Question Mark Symbol in PHP
- Introduction to Ternary Operator in PHP
-
Evaluating
True
andFalse
Expression Using Ternary Operator in PHP
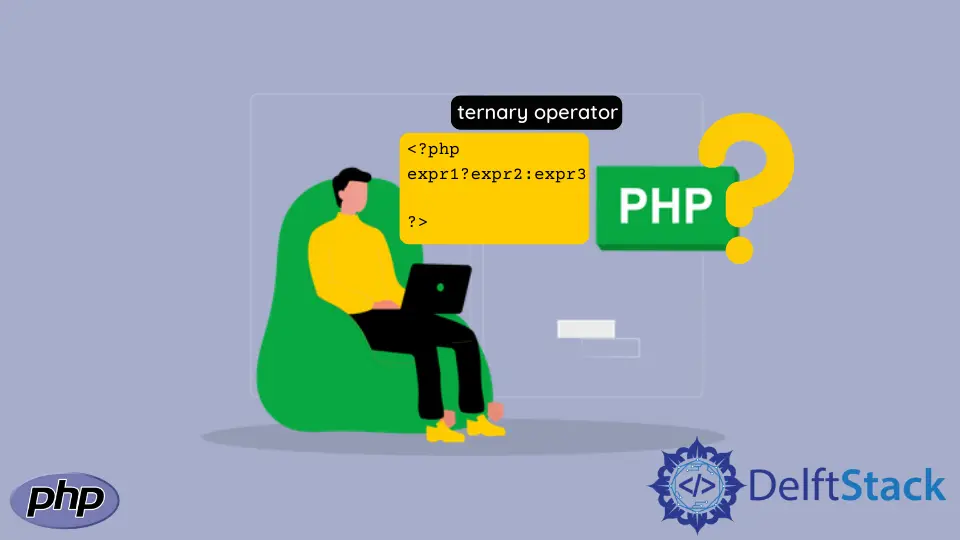
In PHP, The question mark ?
operator is called the ternary operator or conditional ternary operator. This article will go in-depth into the ternary operator in PHP.
Introduction to Ternary Operator in PHP
The ternary conditional operator ?
is also known as the conditional expression or ternary operator. It has the following syntax:
$variable = (condition) ? value_if_true : value_if_false;
Here’s a breakdown of the components:
-
condition
: A Boolean expression that is evaluated. -
value_if_true
: The value assigned to$variable
ifcondition
is true. -
value_if_false
: The value assigned to$variable
ifcondition
is false.
The ternary operator evaluates condition
; if it’s true, it assigns value_if_true
to $variable
; otherwise, it assigns value_if_false
.
Example Code:
<?php
$num = 10;
$result = ($num % 2 == 0) ? "Even" : "Odd";
echo "The number $num is $result.";
?>
Output:
The number 10 is Even.
We can use the ternary operator to substitute for if-else
constructs to shorten the code’s length and improve the readability. We can use it to write simpler conditional expressions.
Example Code:
<?php
$count = (true) ? 1 : 0;
if (true)
{
$count = 1;
}
else
{
$count = 0;
}
echo $count;
?>
Output:
1
However, reading the code when we chain or nest the ternary operator may take work. Another downside of the ternary operator is that it is hard to debug the code as we cannot place breakpoints in the subexpressions.
Evaluating True
and False
Expression Using Ternary Operator in PHP
We will look into the usage of the ternary operator. The example below will evaluate the true
expression and execute the code block accordingly.
The canVote()
function will determine if a person can cast a vote or not. First, the variable $age
is evaluated if greater than or equal to 18
.
Next, we invoked the canVote()
function with the value of 21
as the parameter, which is the legal age of voting. Here, the expression is evaluated as true
, and the second is executed.
Example Code:
<?php
function canVote($age){
echo $age>=18 ?"you can vote": "you cannot vote";
}
canVote(21);
?>
The canVote()
function will determine if a person can cast a vote or not. First, the variable $age
is evaluated if greater than or equal to 18
.
Next, we invoked the canVote()
function with the value of 21
as the parameter, which is the legal age of voting. Here, the expression is evaluated as true
, and the second is executed.
Output:
you can vote
In the next example, If we supply a value less than 18, the comparison expression will be evaluated as false
. Hence, the third expression, you cannot vote
, will be displayed.
Example Code:
<?php
function canVote($age){
echo $age>=18 ?"you can vote": "you cannot vote";
}
canVote(17);
?>
Output:
you cannot vote
In other cases, the evaluation to be expressed will return a false
value. For instance, if the expression returns a null
value, Nan
, 0
, or an empty value, the expression will return a false
value.
The following example shows the conditions of the falsy
value. The ternary operator will execute the third expression when these values are evaluated.
Example Code:
<?php
function Car($name){
echo $name?$name:"no name";
echo "<br>";
}
Car("Tesla");
Car(null);
Car("");
Car(0);
?>
Output:
Tesla
no name
no name
no name
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn