How to Add Element to an Associative Array in PHP
- Add Elements to the End of an Associative Array in PHP
-
Use the
array_merge()
Function to Add Elements at the Beginning of an Associative Array in PHP -
Use the
AddBetween
Function to Add an Element in Between Associative Array in PHP
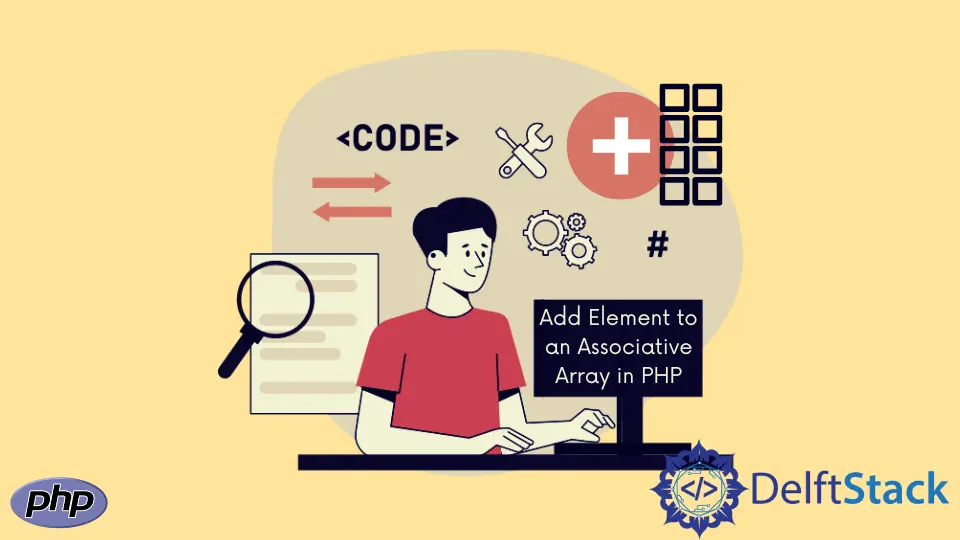
PHP has different ways to add items to an associative array.
If we want to add items to the start of the array, we can use built-in functions like array_merge()
.
We need to do it dynamically to add elements before a particular key of an associative array.
Add Elements to the End of an Associative Array in PHP
We can add elements to the end of an associative array by adding keys with values or by concatenating a new key-value to the array.
<?php
//First Method
$demo_array = array('Jack' => '10');
$demo_array['Michelle'] = '11'; // adding elements by pushing method
$demo_array['Shawn'] = '12';
echo "By Simple Method: <br>";
print_r($demo_array);
echo "<br>";
echo "Replacing the value: <br>";
$demo_array['Jack'] = '13'; // replaces the value at Jack
print_r($demo_array);
echo "<br>";
//Second method
//$demo_array += [$key => $value];
$demo_array += ['John' => '14'];
echo "By Concating Method: <br>";
print_r($demo_array);
?>
The code above tries to add elements to the end of an array by two methods.
Output:
By Simple Method:
Array ( [Jack] => 10 [Michelle] => 11 [Shawn] => 12 )
Replacing the value:
Array ( [Jack] => 13 [Michelle] => 11 [Shawn] => 12 )
By Concating Method:
Array ( [Jack] => 13 [Michelle] => 11 [Shawn] => 12 [John] => 14 )
Use the array_merge()
Function to Add Elements at the Beginning of an Associative Array in PHP
To add elements at the beginning of an associative, we can use the array union of the array_merge()
function.
<?php
$demo_array = array('Senior Developer' => 'Jack', 'Junior Developer' => 'Michelle', 'Intern' => 'John');
echo "The original array : ";
print_r($demo_array);
$temp_array = array('Project Manager' => 'Shawn'); //As per hierarchy Project manager should be at number 1.
// Using array union
$union_array = $temp_array + $demo_array;
echo "<br> The new associative array using union : ";
print_r($union_array);
// Using array_merge() function
$merge_array = array_merge($temp_array, $demo_array);
echo "<br> The new associative array using Array_merge : ";
print_r($merge_array);
?>
The code above uses the union_array
method and array_merge()
to add elements at the beginning of an array.
Output:
The original array : Array ( [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
The new associative array using union : Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
The new associative array using Array_merge : Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
Use the AddBetween
Function to Add an Element in Between Associative Array in PHP
PHP has no built-in functionality to add elements between the given array. But we can create a function that adds an element before the given key.
<?php
function AddBetween( $original_array, $before_key, $new_key, $new_value ) {
$added_array = array();
$added_key = false;
foreach( $original_array as $key => $value ) {
if( !$added_key && $key === $before_key ) {
$added_array[ $new_key ] = $new_value;
$added_key = true;
}
$added_array[ $key ] = $value;
}
return $added_array;
}
$demo_array = array('Project Manager' => 'Shawn', 'Senior Developer' => 'Jack', 'Intern' => 'John');
echo "The Original Array is: <br>";
print_r($demo_array);
echo "<br>";
//Add 'Junior Developer' => 'Michelle' before intern as per hierarchy.
$added_array = AddBetween( $demo_array, 'Intern', 'Junior Developer', 'Michelle' );
echo "The Array With Added Element is: <br>";
print_r($added_array);
?>
The function AddBetween
tries to add an element before a given key.
Output:
The Original Array is:
Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Intern] => John )
The Array With Added Element is:
Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
The Junior Developer
was added before the intern
as per the hierarchy.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook