How to Convert CSV File to Array in PHP
-
Use the
fopen()
andfgetcsv()
to Convert CSV to Array in PHP -
Use the
str_getcsv()
to Convert CSV to Array in PHP -
Use the
array_map()
to Convert CSV to Array in PHP
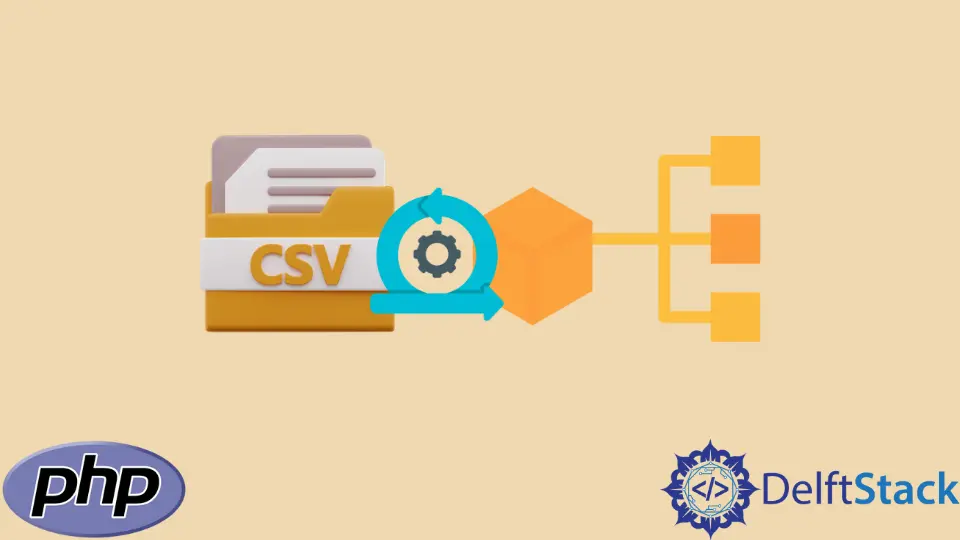
Comma Separated Values (CSV) files are a big part of the data pipeline. Regardless of the programming language, your application has a place for CSV.
CSV files are popular for storing data in row and column format. Therefore, arrays are the perfect data structure to hold their values when we process CSV files within our PHP application.
In this article, we will detail and show you the built-in PHP functions that can allow us to read and convert CSV to an array in PHP.
Use the fopen()
and fgetcsv()
to Convert CSV to Array in PHP
For this section and the other two sections, we will use a CSV file holding state housing values (state_housing_values.csv
) with 51 rows and 4 columns to show how these functions work.
First, we need the fopen()
function to open the CSV file and point it to the fgetcsv()
function as the first argument, with the rest of the arguments optional. The other parameters include length
, separator
, enclosure
, and escape
.
Now, let’s convert the CSV file to an array.
$file = fopen('state_housing_values.csv', 'r');
while (($line = fgetcsv($file)) !== FALSE) {
var_dump($line);
}
fclose($file);
Output:
array(4) {
[0]=>
string(5) "State"
[1]=>
string(5) "Price"
[2]=>
string(13) "1-year change"
[3]=>
string(15) "1-year forecast"
}
array(4) {
[0]=>
string(7) "Alabama"
[1]=>
string(7) "143,072"
[2]=>
string(3) "5.5"
[3]=>
string(4) "-1.1"
}
array(4) {
[0]=>
string(6) "Alaska"
[1]=>
string(7) "300,073"
[2]=>
string(4) "-2.3"
[3]=>
string(4) "-4.8"
}
array(4) {
[0]=>
string(7) "Arizona"
[1]=>
string(7) "277,574"
[2]=>
string(3) "7.5"
[3]=>
string(4) "-0.5"
}
array(4) {
[0]=>
string(8) "Arkansas"
[1]=>
string(7) "130,907"
[2]=>
string(3) "3.8"
[3]=>
string(4) "-1.7"
}
array(4) {
[0]=>
string(10) "California"
[1]=>
string(7) "578,267"
[2]=>
string(3) "4.4"
[3]=>
string(4) "-1.1"
}
...
Before we move on, we can remove the header, which might not be needed in our PHP codebase. To do so, we can just add $line = fgetcsv($file)
before the while
loop.
$file = fopen('state_housing_values.csv', 'r');
$line = fgetcsv($file);
while (($line = fgetcsv($file)) !== FALSE) {
var_dump($line);
}
fclose($file);
Output:
array(4) {
[0]=>
string(7) "Alabama"
[1]=>
string(7) "143,072"
[2]=>
string(3) "5.5"
[3]=>
string(4) "-1.1"
}
array(4) {
[0]=>
string(6) "Alaska"
[1]=>
string(7) "300,073"
[2]=>
string(4) "-2.3"
[3]=>
string(4) "-4.8"
}
...
With this, the header is no longer in the array,
The above array format is very hard to work with. To make the array more workable, we need to refactor the code and use array_combine()
.
<?php
$filename = 'state_housing_values.csv';
$delimiter = ',';
if (file_exists($filename) || is_readable($filename)) {
$header = NULL;
$data = array();
if (($handle = fopen($filename, 'r')) !== FALSE) {
while (($row = fgetcsv($handle, 1000, $delimiter)) !== FALSE) {
if (!$header)
$header = $row;
else
$data[] = array_combine($header, $row);
}
fclose($handle);
}
var_dump($data);
} else {
error_log("Error reading file.");
}
?>
Output:
array(50) {
[0]=>
array(4) {
["State"]=>
string(7) "Alabama"
["Price"]=>
string(7) "143,072"
["1-year change"]=>
string(3) "5.5"
["1-year forecast"]=>
string(4) "-1.1"
}
[1]=>
array(4) {
["State"]=>
string(6) "Alaska"
["Price"]=>
string(7) "300,073"
["1-year change"]=>
string(4) "-2.3"
["1-year forecast"]=>
string(4) "-4.8"
}
...
There are cases where the delimiter is not a comma ,
. Therefore, we will need to specify the delimiter.
Other delimiters could be a semi-colon ;
, space " "
, or a tab \t
. To specify the delimiter within the function, you can follow the code below.
$delimiter = ';'; // semi-colon
fgetcsv($handle, 1000, $delimiter)
Use the str_getcsv()
to Convert CSV to Array in PHP
Here, we will make use of the file()
function to process the CSV, loop through the file pointer using the foreach
loop and place each value within the array $data
via the str_getcsv()
function.
$data = array();
$lines = file('state_housing_values.csv', FILE_IGNORE_NEW_LINES);
foreach ($lines as $key => $value) {
$data[$key] = str_getcsv($value);
}
print_r($data);
Output:
Array
(
[0] => Array
(
[0] => State
[1] => Price
[2] => 1-year change
[3] => 1-year forecast
)
[1] => Array
(
[0] => Alabama
[1] => 143,072
[2] => 5.5
[3] => -1.1
)
[2] => Array
(
[0] => Alaska
[1] => 300,073
[2] => -2.3
[3] => -4.8
)
[3] => Array
(
[0] => Arizona
[1] => 277,574
[2] => 7.5
[3] => -0.5
)
[4] => Array
(
[0] => Arkansas
[1] => 130,907
[2] => 3.8
[3] => -1.7
)
[5] => Array
(
[0] => California
[1] => 578,267
[2] => 4.4
[3] => -1.1
)
[6] => Array
(
[0] => Colorado
[1] => 408,794
[2] => 3.1
[3] => -1.7
)
[7] => Array
(
[0] => Connecticut
[1] => 259,855
[2] => 1.4
[3] => -2.3
)
[8] => Array
(
[0] => Delaware
[1] => 257,521
[2] => 0
[3] => -2.9
)
[9] => Array
(
[0] => Florida
[1] => 252,309
[2] => 3.6
[3] => -1.5
)
[10] => Array
(
[0] => Georgia
[1] => 206,804
[2] => 4.9
[3] => -1.5
)
...
Add some changes to the above code to remove the header. We will use the if
conditional statement to remove the first array that holds the header.
foreach ($lines as $key => $value) {
if (!$key == 0) {
$data[$key] = str_getcsv($value);
}
}
print_r($data);
Output:
Array
(
[1] => Array
(
[0] => Alabama
[1] => 143,072
[2] => 5.5
[3] => -1.1
)
...
Use the array_map()
to Convert CSV to Array in PHP
The above code snippets used loops or pre-processing to convert CSV to an array. However, there is a better, faster, and easy-to-read method to convert CSV files to an array within PHP using the array_map()
and file()
functions.
$csv = array_map('str_getcsv', file('state_housing_values.csv'));
print_r($csv);
Output:
Array
(
[0] => Array
(
[0] => State
[1] => Price
[2] => 1-year change
[3] => 1-year forecast
)
...
[50] => Array
(
[0] => Wyoming
[1] => 256,089
[2] => 5.7
[3] => -1.6
)
)
These functions can handle CSV files with lots of values, and to showcase that, we will make use of the array_map()
function on a CSV file holding leverage data across 1857 rows and 17 columns, and also, remove the header of the array.
$csv = array_map('str_getcsv', file('leverage.csv'));
unset($csv[0]);
print_r($csv);
The output of the code (which exited at 0.257 seconds) is below.
Array
(
[1] => Array
(
[0] => 001300
[1] => 20150331
[2] => 2015
[3] => 1
[4] => INDL
[5] => C
[6] => D
[7] => STD
[8] => USD
[9] => 2015Q1
[10] => 2015Q1
[11] => 45357.0000
[12] => 781.7070
[13] => 5661.0000
[14] => 15432.0000
[15] => A
[16] => 104.3100
)
...
[1856] => Array
(
[0] => 252940
[1] => 20171231
[2] => 2017
[3] => 3
[4] => INDL
[5] => C
[6] => D
[7] => STD
[8] => USD
[9] => 2017Q4
[10] => 2017Q3
[11] =>
[12] =>
[13] =>
[14] =>
[15] => A
[16] => 7.2700
)
)
The CSV files are available below to play with the code.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn