How to Convert XML to JSON in PHP
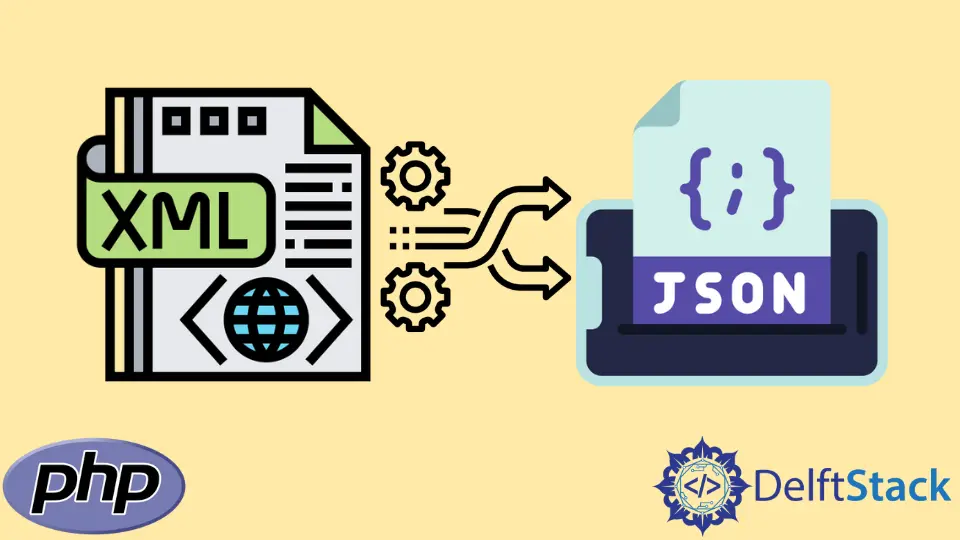
This article will introduce a method to convert an XML string to JSON in PHP.
Use the simplexml_load_string()
and json_encode()
Function to Convert an XML String to JSON in PHP
We will use two functions to convert an XML string to JSON in PHP because there is no specialized function for direct conversion. These two functions are simplexml_load_string()
and json_encode()
. The correct syntax to use these functions for the conversion of an XML string to JSON is as follows.
simplexml_load_string($data, $class_name, $options, $ns, $is_prefix);
The simplexml_load_string()
function accepts five parameters. The details of its parameters are as follows.
Variables | Description | |
---|---|---|
$data |
mandatory | A well-formed XML string. |
$class_name |
optional | We use this optional parameter so that simplexml_load_string() will return an object of the specified class. That class should extend the SimpleXMLElement class. |
options |
optional | We can also use the options parameter to specify additional Libxml parameters. |
ns |
optional | The namespace prefix or URI. |
$is_prefix |
optional | Set to true if $ns is a prefix, false if it is a URI. Its default value false . |
This function returns the object of class SimpleXMLElement
containing the data held within the XML string, or False
on failure.
json_encode($value, $flags, $depth);
The json_encode()
function has three parameters. The details of its parameters are as follows.
Variables | Description | |
---|---|---|
$value |
mandatory | The value being encoded. |
$flags |
optional | A bitmask consisting of JSON_FORCE_OBJECT , JSON_HEX_QUOT , JSON_HEX_TAG , JSON_HEX_AMP , JSON_HEX_APOS , JSON_INVALID_UTF8_IGNORE , JSON_INVALID_UTF8_SUBSTITUTE , JSON_NUMERIC_CHECK , JSON_PARTIAL_OUTPUT_ON_ERROR , JSON_PRESERVE_ZERO_FRACTION , JSON_PRETTY_PRINT , JSON_UNESCAPED_LINE_TERMINATORS , JSON_UNESCAPED_SLASHES , JSON_UNESCAPED_UNICODE , JSON_THROW_ON_ERROR . |
$depth |
optional | The maximum depth. It should be greater than zero. |
This function returns the JSON value. The program below shows the ways by which we can use the simplexml_load_string()
and json_encode()
function to convert an XML string to JSON in PHP.
<?php
$xml_string = <<<XML
<?xml version='1.0' standalone='yes'?>
<movies>
<movie>
<title>PHP: Behind the Parser</title>
<characters>
<character>
<name>Ms. Coder</name>
<actor>Onlivia Actora</actor>
</character>
<character>
<name>Mr. Coder</name>
<actor>El ActÓr</actor>
</character>
</characters>
<plot>
So, this language. It is like, a programming language. Or is it a
scripting language? All is revealed in this thrilling horror spoof
of a documentary.
</plot>
<great-lines>
<line>PHP solves all my web problems</line>
</great-lines>
<rating type="thumbs">7</rating>
<rating type="stars">5</rating>
</movie>
</movies>
XML;
$xml = simplexml_load_string($xml_string);
$json = json_encode($xml); // convert the XML string to JSON
var_dump($json);
?>
Output:
string(415) "{"movie":{"title":"PHP: Behind the Parser","characters":{"character":[{"name":"Ms. Coder","actor":"Onlivia Actora"},{"name":"Mr. Coder","actor":"El Act\u00d3r"}]},"plot":"\n So, this language. It is like, a programming language. Or is it a\n scripting language? All is revealed in this thrilling horror spoof\n of a documentary.\n ","great-lines":{"line":"PHP solves all my web problems"},"rating":["7","5"]}}"