How to Set X Axis Values in Matplotlib
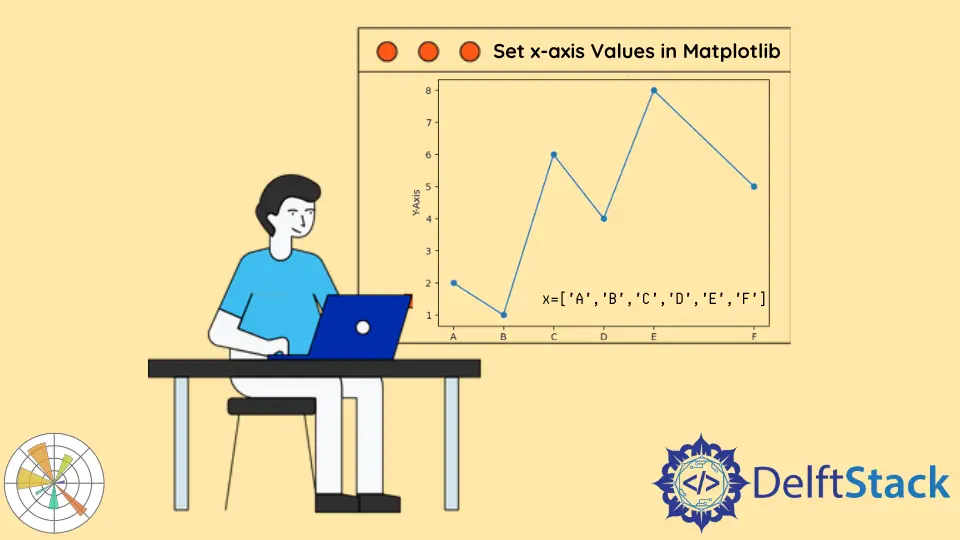
We can set the X-axis values using the matplotlib.pyplot.xticks()
method.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5, 7]
y = [2, 1, 6, 4, 8, 5]
plt.plot(x, y, marker="o")
plt.xlabel("X-Axis")
plt.ylabel("Y-Axis")
plt.title("Figure with default X labels")
plt.show()
Output:
It generates a figure with default labels for both the X-axis and Y-axis. By default, the X-axis and Y-axis ticks are assigned as equally spaced values ranging from minimum to maximum value of the respective axis. To change the default values of ticks for X-axis, we use the matplotlib.pyplot.xticks()
method.
Set X Axis Values Using matplotlib.pyplot.xticks()
Method
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5, 7]
y = [2, 1, 6, 4, 8, 5]
values = ["A", "B", "C", "D", "E", "F"]
plt.plot(x, y, marker="o")
plt.xlabel("X-Axis")
plt.ylabel("Y-Axis")
plt.title("Set X labels in Matplotlib Plot")
plt.xticks(x, values)
plt.show()
Output:
It sets the values of the X-axis ticks to the values in the list values
. Each element in the values
will serve as an X-axis tick for the corresponding element in the list x
.
The ticks will also be spaced as per the spacing of elements used for plotting.
import matplotlib.pyplot as plt
x = [0.01, 0.1, 1, 10, 100]
y = [2, 1, 6, 4, 8]
values = ["A", "B", "C", "D", "E", "F"]
plt.plot(x, y, marker="o")
plt.xlabel("X-Axis")
plt.ylabel("Y-Axis")
plt.title("Set X labels in Matplotlib Plot")
plt.xticks(x, values)
plt.show()
Output:
If the values plotted along the X-axis are not equally spaced, then the ticks along the X-axis will also be unequally spaced, and we might not get a clear figure, as shown in the output figure above.
We can plot the data using a list of equally spaced arbitrary values along the X-axis and then assign the actual values as ticks to the X-axis.
import matplotlib.pyplot as plt
x = [0.01, 0.1, 1, 10, 100]
y = [2, 1, 6, 4, 8]
values = range(len(x))
plt.plot(values, y, marker="o")
plt.xlabel("X-Axis")
plt.ylabel("Y-Axis")
plt.title("Set X labels in Matplotlib Plot")
plt.xticks(values, x)
plt.show()
Output:
It sets the equally placed X-axis ticks to the plot with unequally spaced X-axis values.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn