PHP Spread Operator
- PHP Spread Operator
- Use the Spread Operator Multiple Times in PHP
- Use the Spread Operator With a Return Value of a Function in PHP
- Use the Spread Operator With a Traversable Object in PHP
- Use the Spread Operator With a Generator in PHP
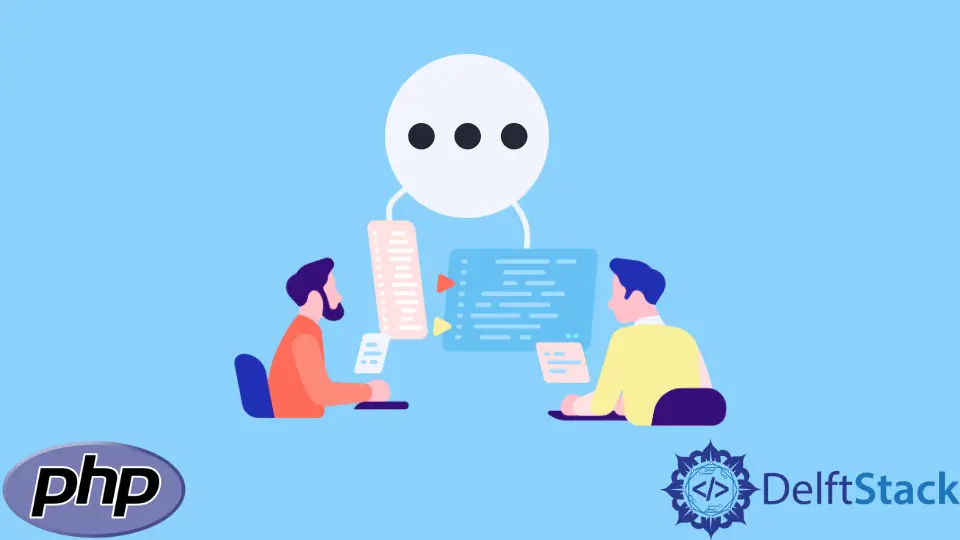
This tutorial demonstrates the spread operator in PHP.
PHP Spread Operator
The spread operator was introduced in PHP 7.4, which is used for the array expression. The spread operator is denoted by three dots ...
.
The spread operator spreads the members of an array, which means if we put these three dots into the prefix of an array, it will spread the values in the place.
For example:
<?php
$names = ["Jack", "John"];
$all_names = ["Mike", "Logan", "Shawn", ...$names]; // Use of the spread operator
print_r($all_names);
?>
The code above will use the spread operator to put the values of array $names
into the array $all_names
.
See output:
Array (
[0] => Mike
[1] => Logan
[2] => Shawn
[3] => Jack
[4] => John )
The array_merge()
method also performs the same operation, but the spread operator is better because it is a language construct that is always better than methods, and PHP will also optimize the performance for arrays at compile time.
We can use the spread operator anywhere in the array, for example, in the beginning, middle, or end.
Let’s see an example.
<?php
$names = ["Jack", "John"];
$all_names = ["Mike", ...$names, "Logan", "Shawn" ]; // Use of the spread operator in between
print_r($all_names);
echo "<br>";
$all_names1 = [...$names, "Mike", "Logan", "Shawn" ]; // Use of the spread operator at the begining
print_r($all_names1);
?>
The code will insert the $names
array to $all_names
arrays using the spread operator at different positions.
See output:
Array (
[0] => Mike
[1] => Jack
[2] => John
[3] => Logan
[4] => Shawn )
Array (
[0] => Jack
[1] => John
[2] => Mike
[3] => Logan
[4] => Shawn )
The spread operator can be used differently, according to position, times, functions or generators, etc. Let’s try examples for each usage of the spread operator of PHP.
Use the Spread Operator Multiple Times in PHP
The spread operator can also be used multiple times in an array. Let’s try an example.
<?php
$names1 = ["Jack", "John"];
$names2 = ["Mike", ...$names1, "Logan", "Shawn" ]; // Use of the spread operator
$all_names = [...$names1, ...$names2 ]; // Use of the multiple spread operator
print_r($all_names);
?>
The code above demonstrates the multiple uses of the spread operator. See output:
Array (
[0] => Jack
[1] => John
[2] => Mike
[3] => Jack
[4] => John
[5] => Logan
[6] => Shawn )
Use the Spread Operator With a Return Value of a Function in PHP
For example, if functions return an array, we can use the spread operator with that function call to put those values in the array. See the example.
<?php
function get_names()
{
$names = ["Jack", "John"];
return $names;
}
$names1 = ["Mike", "Logan", "Shawn" ];
$all_names = [...$names1, ...get_names() ]; // Use of the multiple spread operator with function call
print_r($all_names);
?>
The code above demonstrates the use of the PHP spread operator with the function return value.
See output:
Array (
[0] => Mike
[1] => Logan
[2] => Shawn
[3] => Jack
[4] => John )
Use the Spread Operator With a Traversable Object in PHP
The PHP spread operator can also be used with the objects, not only arrays. It can also be used with traversable objects, which means the object which implements the traversable interface.
Let’s try an example.
<?php
class Names implements IteratorAggregate
{
private $names = ["Mike", "Logan", "Shawn" ];
public function getIterator()
{
return new ArrayIterator($this->names);
}
}
$names = new Names();
$all_names = [...$names];
print_r($all_names);
?>
The code above demonstrates the use of the spread operator with the traversable object.
See output:
Array (
[0] => Mike
[1] => Logan
[2] => Shawn )
Use the Spread Operator With a Generator in PHP
The spread operator can also be used with generator functions.
For example:
<?php
function odd_numbers()
{
for($x =1; $x < 20; $x+=2){
yield $x;
}
}
$odd = [...odd_numbers()];
print_r($odd);
?>
The code above creates a generator function that generates the odd number between 1 and 20 and then uses the spread operator.
See output:
Array (
[0] => 1
[1] => 3
[2] => 5
[3] => 7
[4] => 9
[5] => 11
[6] => 13
[7] => 15
[8] => 17
[9] => 19 )
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook