在 PHP 中将元素添加到关联数组
Sheeraz Gul
2023年1月30日
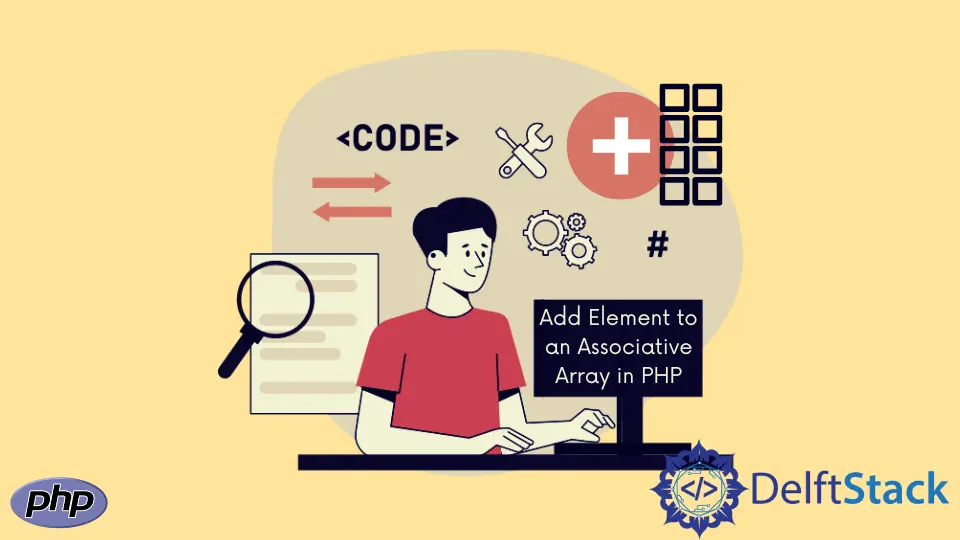
PHP 有不同的方法将项目添加到关联数组中。
如果我们想将项目添加到数组的开头,我们可以使用内置函数,如 array_merge()
。
我们需要动态地在关联数组的特定键之前添加元素。
在 PHP 中将元素添加到关联数组的末尾
我们可以通过添加键和值或将新的键值连接到数组来将元素添加到关联数组的末尾。
<?php
//First Method
$demo_array = array('Jack' => '10');
$demo_array['Michelle'] = '11'; // adding elements by pushing method
$demo_array['Shawn'] = '12';
echo "By Simple Method: <br>";
print_r($demo_array);
echo "<br>";
echo "Replacing the value: <br>";
$demo_array['Jack'] = '13'; // replaces the value at Jack
print_r($demo_array);
echo "<br>";
//Second method
//$demo_array += [$key => $value];
$demo_array += ['John' => '14'];
echo "By Concating Method: <br>";
print_r($demo_array);
?>
上面的代码尝试通过两种方法将元素添加到数组的末尾。
输出:
By Simple Method:
Array ( [Jack] => 10 [Michelle] => 11 [Shawn] => 12 )
Replacing the value:
Array ( [Jack] => 13 [Michelle] => 11 [Shawn] => 12 )
By Concating Method:
Array ( [Jack] => 13 [Michelle] => 11 [Shawn] => 12 [John] => 14 )
在 PHP 中使用 array_merge()
函数在关联数组的开头添加元素
要在关联的开头添加元素,我们可以使用 array_merge()
函数的数组联合。
<?php
$demo_array = array('Senior Developer' => 'Jack', 'Junior Developer' => 'Michelle', 'Intern' => 'John');
echo "The original array : ";
print_r($demo_array);
$temp_array = array('Project Manager' => 'Shawn'); //As per hierarchy Project manager should be at number 1.
// Using array union
$union_array = $temp_array + $demo_array;
echo "<br> The new associative array using union : ";
print_r($union_array);
// Using array_merge() function
$merge_array = array_merge($temp_array, $demo_array);
echo "<br> The new associative array using Array_merge : ";
print_r($merge_array);
?>
上面的代码使用 union_array
方法和 array_merge()
在数组的开头添加元素。
输出:
The original array : Array ( [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
The new associative array using union : Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
The new associative array using Array_merge : Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
在 PHP 中使用 AddBetween
函数的关联数组之间添加元素
PHP 没有在给定数组之间添加元素的内置函数。但是我们可以创建一个在给定键之前添加元素的函数。
<?php
function AddBetween( $original_array, $before_key, $new_key, $new_value ) {
$added_array = array();
$added_key = false;
foreach( $original_array as $key => $value ) {
if( !$added_key && $key === $before_key ) {
$added_array[ $new_key ] = $new_value;
$added_key = true;
}
$added_array[ $key ] = $value;
}
return $added_array;
}
$demo_array = array('Project Manager' => 'Shawn', 'Senior Developer' => 'Jack', 'Intern' => 'John');
echo "The Original Array is: <br>";
print_r($demo_array);
echo "<br>";
//Add 'Junior Developer' => 'Michelle' before intern as per hierarchy.
$added_array = AddBetween( $demo_array, 'Intern', 'Junior Developer', 'Michelle' );
echo "The Array With Added Element is: <br>";
print_r($added_array);
?>
AddBetween
函数尝试在给定键之前添加一个元素。
输出:
The Original Array is:
Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Intern] => John )
The Array With Added Element is:
Array ( [Project Manager] => Shawn [Senior Developer] => Jack [Junior Developer] => Michelle [Intern] => John )
根据层次结构,在 intern
之前添加了 Junior Developer
。
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook