How to Use cURL to Get JSON Data and Decode JSON Data in PHP
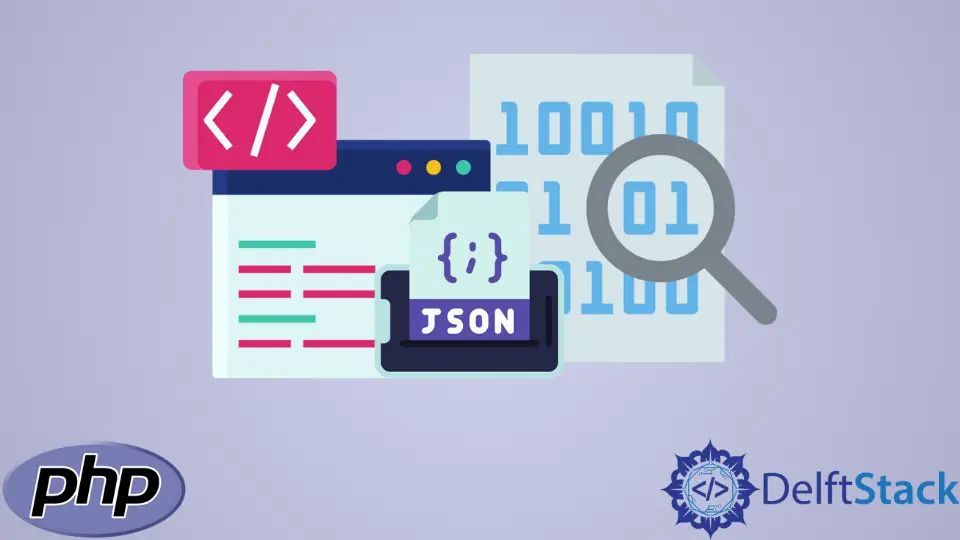
In this article, we will introduce methods to use cURL
to get JSON
data and decode JSON
data in PHP.
- Using
cURL
functions
Use cURL
Functions to Use cURL
to Get JSON Data and Decode JSON Data in PHP
There are different functions of cURL
that are used collectively to get JSON
data and decode JSON data. These are curl_init()
, curl_setopt()
, curl_exec()
and curl_close()
. The detail of these functions is as follows
The function curl_init()
is used to initialize a new session for using cURL
functions. The correct syntax to use this function is as follows
curl_init($url);
The parameters $url
is an optional parameter. If it is provided, its value is set to CURLOPT_URL
. If not, then we can set it later. On success, this function returns a cURL
handle.
The function curl_setopt()
is used to set an option for cURL
process. The correct syntax to use this function is as follows
curl_setopt($handle, $option, $value);
The first parameter is the handle returned by the curl_init()
function. The second parameter is the option for the cURL
process. The third parameter is the value of the option selected. You can check options here.
The function curl_exec()
executes the cURL
session. It returns true on success and false on failure. The correct syntax to use this function is as follows.
curl_exec($handle);
It has only one parameter $handle
that is the handle returned by the curl_init()
function.
The function curl_close()
closes the session initialized by curl_init()
function. The correct syntax to use this function is as follows
curl_close($handle);
It only accepts one parameter $handle
that is the handle returned by the curl_init()
function.
Now we will use these functions to get JSON
data and decode JSON
data.
// Initiate curl session
$handle = curl_init();
// Will return the response, if false it prints the response
curl_setopt($handle, CURLOPT_RETURNTRANSFER, true);
// Set the url
curl_setopt($handle, CURLOPT_URL,$YourUrl);
// Execute the session and store the contents in $result
$result=curl_exec($handle);
// Closing the session
curl_close($handle);
Now we will use file_get_contents()
function to get the JSON
data from the URL and json_decode()
function to convert JSON
string to an array.
$result = file_get_contents($url);
$array = json_decode($result, true);
var_dump($array);
The function var_dump()
will display the JSON
data in the form of an array.