retorno é um inteiro ou um número flutuante, respectivamente.
intval( $StringName );
A variável StringName
contém o valor da string
.
<?php
$variable = "53";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
Resultado:
The variable 53 has converted to a number and its value is 53.
The variable 25.3 has converted to a number and its value is 25.3.
A string dada é uma mistura do número e da representação da string, como 2020Time
, intval()
retornará 2020
. Mas se a string dada não começar com um número, então ela retorna 0
. Assim é o método floatval()
.
Códigos de exemplo:
<?php
$variable = "2020Time";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "Time2020";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3Time";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
echo "\n";
$variable = "Time25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
Resultado:
The variable 2020Time has converted to a number and its value is 2020.
The variable Time2020 has converted to a number and its value is 0.
The variable 25.3Time has converted to a number and its value is 25.3.
The variable Time25.3 has converted to a number and its value is 0.
Utilize settype()
Função para Converter uma String para um Número em PHP
A função settype()
converte a variável de qualquer tipo de dado em um determinado tipo de dado. Ela aceita dois parâmetros, a variável e o nome do tipo.
settype($variableName, "typeName");
A função $variableName
é a variável de qualquer tipo de dado que queremos converter para um número. O "typeName"
contém o nome do tipo de dado que queremos para nossa variável $variableName
.
<?php
$variable = "45";
settype($variable, "integer");
echo "The variable has converted to a number and its value is $variable.";
?>
A função settype()
definiu o tipo de $variable
de string
para integer. Ela só é adequada para os tipos de dados primitivos.
Resultado:
The variable has converted to a number and its value is 45.
Artigo relacionado - PHP String
- Como remover todos os espaços fora de uma string em PHP
- Como Converter DataTime em String em PHP
- Como converter String para Data e Data/Hora em PHP
- Como converter um número inteiro em uma string em PHP
- Como Converter um Array em uma string em PHP