How to Obtain and Read Data in PHP
-
GET
vs.POST
Methods in PHP -
Obtain and Read User Input With
file_get_content()
andphp://input
in PHP -
Obtain and Read Request With
file_get_content()
andjson_decode()
in PHP
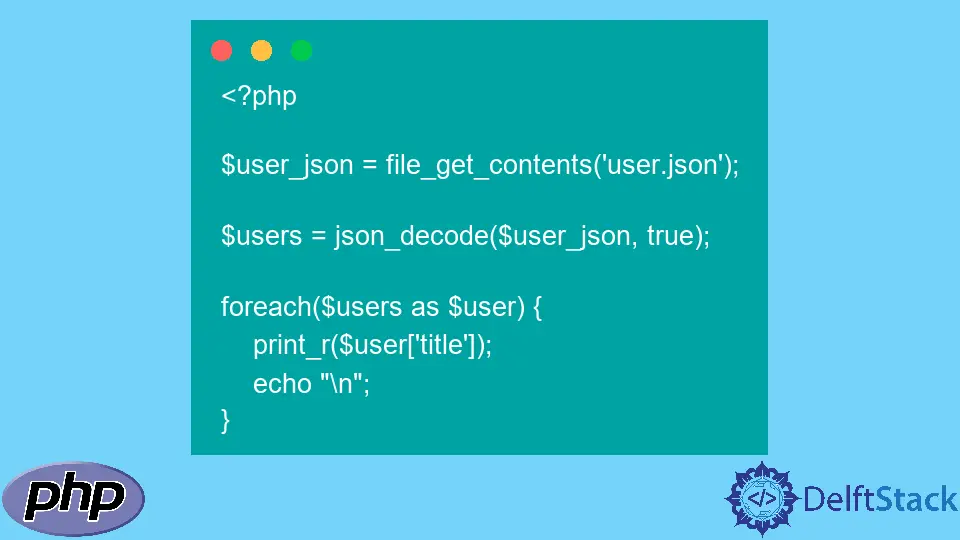
We will come across different content as we develop PHP applications. From time to time, we will need to read files and obtain content from users, other sources, and applications.
In PHP and most programming languages, there are different means and processes that we can use to obtain request and read content. Built-in functions, like file_get_content()
, json_decode()
allows us to get the content and decode JSON files, and wrappers like php://input
to read raw data from the request body.
This article will discuss functions we can use to obtain requests, user input, and read data in PHP.
GET
vs. POST
Methods in PHP
To obtain data, we have to transfer it from one source to another; a prevalent use case is from client to server or vice versa. To do such, we need two popular methods, GET
and POST
.
The GET
method requests data from a specified resource, and the POST
method sends data to a server for creating or updating a resource. However, only ASCII characters are allowed within the GET
method.
Stepping aside from the differences, we need to consider APIs (Application Programming Interfaces), which are the de-facto means of connecting with different applications. Most APIs provide their data in JSON format for us, the end-user, to read or use.
Obtain and Read User Input With file_get_content()
and php://input
in PHP
The wrapper php://input
allows us to read raw POST
data and provides a less memory-intensive alternative approach with no PHP configuration processes (special php.ini
directives). In addition, we can treat php://input
as a filename that we can use anywhere within our codebase within the context of the file_get_content()
process.
To put meaning to this explanation, let us make a simple login process that will use the file_get_content()
and the wrapper php://input
to read the users’ data from the form. The HTML form uses the POST
method to send the users’ data to the api.php
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div>
<form action="api.php" method="POST">
<form method="POST">
<label for="emai">Email address:</label><br>
<input type="text" id="email" name="email"><br>
<label for="password">Password:</label><br>
<input type="text" id="password" name="password">
<br><br>
<button type="submit" name="btn_login" value="btn_login"> Login </button>
</form>
</div>
</body>
</html>
The api.php
uses the function and wrapper and displays the information using the var_dump()
function.
<?php
$data2 = file_get_contents('php://input');
var_dump($data2);
?>
The HTML form with the user input is shown below:
The api.php
processing of the user input is shown below:
Obtain and Read Request With file_get_content()
and json_decode()
in PHP
To read a JSON file, we can use the built-in function file_get_content()
to read the JSON file, and then decode the string we obtain with the function json_decode()
.
JSON (JavaScript Object Notation) is an open standard file format that stores data in key-pair format as a string. With the json_decode()
function, we can process the key-pair and create an associative array or objects that PHP understands.
Aside from the string in the file_get_content()
function, there is another important argument, the associative
parameter. It can be set to two values: true or false; if true, the string will be stored in an associative array, and if false, it will be stored as an object.
Let’s read this JSON file:
[
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
},
{
"userId": 1,
"id": 2,
"title": "qui est esse",
"body": "est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla"
},
{
"userId": 1,
"id": 3,
"title": "ea molestias quasi exercitationem repellat qui ipsa sit aut",
"body": "et iusto sed quo iure\nvoluptatem occaecati omnis eligendi aut ad\nvoluptatem doloribus vel accusantium quis pariatur\nmolestiae porro eius odio et labore et velit aut"
},
{
"userId": 1,
"id": 4,
"title": "eum et est occaecati",
"body": "ullam et saepe reiciendis voluptatem adipisci\nsit amet autem assumenda provident rerum culpa\nquis hic commodi nesciunt rem tenetur doloremque ipsam iure\nquis sunt voluptatem rerum illo velit"
},
{
"userId": 1,
"id": 5,
"title": "nesciunt quas odio",
"body": "repudiandae veniam quaerat sunt sed\nalias aut fugiat sit autem sed est\nvoluptatem omnis possimus esse voluptatibus quis\nest aut tenetur dolor neque"
}
]
Using this PHP code, we can store the JSON file as an associative array and read the first array element in the array.
<?php
$user_json = file_get_contents('user.json');
$users = json_decode($user_json, true);
print_r($users[0]);
We can also use the foreach
loop to read all the titles in the JSON file.
<?php
$user_json = file_get_contents('user.json');
$users = json_decode($user_json, true);
foreach($users as $user) {
print_r($user['title']);
echo "\n";
}
The output of the code snippet is:
sunt aut facere repellat provident occaecati excepturi optio reprehenderit
qui est esse
ea molestias quasi exercitationem repellat qui ipsa sit aut
eum et est occaecati
nesciunt quas odio
However, if we want to read JSON data directly from the API, we can use the cURL library and the json_encode
function. You can check out this example code on GitHub.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn