Converti una stringa in un numero in PHP
- Usa il cast di tipo esplicito per convertire una stringa in un numero in PHP
-
Usa la funzione
intval()
per convertire una stringa in un numero in PHP -
Usa la funzione
settype()
per convertire una stringa in un numero in PHP
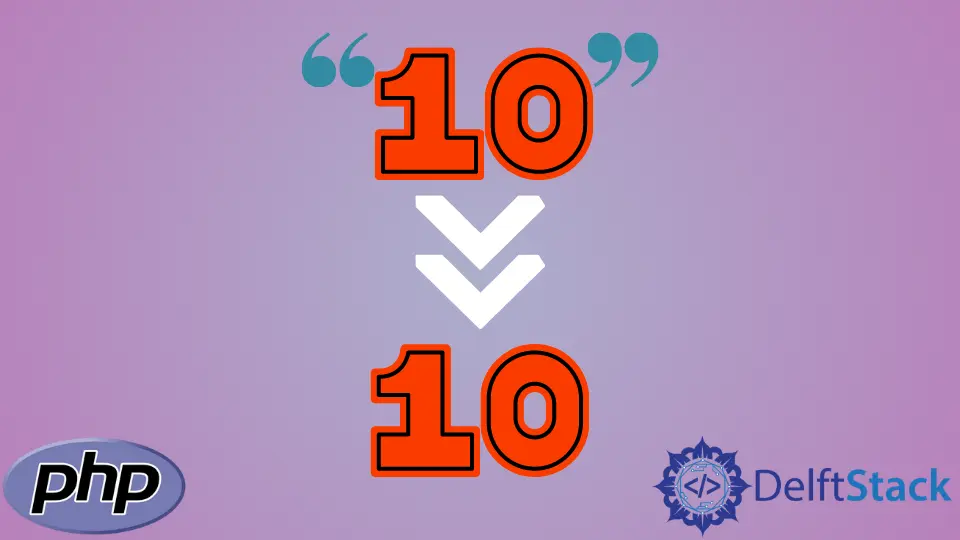
In questo articolo, introdurremo metodi per convertire una stringa in un numero in PHP.
- Utilizzo di casting di tipo esplicito
- Utilizzando la funzione
intval()
/floatval()
- Utilizzo della funzione
settype()
Usa il cast di tipo esplicito per convertire una stringa in un numero in PHP
Il casting esplicito del tipo converte una variabile di qualsiasi tipo in una variabile del tipo richiesto. Converte una variabile in tipi di dati primitivi. La sintassi corretta per tipizzare esplicitamente una variabile in un int
o in un float
è la seguente
$variableName = (int)$stringName
$variableName = (float)$stringName
La variabile $variableName
è la variabile intera che contiene il valore del numero. La variabile $stringName
è la stringa
numerica. Possiamo anche convertire stringhe non numeriche in un numero.
<?php
$variable = "10";
$integer = (int)$variable;
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "8.1";
$float = (float)$variable;
echo "The variable $variable has converted to a number and its value is $float.";
?>
Produzione:
The variable 10 has converted to a number and its value is 10.
The variable 8.1 has converted to a number and its value is 8.1.
Per una stringa non numerica, otterremo uno 0 come output.
<?php
$variable = "abc";
$integer = (int)$variable;
echo "The variable has converted to a number and its value is $integer.";
?>
Produzione:
The variable has converted to a number and its value is 0.
Usa la funzione intval()
per convertire una stringa in un numero in PHP
La funzione intval()
converte una stringa
in un int
.
La funzione floatval()
converte una stringa
in un float
.
Il parametro che queste funzioni accettano è una stringa
e il valore di ritorno è rispettivamente un intero o un numero mobile.
intval( $StringName );
La variabile StringName
contiene il valore della stringa
.
<?php
$variable = "53";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
Produzione:
The variable 53 has converted to a number and its value is 53.
The variable 25.3 has converted to a number and its value is 25.3.
Se la stringa data è una miscela del numero e della rappresentazione della stringa, come 2020Time
, intval()
restituirà 2020
. Ma se la stringa data non inizia con un numero, restituisce 0
. Così è il metodo floatval()
.
Codici di esempio:
<?php
$variable = "2020Time";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "Time2020";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3Time";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
echo "\n";
$variable = "Time25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
Produzione:
The variable 2020Time has converted to a number and its value is 2020.
The variable Time2020 has converted to a number and its value is 0.
The variable 25.3Time has converted to a number and its value is 25.3.
The variable Time25.3 has converted to a number and its value is 0.
Usa la funzione settype()
per convertire una stringa in un numero in PHP
La funzione settype()
converte la variabile di qualsiasi tipo di dato in un particolare tipo di dato. Accetta due parametri, la variabile e il nome del tipo.
settype($variableName, "typeName");
Il $variableName
è la variabile di qualsiasi tipo di dato che vogliamo convertire in un numero. Il "typeName"
contiene il nome del tipo di dati che vogliamo per la nostra variabile $variableName
.
<?php
$variable = "45";
settype($variable, "integer");
echo "The variable has converted to a number and its value is $variable.";
?>
La funzione settype()
ha impostato il tipo di $variabile
da stringa
a intero. È adatto solo per tipi di dati primitivi.
Produzione:
The variable has converted to a number and its value is 45.
Articolo correlato - PHP String
- Rimuovi tutti gli spazi da una stringa in PHP
- Converti DateTime in String in PHP
- Converti la stringa in data e data-ora in PHP
- Converti un intero in una stringa in PHP
- Converti un array in una stringa in PHP