How to Pretty Print the JSON in PHP
-
Use the HTML
<pre>
Tag and theJSON_PRETTY_PRINT
Option to Prettify the JSON String in PHP -
Use the
application/json
andJSON_PRETTY_PRINT
Options to Prettify the JSON String in PHP -
Use the
json_encode()
andjson_decode()
Functions to Prettify the JSON String in PHP
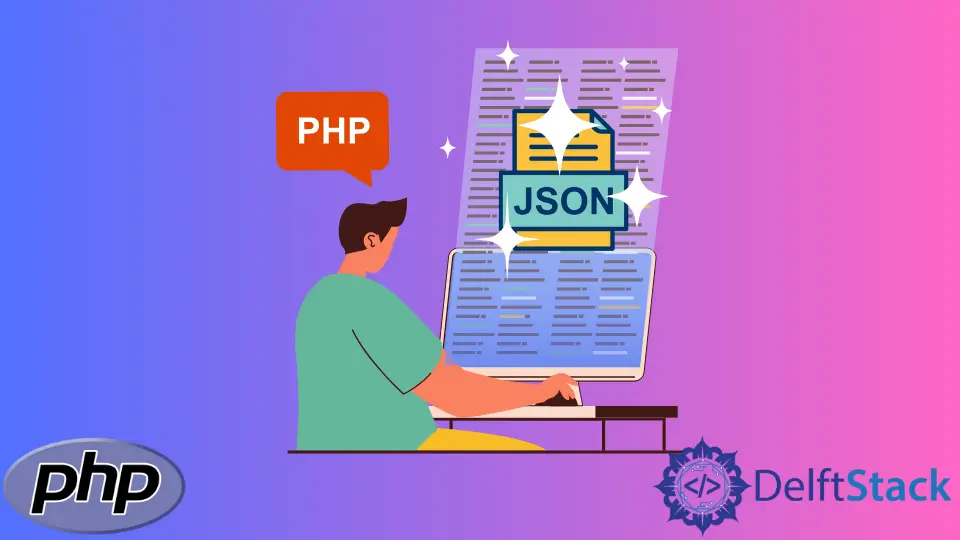
This article will introduce different methods to prettify the raw JSON string in PHP.
Use the HTML <pre>
Tag and the JSON_PRETTY_PRINT
Option to Prettify the JSON String in PHP
We can use the json_encode()
function to convert a value to a JSON format. We can encode indexed array, associative array, and objects to the JSON format. The json_encode()
function has a option JSON_PRETTY_PRINT
which prettifies the JSON string. We can specify the string to be prettified and then the option in the json_encode()
function. It will add some spaces between the characters and makes the string looks better. However, we can use the <pre>
HTML tags to indent the strings to the new line. We will prettify an associative array in the example below. The tag preserves the line break after each key-value pair in the string.
For example, create an associative array in the variable $age
. Write the keys Marcus
, Mason
, and Jadon
and the values 23
, 19
, and 20
. Next, use the json_encode()
function on the $age
variable and write the option JSON_PRETTY_PRINT
as the second parameter and store the expression in $json_pretty
variable. Then, echo the variable enclosing it with the HTML <pre>
tag.
Example Code:
$age = array("Marcus"=>23, "Mason"=>19, "Jadon"=>20)
$json_pretty = json_encode($age, JSON_PRETTY_PRINT);
echo "<pre>".$json_pretty."<pre/>";
Output:
{
"Marcus": 23,
"Mason": 19,
"Jadon": 20
}
Use the application/json
and JSON_PRETTY_PRINT
Options to Prettify the JSON String in PHP
We can use the header()
function to set the Content-Type
to application/json
to notify the browser type. It will display the data in JSON format. We can use the JSON_PRETTY_PRINT
option as in the first method to prettify the string. We will use the same associative array for the demonstration. We can use the json_encode()
function as in the first method.
For example, write the header()
function and set the Content-Type
to application/json
. In the next line, use the json_encode()
function with the JSON_PRETTY_PRINT
option on the array as we did in the first method. As a result, we will get a prettified version of JSON data in each new line.
Example Code:
$age = array("Marcus"=>23, "Mason"=>19, "Jadon"=>20);
header('Content-Type: application/json');
echo json_encode($age, JSON_PRETTY_PRINT);
?>
Output:
{
"Marcus": 23,
"Mason": 19,
"Jadon": 20
}
Use the json_encode()
and json_decode()
Functions to Prettify the JSON String in PHP
We can use the json_encode()
function with the json_decode()
function and the JSON_PRETTY_PRINT
as the parameters to prettify the JSON string in PHP. We also use the header()
function like in the second method to notify the browser about the JSON format. We will prettify a JSON object in the following example. We will take the JSON object and decode it using the json_decode()
function and then will encode it with the json_encode()
function along with the JSON_PRETTY_PRINT
option.
For example, set the Content-Type
to application/json
as we did in the method above. Create a variable $json1
and store a raw JSON object in it. Then, use the json_decode()
function on the variable $json1
. Use the decoded JSON object as the first parameter to the json_encode()
function and the JSON_PRETTY_PRINT
option as the second parameter. Store the expression in a $json2
variable and echo it.
Example Code:
header('Content-Type: application/json');
$json1 = '{"a":10,"b":20,"c":30,"d":40,"e":50}';
$json2 = json_encode(json_decode($json1), JSON_PRETTY_PRINT);
echo $json2;
Output:
{
"a": 10,
"b": 20,
"c": 30,
"d": 40,
"e": 50
}
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn