Null Coalescing vs Elvis Operator in PHP
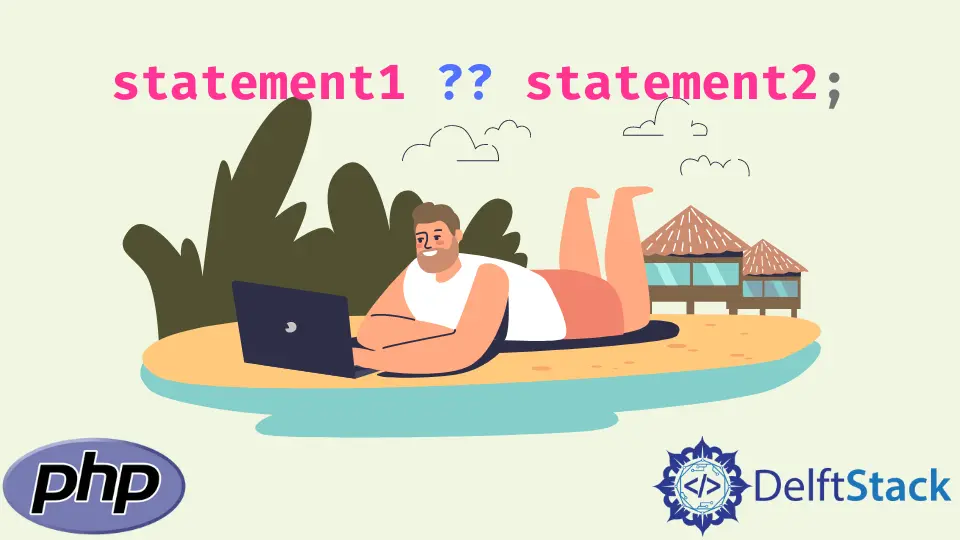
This article will differentiate the null coalescing operator and the Elvis operator in PHP with demonstrations.
Elvis Operator in PHP
First, let’s know about the Elvis operator. PHP Elvis operator is a shorthand operator for the ternary operator.
We can also say that it is a modified form of the ternary operator. To understand the PHP Elvis operator, we must know the ternary operator and how it works.
A ternary operator is a conditional operator used to perform a simple comparison or check on a condition having simple statements. It is a shorter version of the if-else
statement.
It decreases the length of code performing conditional operations. The order of the operation is from left to right.
Syntax:
condition? statement1 : statement2;
In the ternary operator, firstly, the condition
is evaluated. If the condition is true, statement1
is executed, and if the condition is false, statement2
is executed.
It is called a ternary operator as it takes three operands, i.e., a condition, a result statement for true, and a statement for false.
Example Code:
<?php
$marks = 80;
$result = $marks >= 40 ? 'Pass': 'Fail';
echo $result;
?>
The above example will display Pass
as the condition $marks >= 40
is true.
Output:
Pass
Now let’s have a look at the Elvis operator. It is slightly different from the ternary operator; it just leaves out the second operand (between condition
and statement2
) and makes it even shorter.
Syntax:
condition?:statement;
Here, if the condition
evaluates to true, it returns condition
. Else, it will return statement
.
The ternary representation of the above Elvis syntax is shown below.
conditon ? condition : statement;
Note: Values like 0
, false
, NULL
, ""
, []
, "0"
, etc., are considered to be false values in PHP.
Let’s see some examples of Elvis operators.
Example Code:
<?php
$name = "John Doe";
$yourName = $name ?: 'No name';
echo $yourName."<br>";
$marks = "";
$yourMarks = $marks ?: 'No marks';
echo $yourMarks."<br>";
$address = null;
$yourAddress = $address ?: 'No Address';
echo $yourAddress."<br>";
$age = false;
$yourAge = $age ?: 'No age';
echo $yourAge."<br>";
?>
Output:
John Doe
No marks
No Address
No age
Since the $name
variable returns true
, the variable’s value is evaluated. In the rest of the cases, the variables $marks
, $address
, and $age
return false
, so the operands on the right-hand side are evaluated.
Thus, we can conclude that the output of the Elvis operator depends on the true
or false
state of the condition.
Null Coalescing Operator in PHP
The null coalescing operator checks whether the given variable is null or not, and it returns the non-null value from the pair of the values. The output of the null coalescing operator depends on whether the variable is null.
Let’s see the syntax below to understand it.
statment1 ?? statement2;
Here, if statment1
evaluates to be null, statment2
will be returned. If statment1
is a non-null value, it will be returned.
In the null coalescing operator, it does not matter if the value of statement
is false
, 0
, or ''
. Even if statement
has falsy values, its value will be returned.
This is the main difference between the Elvis operator and the null coalescing operator. Some examples of the null coalescing operators are:
Example Code:
<?php
$name = "John Doe";
$yourName = $name ?? 'No name';
echo $yourName."<br>";
$marks = "";
$yourMarks = $marks ?? 'No marks';
echo $yourMarks."<br>";
$age = false;
$yourAge = $age ?? 'No age';
echo $yourAge."<br>";
$address = null;
$yourAddress = $address ?? 'No Address';
echo $yourAddress."<br>";
?>
Output:
John Doe
No Address
Here, the variable $name
is true, then it is evaluated and printed in the output section. Next, the variable $marks
is an empty string; an empty string is a falsy value.
The second operand is not evaluated since it is not a null value. There is a blank in the output section because the empty string is evaluated.
The case is the same for the $age
variable as it holds a falsy value but not a null value. Finally, the $address
variable has a null value; therefore, the second operand, No Address
, is evaluated.
From the above examples and explanation, we can conclude the significant difference between the Elvis operator and the null coalescing operator is that the Elvis operator checks whether the value is true. Still, the null coalescing operator checks if the value is not null.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn