How to Parse a CSV File in PHP
- Understanding CSV Files
- Method 1: Using fopen and fgetcsv
- Method 2: Using SplFileObject
- Method 3: Parsing CSV with PHP’s built-in functions
- Conclusion
- FAQ
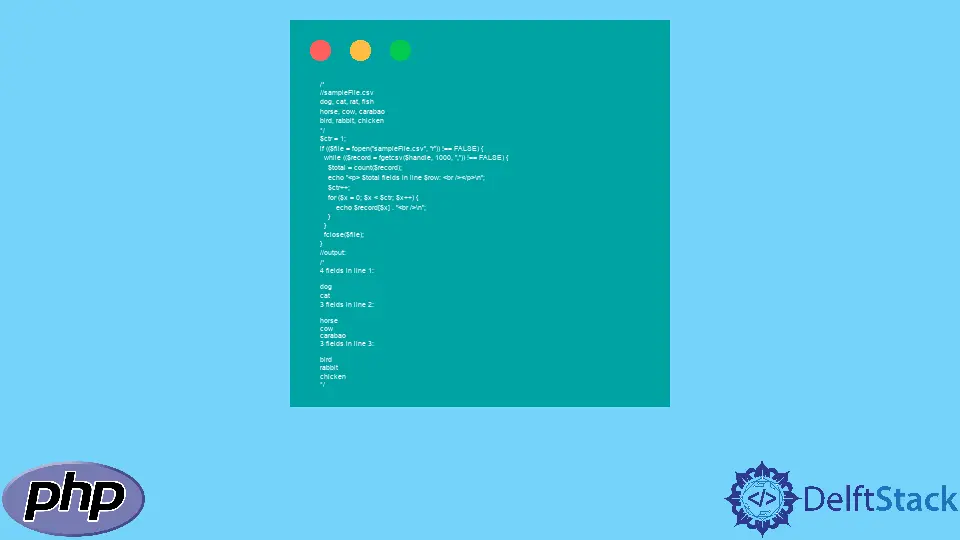
When working with data, CSV files are a common format for storing and sharing information. Whether you’re importing data from a spreadsheet or exporting it for use in another application, knowing how to parse a CSV file in PHP is essential. PHP offers several built-in functions that make this task straightforward and efficient.
In this article, we will explore different methods to parse CSV files using PHP functions, focusing on the combination of fopen
, fgetcsv
, and fclose
. By the end of this guide, you’ll be equipped with the knowledge to handle CSV files effortlessly in your PHP projects.
Understanding CSV Files
CSV, or Comma-Separated Values, is a simple text format used to store tabular data. Each line in a CSV file corresponds to a row in a table, with individual values separated by commas. This format is widely supported across various platforms, making it a popular choice for data interchange. When parsing CSV files in PHP, you can easily convert this data into arrays or objects for further processing. Now, let’s dive into the methods you can use to parse CSV files in PHP.
Method 1: Using fopen and fgetcsv
The most common way to parse a CSV file in PHP is by using the fopen
function to open the file and fgetcsv
to read its contents line by line. This method is efficient and allows for handling large files without consuming too much memory.
Here’s a simple example to illustrate this approach:
<?php
$file = fopen("data.csv", "r");
$data = [];
while (($row = fgetcsv($file)) !== FALSE) {
$data[] = $row;
}
fclose($file);
print_r($data);
?>
In this code, we first open the CSV file using fopen
, specifying the mode as “r” for reading. We initialize an empty array, $data
, to store the parsed rows. The fgetcsv
function is called in a while
loop, which continues until there are no more rows to read. Each row is appended to the $data
array. Finally, we close the file with fclose
and print the contents of the array.
Output:
Array
(
[0] => Array
(
[0] => Name
[1] => Age
[2] => City
)
[1] => Array
(
[0] => John
[1] => 30
[2] => New York
)
[2] => Array
(
[0] => Jane
[1] => 25
[2] => Los Angeles
)
)
This method is straightforward and effective for reading CSV files. The fgetcsv
function automatically handles the parsing of each line into an array, making it easy to work with the data afterward. Additionally, this approach can be adapted to handle different delimiters or enclosures by passing optional parameters to fgetcsv
.
Method 2: Using SplFileObject
Another powerful method to parse CSV files in PHP is by utilizing the SplFileObject
class. This object-oriented approach provides a more flexible interface for file handling and is particularly useful when dealing with larger datasets.
Here’s how you can use SplFileObject
to parse a CSV file:
<?php
$file = new SplFileObject("data.csv");
$file->setFlags(SplFileObject::READ_CSV);
$data = [];
foreach ($file as $row) {
$data[] = $row;
}
print_r($data);
?>
In this example, we create a new SplFileObject
instance for our CSV file. By setting the READ_CSV
flag, we instruct PHP to automatically parse each line as a CSV. We then iterate over the file using a foreach loop, appending each row to the $data
array. Finally, we print the parsed data.
Output:
Array
(
[0] => Array
(
[0] => Name
[1] => Age
[2] => City
)
[1] => Array
(
[0] => John
[1] => 30
[2] => New York
)
[2] => Array
(
[0] => Jane
[1] => 25
[2] => Los Angeles
)
)
Using SplFileObject
is advantageous for its simplicity and built-in functionality. It automatically handles the CSV parsing, which reduces the amount of code you need to write. This method is especially useful for larger files, as it allows you to read data in a more memory-efficient manner.
Method 3: Parsing CSV with PHP’s built-in functions
PHP also provides a variety of built-in functions for handling CSV files, such as fgetcsv
, fputcsv
, and more. You can leverage these functions for both reading and writing CSV data. Here, we will focus on reading CSV files.
<?php
$file = fopen("data.csv", "r");
$data = [];
while (($row = fgetcsv($file, 1000, ",")) !== FALSE) {
$data[] = $row;
}
fclose($file);
print_r($data);
?>
This example is similar to the first method but includes an additional parameter in the fgetcsv
function. The second parameter specifies the maximum line length, while the third parameter defines the delimiter (in this case, a comma). This allows for greater control over how the CSV file is parsed.
Output:
Array
(
[0] => Array
(
[0] => Name
[1] => Age
[2] => City
)
[1] => Array
(
[0] => John
[1] => 30
[2] => New York
)
[2] => Array
(
[0] => Jane
[1] => 25
[2] => Los Angeles
)
)
This method is particularly useful when you need to customize how the CSV data is read. By adjusting the parameters of fgetcsv
, you can tailor the parsing process to suit your specific needs, such as handling different delimiters or line lengths.
Conclusion
Parsing CSV files in PHP is a straightforward process thanks to the various built-in functions available. Whether you choose to use fopen
and fgetcsv
, SplFileObject
, or other built-in functions, each method offers unique advantages. By mastering these techniques, you can efficiently handle CSV data in your PHP applications. As you work with CSV files, remember to consider the size of your data and choose the method that best suits your needs. Happy coding!
FAQ
-
What is a CSV file?
A CSV file is a text file that uses commas to separate values, making it a common format for storing tabular data. -
How do I read a CSV file in PHP?
You can read a CSV file in PHP using functions likefopen
,fgetcsv
, or by using theSplFileObject
class. -
Can I parse CSV files with different delimiters?
Yes, you can specify different delimiters when using thefgetcsv
function by passing the desired delimiter as an argument. -
What is the advantage of using
SplFileObject
?
SplFileObject
provides a more flexible and memory-efficient way to handle large CSV files compared to traditional file handling methods. -
How can I write data to a CSV file in PHP?
You can write data to a CSV file using thefputcsv
function, which takes an array and writes it as a CSV line.