PHP Spaceship Operator
- What is the Spaceship Operator?
- Basic Usage of the Spaceship Operator
- Sorting Arrays with the Spaceship Operator
- Comparing Strings with the Spaceship Operator
- Handling Null Values with the Spaceship Operator
- Conclusion
- FAQ
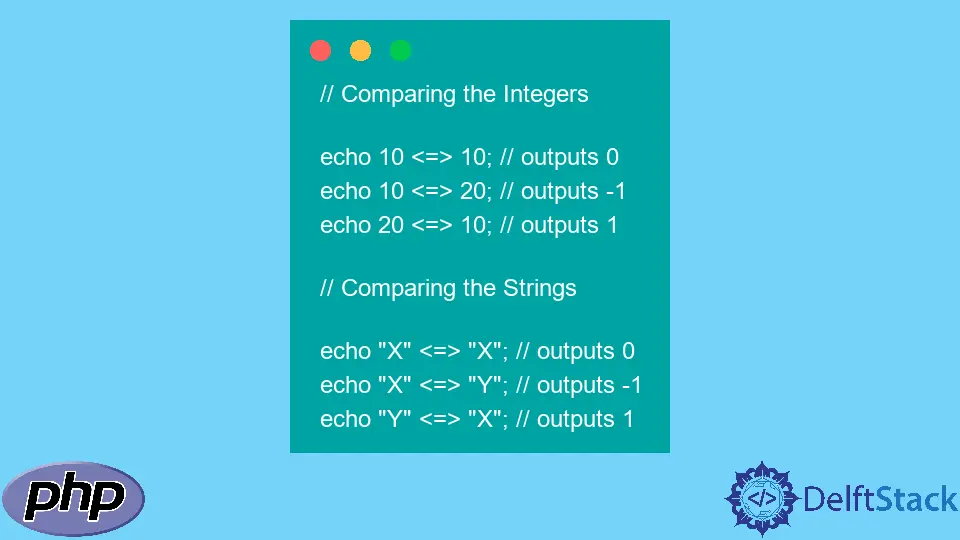
The PHP spaceship operator, introduced in PHP 7, is a powerful tool for developers looking to simplify their code when comparing values. This operator, represented as <=>
, provides a streamlined way to compare two expressions, returning -1, 0, or 1 depending on whether the left operand is less than, equal to, or greater than the right operand.
In this tutorial, we will dive deep into the functionality of the spaceship operator, showcasing its usage through clear examples and practical applications. Whether you’re a seasoned PHP developer or just starting out, understanding this operator can enhance your coding efficiency and make your comparisons more intuitive. Let’s explore how the spaceship operator works and how you can implement it in your PHP projects.
What is the Spaceship Operator?
The spaceship operator is a concise way to compare two values in PHP. It simplifies the traditional comparison methods, allowing developers to write cleaner and more readable code. The operator can be particularly useful in sorting functions and when working with complex data structures.
When using the spaceship operator, the outcome is straightforward:
- It returns -1 if the left operand is less than the right operand.
- It returns 0 if both operands are equal.
- It returns 1 if the left operand is greater than the right operand.
This functionality makes it a valuable addition to any PHP developer’s toolkit, especially when dealing with arrays or objects that require sorting or comparison.
Basic Usage of the Spaceship Operator
Let’s look at a simple example to illustrate how the spaceship operator works in PHP.
$a = 5;
$b = 10;
$result = $a <=> $b;
echo $result;
Output:
-1
In this example, we compare the values of $a
and $b
. Since 5 is less than 10, the spaceship operator returns -1, indicating that $a
is less than $b
. This is a straightforward demonstration, but the true power of the spaceship operator shines in more complex scenarios, such as sorting arrays.
Sorting Arrays with the Spaceship Operator
One of the most common applications of the spaceship operator is in sorting arrays. Let’s see how we can use it in a custom sorting function.
$array = [3, 1, 4, 1, 5, 9];
usort($array, function($a, $b) {
return $a <=> $b;
});
print_r($array);
Output:
Array
(
[0] => 1
[1] => 1
[2] => 3
[3] => 4
[4] => 5
[5] => 9
)
In this example, we define an array of integers and use the usort
function to sort it. The anonymous function utilizes the spaceship operator to compare each pair of elements. The result is a neatly sorted array. This approach is not only concise but also enhances readability, making it clear that we’re comparing values directly.
Comparing Strings with the Spaceship Operator
The spaceship operator can also be effectively used to compare strings. Here’s how it works with string values.
$str1 = "apple";
$str2 = "banana";
$result = $str1 <=> $str2;
echo $result;
Output:
-1
In this case, “apple” is lexicographically less than “banana”, so the spaceship operator returns -1. This functionality is particularly useful when you need to sort or compare string data, such as names or titles. It allows for a clear and efficient comparison without needing to write verbose conditional statements.
Handling Null Values with the Spaceship Operator
When dealing with null values, the spaceship operator can also come in handy. Here’s an example of how it behaves with null comparisons.
$value1 = null;
$value2 = 10;
$result = $value1 <=> $value2;
echo $result;
Output:
-1
In this scenario, null is considered less than any integer, so the operator returns -1. This behavior is crucial when you’re performing comparisons where null values may be present. It helps maintain consistency and clarity in your comparisons, ensuring that nulls are handled gracefully.
Conclusion
The PHP spaceship operator is a valuable tool for developers, offering a clean and efficient way to compare values. Whether you’re sorting arrays, comparing strings, or handling null values, the spaceship operator simplifies your code and enhances readability. By embracing this operator, you can write more intuitive and maintainable PHP code. As you continue to explore PHP’s features, integrating the spaceship operator into your coding practices will undoubtedly elevate your programming skills.
FAQ
-
What does the spaceship operator return when both values are equal?
It returns 0 when both values are equal. -
Can the spaceship operator be used with objects?
Yes, it can be used, but you need to ensure that the objects implement the appropriate comparison methods. -
Is the spaceship operator available in PHP versions prior to 7?
No, the spaceship operator was introduced in PHP 7. -
How does the spaceship operator handle string comparisons?
It compares strings lexicographically, returning -1, 0, or 1 based on their order. -
What are the advantages of using the spaceship operator?
It simplifies comparisons, enhances code readability, and reduces the need for verbose conditional statements.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook