How to Set Tick Labels Font Size in Matplotlib
-
Using
plt.xticks(fontsize= )
to Set Matplotlib Tick Labels Font Size -
Using
ax.set_xticklabels(xlabels, fontsize= )
to Set Matplotlib Tick Labels Font Size -
Using
plt.setp(ax.get_xticklabels(), fontsize=)
to Set Matplotlib Tick Labels Font Size -
Using
ax.tick_params(axis='x', Labelsize= )
to Set Matplotlib Tick Labels Font Size - Conclusion
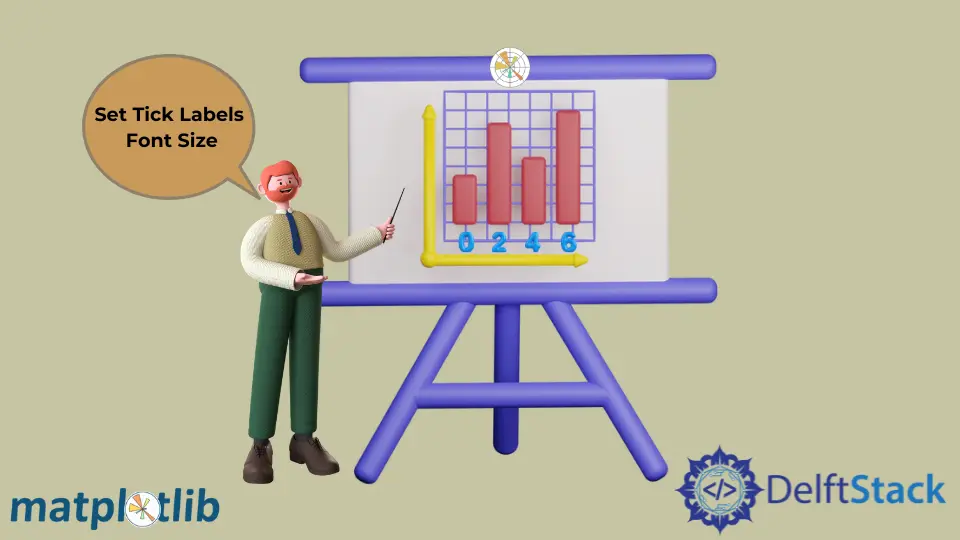
Matplotlib, a versatile plotting library in Python, empowers users with an array of methods to fine-tune the aesthetics of their plots. One such customization is the adjustment of tick label font size – a subtle yet impactful modification that significantly enhances the legibility and visual appeal of your visualizations.
We will use different methods available in Matplotlib for setting tick label font size. Each method offers a unique approach, allowing users to tailor their plots to meet specific needs and preferences.
Using plt.xticks(fontsize= )
to Set Matplotlib Tick Labels Font Size
The fontsize
parameter is applied as an argument within the xticks
and yticks
functions, specifying the desired font size in points. The points here refer to the typographic points commonly used in print, with one point equal to 1/72 of an inch.
Let’s start with a simple example to illustrate how to use the fontsize
parameter in the xticks
and yticks
methods to set the font size for both x-axis and y-axis tick labels:
Example:
import matplotlib.pyplot as plt
# Sample data for illustration
x_values = [1, 2, 3, 4, 5]
y_values = [2, 4, 6, 8, 10]
# Creating a basic plot
plt.plot(x_values, y_values)
# Setting font size for x-axis and y-axis tick labels
plt.xticks(fontsize=12)
plt.yticks(fontsize=6)
# Displaying the plot
plt.show()
Now, let’s break down the code step by step to understand how the fontsize
parameter in the xticks
and yticks
methods is utilized.
We start by importing the Matplotlib library, commonly aliased as plt
, which provides a convenient interface for creating and customizing plots.
Then, we define some sample data for our plot. In this case, x_values
represent the x-axis values, and y_values
represent the corresponding y-axis values.
We create a basic line plot using the plot
function, visualizing the relationship between x
and y
values.
Next is the key part of the code: the two lines that set the font size for the x-axis and y-axis tick labels.
The fontsize
parameter is used within the xticks
and yticks
methods to set the font size for the x-axis and y-axis tick labels, respectively. In this example, we’ve set the font size to 12
and 6
.
Finally, the plt.show()
is used to render and display the plot.
Output:
Using ax.set_xticklabels(xlabels, fontsize= )
to Set Matplotlib Tick Labels Font Size
In Matplotlib, the ax.set_xticklabels()
method is used to customize the appearance of x-axis tick labels on a specific subplot (axes). One of the parameters available for customization is fontsize
, which allows you to set the font size for the x-axis tick labels.
Let’s dive into a practical example that demonstrates how to leverage the set_xticklabels
and set_yticklabels
methods to adjust the font size of tick labels.
Example:
import matplotlib.pyplot as plt
# Sample data for illustration
x_values = [1, 2, 3, 4, 5]
y_values = [2, 4, 6, 8, 10]
# Creating a basic plot
fig, ax = plt.subplots()
ax.plot(x_values, y_values)
# Setting ticks first
ax.set_xticks(x_values)
ax.set_yticks(y_values)
# Setting font size for x-axis and y-axis tick labels
ax.set_xticklabels(ax.get_xticks(), fontsize=12)
ax.set_yticklabels(ax.get_yticks(), fontsize=10)
# Displaying the plot
plt.show()
Let’s break down the code step by step, unraveling the usage of set_xticklabels
and set_yticklabels
.
We start by importing the Matplotlib library using the common alias plt
.
Then, we define some sample data to be visualized in the plot. Here, x_values
represent the x-axis values, and y_values
represent the corresponding y-axis values.
We create a basic line plot using the plot
function within a figure and axis object (fig, ax
). This allows us to manipulate the properties of the plot more flexibly.
The crux of the matter – the set_xticklabels
and set_yticklabels
methods are employed here. We use ax.get_xticks()
and ax.get_yticks()
to retrieve the tick positions, and then we set their font size to 12
and 10
.
Lastly, the output of the code is shown using plt.show()
.
Output:
Using plt.setp(ax.get_xticklabels(), fontsize=)
to Set Matplotlib Tick Labels Font Size
In Matplotlib, the setp
function is a powerful utility that allows you to set properties for a collection of objects, such as tick labels, in a concise and efficient manner.
The ax.get_xticklabels()
method retrieves the x-axis tick labels associated with a specific subplot (axes). When combined with setp
, it becomes a versatile way to customize various properties of these tick labels, with fontsize
being one of the properties that can be easily adjusted.
Let’s dive into a practical example that demonstrates the application of the setp
method to adjust the font size of tick labels.
Example:
import matplotlib.pyplot as plt
# Sample data for illustration
x_values = [1, 2, 3, 4, 5]
y_values = [2, 4, 6, 8, 10]
# Creating a basic plot
fig, ax = plt.subplots()
ax.plot(x_values, y_values)
# Setting font size for both x-axis and y-axis tick labels using setp
plt.setp(ax.get_xticklabels(), fontsize=16)
plt.setp(ax.get_yticklabels(), fontsize=12)
# Displaying the plot
plt.show()
Let’s break down the code, exploring the use of the setp
method to dynamically set the font size for both x-axis and y-axis tick labels.
First, we import Matplotlib, the powerhouse for creating various types of plots in Python. We then define the sample data that will be used to create a basic line plot.
We create a simple line plot within a figure and axis object (fig, ax
). This object-oriented approach allows us to manipulate the plot properties more flexibly.
Next, the main part of the code snippet is the two lines that set the font size for both the x-axis and y-axis tick labels using the setp
method.
The setp
method is employed to set the font size of tick labels dynamically. We use ax.get_xticklabels()
and ax.get_yticklabels()
to retrieve the tick labels for the x-axis and y-axis, respectively, and then apply the fontsize
parameter.
Finally, the plt.show()
is used to display the plot.
Output:
Using ax.tick_params(axis='x', Labelsize= )
to Set Matplotlib Tick Labels Font Size
In Matplotlib, the tick_params
method is a versatile tool for configuring the appearance of ticks and tick labels on both the x-axis and y-axis of a subplot (axes). The axis
parameter within tick_params
allows you to specify whether the configurations apply to the x-axis (x
) or y-axis (y
). The labelsize
parameter, in particular, enables you to set the font size for tick labels on the specified axis.
Let’s explore a practical example that showcases how to leverage the tick_params
method to set the font size for tick labels:
Example:
import matplotlib.pyplot as plt
# Sample data for illustration
x_values = [1, 2, 3, 4, 5]
y_values = [2, 4, 6, 8, 10]
# Creating a basic plot
fig, ax = plt.subplots()
ax.plot(x_values, y_values)
# Setting font size for both x-axis and y-axis tick labels using tick_params
ax.tick_params(axis="both", labelsize=12)
# Displaying the plot
plt.show()
Let’s break down the code, highlighting the utilization of the tick_params
method to control the font size of tick labels.
We begin by importing Matplotlib, the go-to library for creating a wide range of plots in Python. We then define sample data to be visualized in a basic line plot.
Next, we create a simple line plot within a figure and axis object (fig, ax
). This object-oriented approach allows for more flexible customization.
The focal point of the code is the tick_params
method. By specifying axis='both'
and setting labelsize=12
, we dynamically adjust the font size of both the x-axis and y-axis tick labels.
Finally, we use plt.show()
to render and display the plot.
Output:
Conclusion
The article delves into diverse methods for adjusting tick label font size in Matplotlib, addressing the need for precise control over plot aesthetics. The covered methods include:
- Using
fontsize
inxticks
andyticks
: Directly sets the font size for both the x-axis and y-axis tick labels, offering a straightforward approach. set_xticklabels
andset_yticklabels
Methods: Explores the use of these methods to customize font size, providing more granular control over tick label appearance.setp
Method: Showcases the versatility of thesetp
method, enabling efficient customization of tick label properties, including font size.tick_params
Method: Illustrates the use oftick_params
to configure various tick-related parameters, with a focus on adjusting font size for x-axis and y-axis tick labels.
Each method is demonstrated through practical code examples, emphasizing its application in Matplotlib. These techniques collectively empower users, from beginners to seasoned data scientists, to tailor the font size of tick labels, contributing to clearer and visually compelling data visualizations.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Matplotlib Axes
- How to Rotate X-Axis Tick Label Text in Matplotlib
- How to Add a Y-Axis Label to the Secondary Y-Axis in Matplotlib
- How to Plot Logarithmic Axes in Matplotlib
- How to Set Limits for Axes in Matplotlib
- How to Reverse Axes in Matplotlib