How to Convert XML Into an Array in PHP
-
Use the
simplexml_load_string()
,json_encode()
, andjson_decode()
Functions to Convert XML Into an Array in PHP -
Use the
simplexml_load_file()
Function and Typecast It Into an Array to Convert XML Into Array in PHP
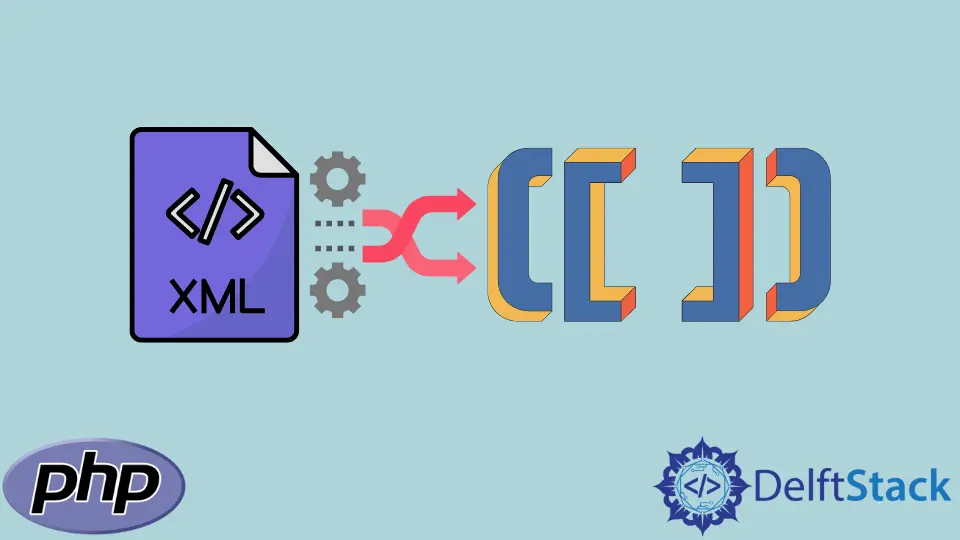
This tutorial will introduce how to convert XML into an array in PHP.
Use the simplexml_load_string()
, json_encode()
, and json_decode()
Functions to Convert XML Into an Array in PHP
We can represent XML data as a PHP array with a series of conversions.
We can use the simplexml_load_string()
function to interpret the XML string as an object. The function takes the XML string as its first parameter.
We can specify a class as the second option. The function will return the object of the specified class. The third parameter of the function is the LIBXML
constants.
Next, we should convert the object into JSON using the json_encode()
function. For the final part, we can use the json_decode()
function with the parameter TRUE
to convert the JSON into an array.
For example, consider the following XML data.
<students>
<bachelors>
<name>Jack</name>
<name>Hari</name>
</bachelors>
<masters>
<name>Sia</name>
<name>Paul</name>
</masters>
</students>
Create a variable $xmlstr
and store all the XML as a string in the variable. Next, create another variable $XML
and assign the simplexml_load_string()
function to it.
In the function, set the $xmlstr
as the first parameter and set SimpleXMLElement
as the class and LIBXML_NOCDATA
as the third parameter. Next, convert $XML
into JSON using the json_encode()
function.
Then, convert the JSON to array with the json_decode()
function. Do not forget to include TRUE
as the second parameter of the json_decode()
function. Finally, print the array.
Here, the LIBXML_NOCDATA
constant merges the character data (CDATA) as text nodes. Thus, we can convert XML into a PHP array using these various PHP functions.
$xmlstr ='
<students>
<bachelors>
<name>Jack</name>
<name>Hari</name>
</bachelors>
<masters>
<name>Sia</name>
<name>Paul</name>
</masters>
</students>';
$XML = simplexml_load_string($xmlstr, "SimpleXMLElement", LIBXML_NOCDATA);
$json = json_encode($XML);
$arr = json_decode($json,TRUE);
print_r($arr);
Output:
Array ( [bachelors] => Array ( [name] => Array ( [0] => Jack [1] => Hari ) ) [masters] => Array ( [name] => Array ( [0] => Sia [1] => Paul ) ) )
Use the simplexml_load_file()
Function and Typecast It Into an Array to Convert XML Into Array in PHP
We can use the simplexml_load_file()
function to interpret the XML into an object. Then, we can convert the object into an array by typecasting it.
The function takes the path of the XML file as the first parameter. The function is almost similar to the simplexml_load_string()
function. We can create a XML file and use its path in the simplexml_load_file()
function.
For example, we can use the same XML file in the first method, save the XML file as file.xml
, and create a variable $XML
in PHP. Assign the simplexml_load_file()
to the variable with file.xml
as the parameter.
Next, create a $arr
variable and typecast the $XML
variable into an array. Assign the typecasted array to the $arr
variable. Finally, print the $arr
variable using the print_r()
function.
In this way, we can use the simplexml_load_file()
function to load the XML file and convert it into an array.
$XML = simplexml_load_file('file.xml');
$arr = (array)$XML;
print_r($arr);
Output:
Array ( [bachelors] => SimpleXMLElement Object ( [name] => Array ( [0] => Jack [1] => Hari ) ) [masters] => SimpleXMLElement Object ( [name] => Array ( [0] => Sia [1] => Paul ) ) )
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn